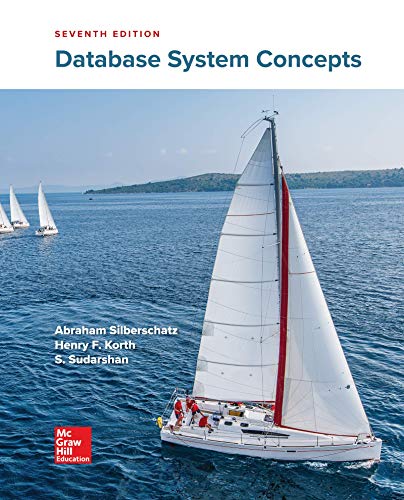
Concept explainers
Can you please help me with this code because I am really struggling and I don't know where to start because I find it confusing. Can you please help me out. I added the text.
question:
you will investigate the movement of disks on a linear grid. The starting configuration of this puzzle is a row of L cells, with disks located on cells 0 through n - 1. The goal is to move the disks to the end of the row using a constrained set of actions. At each step, a disk can only be moved to an adjacent empty cell, or to an empty cell two spaces away, provided another disk is located on the intervening cell. Given these restrictions, it can be seen that in many cases, no movements will be possible for the majority of the disks. For example, from the starting position, the only two options are to move the last disk from cell n - 1 to cell n, or to move the second to-last disk from cell n - 2 to cell n.
Task:
Write a function solve_identical_disks(length, n) that returns an optimal solution to the above problem as a list of moves, where length is the number of cells in the row and n is the number of disks. Each move in the solution should be a two-element tuple of the form (from, to) indicating a disk movement from the first cell to the second. As suggested by its name, this function should treat all disks as being identical. Your solver for this problem should be implemented using a breadth-first graph search. The exact solution produced is not important, as long as it is of minimal length. Unlike in the previous two sections, no requirement is made with regards to the manner in which puzzle configurations are represented. Before you begin, think carefully about which data structures might be best suited for the problem, as this choice may affect the efficiency of your search.
>>> solve_identical_disks(4, 2)
[(0, 2), (1, 3)]
>>> solve_identical_disks(5, 2)
[(0, 2), (1, 3), (2, 4)]
>>> solve_identical_disks(4, 3)
[(1, 3), (0, 1)]
>>> solve_identical_disks(5, 3)
[(1, 3), (0, 1), (2, 4), (1, 2)]
Write a function solve_distinct_disks(length, n) that returns an optimal solution to the same problem with a small modification: in addition to moving the disks to the end of the row, their final order must be the reverse of their initial order. More concretely, if we abbreviate length as L, then a desired solution moves the first disk from cell 0 to cell L - 1, the second disk from cell 1 to cell L - 2, . . . , and the last disk from cell n - 1 to cell L - n. Your solver for this problem should again be implemented using a breadth-first graph search. As before, the exact solution produced is not important, as long as it is of minimal length.
>>> solve_distinct_disks(4, 2)
[(0, 2), (2, 3), (1, 2)]
>>> solve_distinct_disks(5, 2)
[(0, 2), (1, 3), (2, 4)]
>>> solve_distinct_disks(4, 3)
[(1, 3), (0, 1), (2, 0), (3, 2), (1, 3), (0, 1)]
>>> solve_distinct_disks(5, 3)
[(1, 3), (2, 1), (0, 2), (2, 4), (1, 2)]
code that needs to be added:
def solve_identical_disks(length, n):
pass
def solve_distinct_disks(length, n):
pass

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Part C: Function, for and plotting We did a project in the lecture on calculating the free fall speeds and plotting them on a graph. This part is similar to the project. An engineer has derived a relationship between the force applied to a material and the extension in length that the force would cause. The relationship between force f and extension e is given by: You are asked to plot a graph showing the relationship between force and extension. You are asked to complete the following tasks: Task 1 Write a Python function which returns the value of e for a given input f. Do not use literals (e.g. 5.5, 10) in the expressions for e in the function. Instead you should define constants and use them. Note that the relationship between e and f depends on whether f is bigger than 10 or not, this means you need a certain Python construction in your function. If you can't think of that, have a look at Part A of Lab03.arrow_forwardBuild a polyhedron that looks roughly like the Washington monument. Some key dimensions of the Washington Monument: o The width of the base is about 55 feet.o The width of each side at the top is about 34 feet.o The height of the small pyramid (pyramidion) at the top of the monument is 55 feet. o The height of the whole monument is 555 feet. This should help you get something that looks approximately like the Washington monument. Exactness is not necessary, and indeed, you should make your code appropriately parameterized.Some additional requirements. Your polyhedron will have 8 sides (four for the pyramidion and four for the tower). You should not have a base. The origin of the monument should be at the center of the base, directly below the peak. Each side should be a different color, so that all the edges are plainly visible. o Use a variety of different ways of specifying the colors (e.g. THREE.ColorKeywords, hexidecimal notation, CSS string, RGB) o Use RGB color at least…arrow_forwardYou are given a 2 by n grid, where the cell on row i column j contains a non-negative number ai,j . You can start at either cell in the lefttmost column, and your goal is to reach either cell in the rightmost column by a sequence of moves. You can move to an adjacent cell (if it exists) in each of the 4 cardinal directions (up,down, left and right). A path achieves a score equal to the sum of values in its cells. Note that a cell which is used twice in a path only counts its value once to the score of that path.Design an algorithm which runs in O(n) time and finds a path of minimum score from the leftmost column to the rightmost column.arrow_forward
- Determine the value of the decision parameter for the circle drawing technique described by Bresenham on page. Bresenham's method for drawing circles is shown here in a stage-by-stage format.arrow_forwardCan you help me with this code because this is a little difficult for me:question that i need help with:The Lights Out puzzle consists of an m x n grid of lights, each of which has two states: on and off. The goal of the puzzle is to turn all the lights off, with the caveat that whenever a light is toggled, its neighbors above, below, to the left, and to the right will be toggled as well. If a light along the edge of the board is toggled, then fewer than four other lights will be affected, as the missing neighbors will beignored. In this section, you will investigate the behavior of Lights Out puzzles of various sizes by implementing a LightsOutPuzzle class task: A natural representation for this puzzle is a two-dimensional list of Boolean values, where True corresponds to the on state and False corresponds to the off state. In the LightsOutPuzzle class, write an initialization method __init__(self, board) that stores an input board of this form for future use. Also write a method…arrow_forwardBuild a polyhedron that looks roughly like the Washington monument. Some key dimensions of the Washington Monument: o The width of the base is about 55 feet.o The width of each side at the top is about 34 feet.o The height of the small pyramid (pyramidion) at the top of the monument is 55 feet. o The height of the whole monument is 555 feet. This should help you get something that looks approximately like the Washington monument. Exactness is not necessary, and indeed, you should make your code appropriately parameterized.Some additional requirements. Your polyhedron will have 8 sides (four for the pyramidion and four for the tower). You should not have a base. The origin of the monument should be at the center of the base, directly below the peak. Each side should be a different color, so that all the edges are plainly visible. o Use a variety of different ways of specifying the colors (e.g. THREE.ColorKeywords, hexidecimal notation, CSS string, RGB) o Use RGB color at least…arrow_forward
- I have modified the code above. The input to test the method floydWarshal(graph,v) will come from the user (from the console through the scanner). v can not be a final variable because the user will determine the value of v. The double array graph will also be determined and input by the user (from the console through the scanner). I'm having issues getting the input from the user and putting it into the double array. I'm also having issues printing the result of the method, which should be the shortest path graph. Please help ------------------------------------------------------------------------------------ HERE IS THE CODE THAT I HAVE SO FAR import java.io.*;import java.util.*;import java.lang.*; public class Solution { final static int INF = 99999; public static void shortWarshall(int graph[][],int v) { int dist[][] = new int[v][v]; int i, j, k; /* Initialize the solution matrix same as input graph matrix. Or we can say the initial values of shortest distances are…arrow_forwardDetermine the value of the decision parameter for the circle drawing technique described by Bresenham on page. Bresenham's method for drawing circles is shown here in a stage-by-stage format.arrow_forwardWrite a Java Program to print a 2D matrix in the Spiral format. You can assume the 2D matrix is your own.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
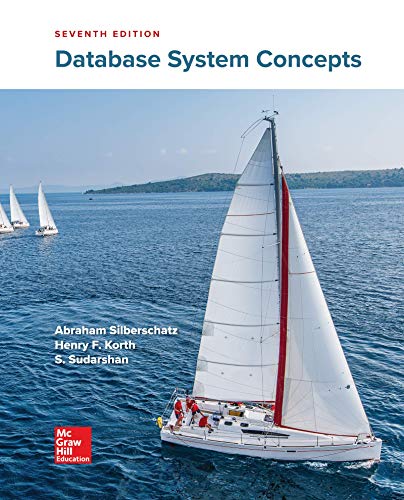
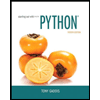
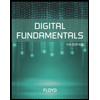
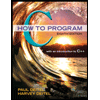
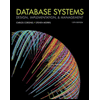
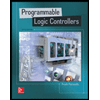