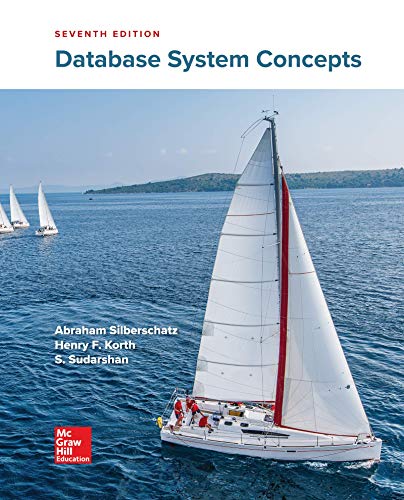
Complete the PoundDog code by adding a constructor having a constructor initializer list that initializes age with 1, id with -1, and name with "NoName". Notice that MyString's default constructor does not get called.
Note: If you instead create a traditional default constructor as below, MyString's default constructor will be called, which prints output and thus causes this activity's test to fail. Try it!
#include <iostream>
#include <string>
using namespace std;
class MyString {
public:
MyString();
MyString(string s);
string GetString() const { return str; };
void SetString(string s) { str = s; };
private:
string str;
};
MyString::MyString() {
cout << "MyString default constructor called" << endl;
str = "";
}
MyString::MyString(string s): str(s) {
}
class PoundDog {
public:
PoundDog();
void Print() const;
private:
int age;
int id;
MyString name;
};
/* Your solution goes here */
void PoundDog::Print() const {
cout << "age: " << age << endl;
cout << "id: " << id << endl;
cout << "name: " << name.GetString() << endl;
}
int main() {
PoundDog currDog;
currDog.Print();
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- omplete the PoundDog code by adding a constructor having a constructor initializer list that initializes age with 1, id with -1, and name with "NoName". Notice that MyString's default constructor does not get called. Note: If you instead create a traditional default constructor as below, MyString's default constructor will be called, which prints output and thus causes this activity's test to fail. Try it! // A wrong solution to this activity... PoundDog::PoundDog() { age = 1; id = -1; name.SetString("NoName"); } #include <iostream>#include <string>using namespace std; class MyString { public: MyString(); MyString(string s); string GetString() const { return str; }; void SetString(string s) { str = s; }; private: string str;}; MyString::MyString() { cout << "MyString default constructor called" << endl; str = "";} MyString::MyString(string s): str(s) {} class PoundDog { public: PoundDog(); void Print() const;…arrow_forwardComplete the PoundDog code by adding a constructor having a constructor initializer list that initializes age with 1, id with -1, and name with "NoName". Notice that MyString's default constructor does not get called. Note: If you instead create a traditional default constructor as below, MyString's default constructor will be called, which prints output and thus causes this activity's test to fail. Try it! // A wrong solution to this activity... PoundDog::PoundDog() { age = 1; id = -1; name.SetString("NoName"); } Here is the code: #include <iostream>#include <string>using namespace std; class MyString {public:MyString();MyString(string s);string GetString() const { return str; };void SetString(string s) { str = s; };private:string str;}; MyString::MyString() {cout << "MyString default constructor called" << endl;str = "";} MyString::MyString(string s): str(s) {} class PoundDog {public:PoundDog();void Print() const; private:int age;int id;MyString…arrow_forwardComplete the PoundDog code by adding a constructor having a constructor initializer list that initializes age with 1, id with -1, and name with "NoName". Notice that MyString's default constructor does not get called. Note: If you instead create a traditional default constructor as below, MyString's default constructor will be called, which prints output and thus causes this activity's test to fail. Try it! // A wrong solution to this activity... PoundDog::PoundDog() { age = 1; id = -1; name.SetString("NoName"); } In C++arrow_forward
- To overload constructors, we write multiple constructor declarations with the same signatures. True Falsearrow_forwardModify the student class presented in this chapter as follows. Each student object should also contain the scores for three tests. Provide a constructor that sets all instance values based on parameter values. Overload the constructor such that each test score is assumed to be initially zero. Provide a method called setTestScore that accepts two parameters: the test number (1 through 3) and the score. Also provide a method called getTestScore that accepts the test number and returns the appropriate score Provide a method called average that computes and returns the average test score for this student. Modify the toString method such that the test scores and average are included in the description of the student. Modify the driver class main method to exercise the new Student methods. (Java)arrow_forwardModify the student class presented in this chapter as follows. Each student object should also contain the scores for three tests. Provide a constructor that sets all instance values based on parameter values. Overload the constructor such that each test score is assumed to be initially zero. Provide a method called setTestScore that accepts two parameters: the test number (1 through 3) and the score. Also provide a method called getTestScore that accepts the test number and returns the appropriate score Provide a method called average that computes and returns the average test score for this student. Modify the toString method such that the test scores and average are included in the description of the student. Modify the driver class main method to exercise the new Student methods. (c++ language)arrow_forward
- Modify the Car class example and add the following: A default constructor that initializes strings to “N/A” and numbers to zero. An overloaded constructor that takes in initial values to set your fields (6 parameters). accel() method: a void method that takes a double variable as a parameter and increases the speed of the car by that amount. brake() method: a void method that sets the speed to zero. To test your class, you will need to instantiate at least two different Car objects in the main method in the CarDemo class. Each of your objects should use a different constructor. For the object that uses the default constructor, you will need to invoke the mutator methods to assign values to your fields. Invoke the displayFeatures() method for both objects and display the variables. Demonstrate the use of the accessor method getColor() by accessing the color of each car and printing it to the console. Use the method accel to increase the speed of the first car by 65 mph. Use…arrow_forwardUse this exercise to test your code that you wrote in the previous exercise. Problem Class In this exercise, you are going to create the Problem class. The Problem class is used to help simulate a math fact, for example: 2 + 5 = Your class needs to contain two constructors, one that takes String, int, int that represents the operator sign(+, -, *, or /), the minimum, and maximum values for the number range, and a second constructor that takes only a String that represents the operator sign. For the second constructor, the minimum should default to zero and the maximum to ten. Your Problem object should generate 2 random integers between the minimum and maximum values (inclusively). Each Problem object should only have one set of numbers that do not change. While you may include additional helper methods, two methods need to be available to the user. The first is the answer method that should return a double that represents the answer to the problem. The second is the toString that…arrow_forwardhttps://youtu.be/4hAoK54Pfdc Here is a video regarding the kit component.arrow_forward
- Help, I tried to write what I could but I am not sure what to write next. The code need to include Poylmorphism of passenger and elevator. Any help would be appreciated. There are 4 types of passengers in the system:Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements.VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators.Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators.Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. There are 4 types of elevators in the system:StandardElevator: This is the most common type of elevator and has a request…arrow_forwardJAVA ENCAPSULATIONarrow_forwardAdd comments as appropriate. Be sure that your program output is neatly presented to the user. Add documentation comments to your functions. You are going to change the class you created in Program7 so that it has a constructor and some properties. In your GeoPoint class make the following changes (note your variable, parameter and method names may be different. Adjust as needed.): Add a constructor __init__(self, lat=0, lon=0,description = ‘TBD’) that will initialize the class variables __lat ,__lon and the __description. Notice that the constructor will also default lat and lon to zero and description to ‘TBD’ if they are not provided. Change the SetPoint method so that instead of individual coordinates SetPoint(self, lat, lon) it takes a single sequence. Add a property: Point = property(GetPoint,SetPoint). Make sure GetPoint and SetPoint are the names you used for the get and set methods you already wrote for points. Add another property: Description = property(GetDescription,…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
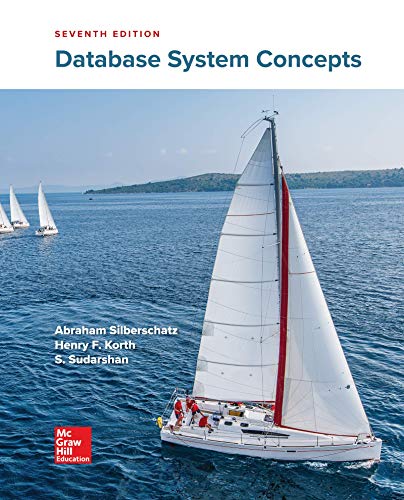
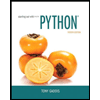
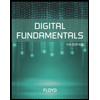
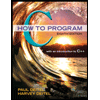
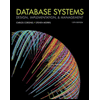
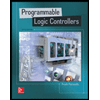