Create a binary linked tree, and traverse the tree by using the recursive function. The structure of the tree is as follow: You should input the nodes in pre-order sequence. If a child of a node is NULL, input a space. Write the function of create binary tree, pre-order to print the nodes, in-order to print the nodes and post-order to print the nodes. Count the height of the tree. Header file typedef char ElemType; typedef struct node//define the type of binary tree node { }BTnode; Source file #include #include #include "tree.h" BTnode * createTree()//create the binary tree,return the root { BTnode *tnode;// tnode is the root char elem; ;//input the character //if the input is a space,set the pointer as NULL Else// if the input is not a space,generate the binary node and create its left sub-tree and right sub-tree { ; ; ; ; } return tnode; } void preOrder(BTnode *root)//preorder traverse the tree and print the node sequence { } void inOrder(BTnode *root)// inorder traverse the tree and print the node sequence { } void postOrder(BTnode *root)// postorder traverse the tree and print the node sequence { } } int Height(BTnode *root) { } void main()//call the function to create binary tree and print the traverse sequence. Print the height of the tree. { }
- Create a binary linked tree, and traverse the tree by using the recursive function.
The structure of the tree is as follow:
You should input the nodes in pre-order sequence. If a child of a node is NULL, input a space.
Write the function of create binary tree, pre-order to print the nodes, in-order to print the nodes and post-order to print the nodes.
Count the height of the tree.
Header file
typedef char ElemType;
typedef struct node//define the type of binary tree node
{
}BTnode;
Source file
#include <stdio.h>
#include <stdlib.h>
#include "tree.h"
BTnode * createTree()//create the binary tree,return the root
{
BTnode *tnode;// tnode is the root
char elem;
;//input the character
//if the input is a space,set the pointer as NULL
Else// if the input is not a space,generate the binary node and create its left sub-tree and right sub-tree
{
;
;
;
;
}
return tnode;
}
void preOrder(BTnode *root)//preorder traverse the tree and print the node sequence
{
}
void inOrder(BTnode *root)// inorder traverse the tree and print the node sequence
{
}
void postOrder(BTnode *root)// postorder traverse the tree and print the node sequence
{
}
}
int Height(BTnode *root)
{
}
void main()//call the function to create binary tree and print the traverse sequence. Print the height of the tree.
{
}

Step by step
Solved in 2 steps

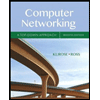
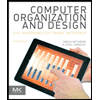
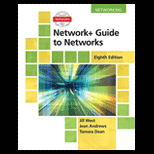
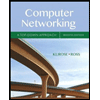
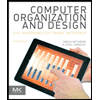
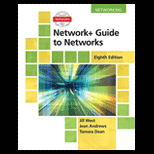
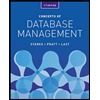
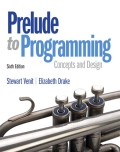
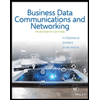