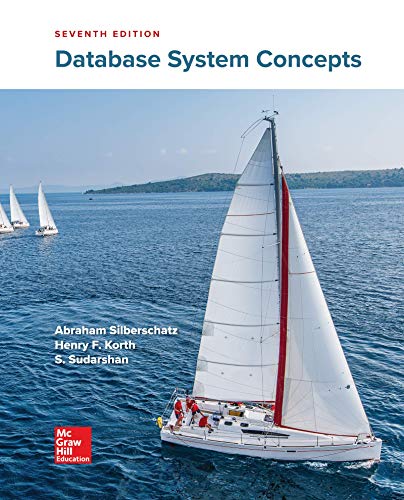
Concept explainers
Create a class called Rational for performing arithmetic with fractions. Write a
a) Adding two Rational numbers. The result should be stored in reduced form.
b) Subtracting two Rational numbers. The result should be stored in reduced form.
c) Multiplying two Rational numbers. The result should be stored in reduced form.
d) Dividing two Rational numbers. The result should be stored in reduced form.
e) Printing Rational numbers in the form a/b, where a is the numerator and b is the denominator.
f) Printing Rational numbers in floating-point format.
Design (UML/PC):
Code:
Output:

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- 1. Create a properly encapsulated class named Person that has the following: A String instance variable called name. A constructor that takes a String parameter and sets the instance variable. A properly named getter for the instance variable. A correctly overrided equals method which returns true if the name instance variable of the parameter and the reference variable calling the method are equal. (Do not forget the null and class type checks!) Compile your Person class. 2. Create a properly encapsulated class named Employee that inherits from Person and has the following: ● ● ● A String instance variable called office (e.g. "LWH 2222"). A String instance variable called hourlywage (e.g. "$45.15"). A constructor that takes three Strings as parameters for name, office, and hourlyWage and sets the instance variables of both the parent class and the Employee class. An overridden toString method that returns the concatenation of name and hourlyWage instance variables (separated by a…arrow_forwardA. Build a class named car. This class is defined as follows: It has the fields: Car ID, Car model, Car make, Car color, Car year. Build a constructor that accepts the five parameters (Car ID, Car model, Car make, Car color, Car year). Override the method toString() to return the string representation of Car ID, Car model, Car make, Car color, Car year. Build a method named display to print the values returned by toString() method. Override the equals() method to compare the Car model, Car make, Car color of two Car objects. The method returns true if all the values of these fields are the same, and false otherwise. In the equals method you must check the following: a. If you compare the Car object to itself, return true.! b. If you compare car object with another car object return true, otherwise return false. Hint use : instanceof operator for this purpose. Override the hashcode() method to return same hash codes for objects that are equal according to the equals() method you built…arrow_forward!! E! 4 2 You are in process of writing a class definition for the class Book. It has three data attributes: book title, book author, and book publisher. The data attributes should be private. In Python, write an initializer method that will be part of your class definition. The attributes will be initialized with parameters that are passed to the method from the main program. Note: You do not need to write the entire class definition, only the initializer method lili lilıarrow_forward
- Which of the following statements is false? a. A class can contain only one constructor. b. An example of a behavior is the SetTime method in a Time class. c. An object created from a class is referred to as an instance of the class. d. An instance of a class is considered an object.arrow_forwardWrite a class Runner having three private data members (distance, minutes, seconds) The class has A constructor which having no parameter – for setting values to zero or null.Getters/setters for data members.Write a function show() which displays the“The player covered “+distance+ “ in “ +minutes +” minutes , and “ + seconds+” seconds”; Write test Application that demonstrates the above class by calling all the methods, creating a Create 3 Runner objects, input values and then display the record of winner.arrow_forwardCreate a class called time. The time class has three private data members of type int, named hours, minutes and seconds. • Provide a no‐argument constructor for the class which initializes the data members to 0. • Provide another constructor which sets the data members to the values passed from the main. It should also validate whether the values are correct i.e., hours should be between 0 and 23 (inclusive), minutes should be between 0 and 60 (inclusive) and seconds should also be between 0 and 60 (inclusive). If an invalid value is entered, the constructor should automatically change it to 0. • Write a function getTime() which asks the user to input the values for hours, minutes and seconds. While taking the input values, it must also validate the input values as in the above case. • Write a function displayTime() which displays the time in the format 11:39:45 i.e. hours:minutes:seconds. • Write a function totaltime () that adds two objects of type Time passed as…arrow_forward
- Using c++ programming language. Write a class Distance that holds distances or measurements expressed in feets and inches. Thisclass has two private data members: feet: An integer that holds the feet. inches: An integer that holds the inches. Write a constructor with default parameters that initializes each data member of the class.If inches are greater than equal to 12 then they must be appropriately converted tocorresponding feet. Generate appropriate getter-setter functions for the data members.o void setFeet(int f) and int getFeet()consto void setInches(int i) It should ensure proper conversion to feet.o int getInches() const Define an operator ‘+’ that overloads the standard ‘+’ math operator and allows oneDistance object to be added to another. Distance operator+ (const Distance &obj). Define an operator - function that overloads the standard ‘-‘ math operator and allowssubtracting one Distance object from another. Distance operator-(const Distance &obj)…arrow_forwardWrite a class definition line and a one line docstring for the class Dog. Write an __init__ method for the class Dog that gives each dog its own name and breed. Test this on a successful creation of a Dog object.>>> import dog>>> sugar = dog.Dog('Sugar', 'border collie')>>> sugar.name'Sugar'>>> sugar.breed'border collie' Add a data attribute tricks of type list to each Dog instance and initialize it in __init__ to the empty list. The user does not have to supply a list of tricks when constructing a Dog instance. Make sure that you test this successfully.>>> sugar.tricks[] Write a method teach as part of the class Dog. The method teach should add a passed string parameter to tricks and print a message that the dog knows the trick.>>> sugar.teach('frisbee')Sugar knows frisbeearrow_forwardInside the FlightController class, you will define an object of type FlightSchedule which willcontain an array of 3 objects of type Flight. In your Flight objects, the constructor of theseobjects will ask from the user for the inputs of: flight name, launch hour, launch minutes,flight duration. The value of state of the Flight object will be assigned to “Idle” as a defaultvalue in the constructor also and you will call the member function within the Flight classwhich will calculate the landing time for your flight object by using the duration and thelaunch time. Definition of Flight class will also contain the necessary getters and setters thatare given in the diagram and needed throughout your program.In the constructor of your FlightController you will need to define a pointer that will point tothe adress of the FlightSchedule object you have created. You will send that pointer into thefunctions launchSchedule and landingSchedule in your base classes and these functions willperform a…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
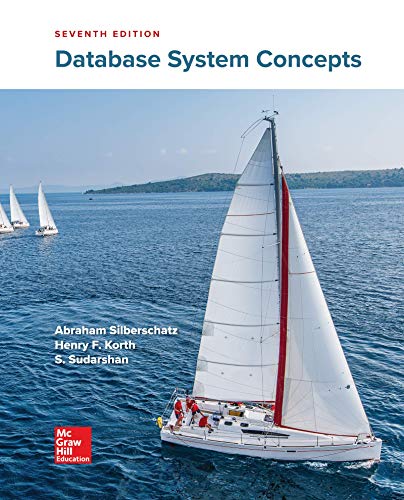
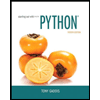
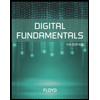
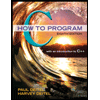
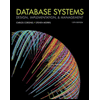
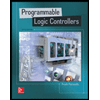