Counting Operations to Produce Polynomials In the following section, I will present you with multiple different bodies of code, and it is your job to analyze each code section and build a polynomial that represents the number of abstract operations the code performs. Once we’re done here, we’ll have built a polynomial that we will analyze further (in terms of g(x), c, 5 and k) in the next section. For the following code segments below, count the operations and produce a corresponding polynomial representation. f(x)= public static boolean isEmpty() { return head == null; } f(x) public static int num_occurrences(int n) { int count = 0;
Counting Operations to Produce Polynomials
In the following section, I will present you with multiple different bodies of code, and it is your job to analyze each code section and build a polynomial that represents the number of abstract operations the code performs. Once we’re done here, we’ll have built a polynomial that we will analyze further (in terms of g(x), c, 5 and k) in the next section. For the following code segments below, count the operations and produce a corresponding polynomial representation.
f(x)=
public static boolean isEmpty() {
return head == null;
}
f(x)
public static int num_occurrences(int n) {
int count = 0;
for(int i = 0; i < n; i++) {
for(int j = 0; j < n; j++) {
if( i == j ) continue;
if(strings[i] == strings[j]) {
count++;
}
}
}
return count;
}
f(x)
public static void c(int n) {
for(int a = 0; a < n; a++) {
System.out.println( a * a);
}
num_occurrences(n);
}
f(x)
public static boolean isPrime(int n) {
if(n == 1) return false;
for(int i = 2; i <n; i++) {
if( n % i == 0 ) {
return false;
}
}
return true;
}
Demonstrating | f(x) | < c | g(x) | for all n > k
For each of the polynomials above, pick a reference function (g(x)) and constants c,k such that the g(x) bounds f(x) in the most succinct terms possible for Big O.
1. For the function isEmpty() above, what is ...
f(x)=
g(x)=
c=
k=
2. For the function num_occurences() above, what is...
f(x)
g(x)=
c=
k=
3. For the function c() above, what is...
f(x)=
g(x)=
c=
k=
4. For the function isPrime() above, what is...
f(x)=
g(x)=
c=
k=
Future Exam Practice (optional)
We have more lecture and homework to go before we completely understand this next set of questions, but keep them in mind for future exams.
5. For your Bubble Sort, what is...
f(x)=
g(x)=
c=
k=
6. For your Selection Sort, what is...
f(x)=
g(x)=
c=
k=



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

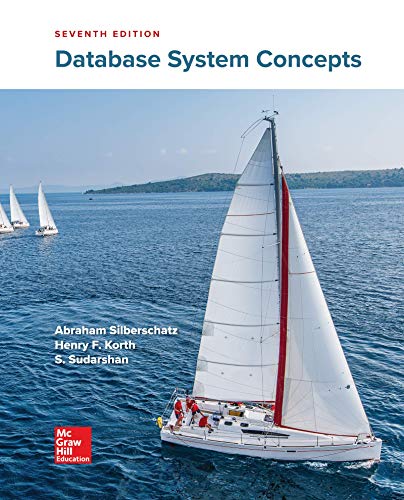
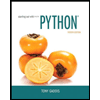
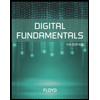
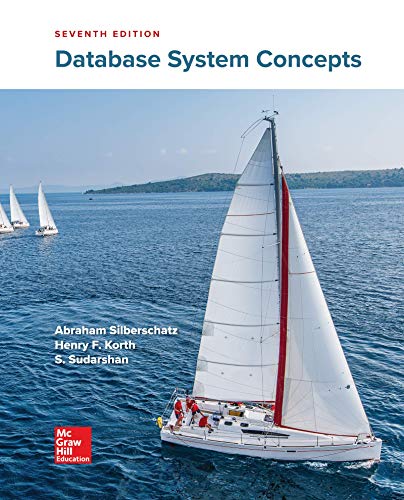
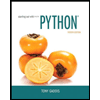
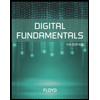
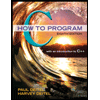
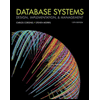
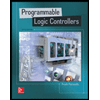