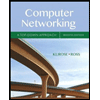
Create a class called Quadratic for performing arithmetic on and solving quadratic equations. A quadratic equation is an equation of the form
ax2 + bx + c = 0
Where a !=0. Use double variables to represent the values of a, b, and c and provide a constructor that enables objects of this class to be initialized when they are created. Give default values of a = 1, b = 0, and c = 0. Create a char variable called variable to represent the variable used in the equation and give it a default value of x. The constructor should not allow the value of a to be 0. If 0 is given, assign 1 to a). Provide public member functions that perform the following tasks.
- add—adds two Quadratic equations by adding the corresponding values of a, b, and c.
The function takes another object of type Quadratic as its parameter and adds it to the calling object.
- subtract—subtracts two Quadratic equations by subtracting corresponding values of a, b, and c. The function takes another object of type Quadratic as its parameter and subtracts it from the calling object.
- toString—returns a string representation of a quadratic equation in the form ax2 + bx + c = 0 using the actual values of the data members.
- solve—solves a quadratic equation using the quadratic formula x =
and displays the solutions if (b2 − 4ac) is greater than 0. Otherwise, it displays “No Real Roots ” your program should also contain constructor overloading.
Write a main function to test the functionality of the Quadratic class

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- 1. Create a class called Elevator that can be moved between floors in an N-storey building. Elevator uses a constructor to initialize the number of floors (N) in the building when the object is instantiated. Elevator also has a default constructor that creates a five- (5) storey building. The Elevator class has a termination condition that requires the elevator to be moved to the main (i.e., first) floor when the object is cleaned up. Write a finalize() method that satisfies this termination condition and verifies the condition by printing a message to the output, Elevator ending: elevator returned to the first floor. In main(), test at least five (5) possible scenarios that can occur when Elevator is used in a building with many floors (e.g., create, move from one floor to another, etc.). Hint: Termination occurs when the instance is set to null. You may wish to investigate “garbage collection” for this exercise. When solving this problem make sure that the user can interact with the…arrow_forwardCreate a class named Person.a. This class must have two variables to store name (string) and age (int). These variables should not be accessible from outside the class.b. Encapsulate the variables defined in item a with a property (an intermediate variable) so that they can be accessed from other classes. And after this step, use only intermediate variables that you defined for encapsulation within methods and other classes.c. Define a constructor method for this class. Code the constructor method to initialize the properties of the class at the time of object definition.D. Define a method called Print Info that will print the information of the class object on the screen in a proper format.arrow_forwardI need this assignment done in java with screenshots of it running.arrow_forward
- Construct a Time class containing integer data members seconds, minutes, and hours. Have the class contain two constructors: The first should be a default constructor having the prototype Time(int, int, int), which uses default values of 0 for each data member. The second constructor should accept a long integer representing a total number of seconds and disassemble the long integer into hours, minutes, and seconds. The final member method should display the class data members.arrow_forwardCreate a class called Pizza (Pizza.java). It should have the following private data members: String size: size of the pizza could be small, medium, or large int numofToppings: number of toppings could be 1, 2 or 3. double price and should provide the following methods: default constructor (set size to an empty string, numOfTopping and price to 0) non-default constructor Pizza(String s, int n, double p) getters and setters for the three data members method toString() returns a string representing the Pizza object, formatted as: Size: large Toppings: 2 Price: 9.95 method equals(Object obj) to indicate some other object is "equal to" this one public class Pizza { Complete the following unit test for the class Pizza described above. Make sure to test each public constructor and each public method, print the expected and actual results. // Start of the unit test of class Pizza public class PizzaTester { public static void main() { // Your source codes are placed herearrow_forwardPLEASE WRITE THE CODE WITH USER ERROR AND ALL ACCEPTABLE ENTRIES!!!arrow_forward
- A complex number has the form a+bi ,can be expressed as the ordered pair of real numbers (a,b).Theclassrepresents the real and imaginary parts as double precision values.Provide a constructor that enables an object of this class to be initialized when it is instantiated. The constructorshould contain default values.Provide Public member functions for each of the following arithmetic’sfunctions (addition –subtraction –multiplication –division), a complex absolute value operation, printing the number in the form (a,b), printing the real part , printing the imaginary part and final overload the == operator to allow comparisons of two complex numbers.Include any additional operations that you think would be useful for a complex number class.Design, implement, and test your class.arrow_forwardIt not complex please don't reject it. give code of it. Wrong answer downvote surearrow_forwardPart 1: Setting the turtle size when it is instantiated Currently, a turtle is created and given a default size of 50. To change the size of the turtle its increaseTurtleSizeBy10 and decreaseTurtleSizeBy10 methods can be called. Add functionality so that a turtle can be created with a specified size. In the DoodleTurtle class do the following: Overload the DoodleTurtle constructor by creating a one-parameter constructor that takes the size of the turtle as an integer. Add an empty method body. Complete the method specification for the new constructor. The default constructor method specification can be used as a model. The only difference will be that the getTurtleSize() will equal the name of the size parameter instead of INITIAL_SIZE. In the method body of the one-parameter constructor do the following in this order: Call the initializeWithDefaultValues helper method. Call the setSize method passing it the size parameter. Compile the program and fix any…arrow_forward
- 1. Create a properly encapsulated class named Person that has the following: A String instance variable called name. A constructor that takes a String parameter and sets the instance variable. A properly named getter for the instance variable. A correctly overrided equals method which returns true if the name instance variable of the parameter and the reference variable calling the method are equal. (Do not forget the null and class type checks!) Compile your Person class. 2. Create a properly encapsulated class named Employee that inherits from Person and has the following: ● ● ● A String instance variable called office (e.g. "LWH 2222"). A String instance variable called hourlywage (e.g. "$45.15"). A constructor that takes three Strings as parameters for name, office, and hourlyWage and sets the instance variables of both the parent class and the Employee class. An overridden toString method that returns the concatenation of name and hourlyWage instance variables (separated by a…arrow_forwardLittle debugging help The program runs correctly but doesn't follow instructions completely. Currently, I have a parameterized constructor in the circle class when it asked for default I need help to fix the circle subclass. Please Write a default constructor that initializes the name of the Circle object to "Circle". You will need to call the constructor of the parent Shape class. The program runs everything in ShapeDriver /** * Write a Circle class that extends the Shape class. Do the following:* 1. Write the class header.* 2. Write a default constructor that initializes the name of the Circle object to "Circle".* You will need to call the constructor of the parent Shape class.* 3. Write a void method printMessage() that prints the message " I have no sides".**///Your code goes herepublic class Circle extends Shape {public Circle(String myName) {super(myName);}public void printMessage() {System.out.println("I have no sides");}} /*** * Write a Rectangle class that extends the…arrow_forwardCreate a class called Rational for performing arithmetic with fractions. Write a program to test your class. Use integer variables to represent the private data of the class—the numerator and the denominator. Provide a constructor that enables an object of this class to be initialized when it’s declared. The constructor should contain default values in case no initializers are provided and should store the fraction in reduced form. For example, the fraction 12/27 would be stored in the object as 4 in the numerator and 9 in the denominator. Provide public member functions that perform each of the following tasks: a) Adding two Rational numbers. The result should be stored in reduced form. b) Subtracting two Rational numbers. The result should be stored in reduced form. c) Multiplying two Rational numbers. The result should be stored in reduced form. d) Dividing two Rational numbers. The result should be stored in reduced form. e) Printing Rational numbers in the form…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
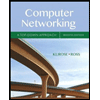
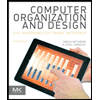
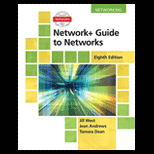
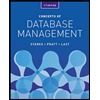
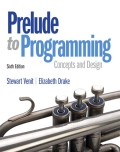
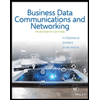