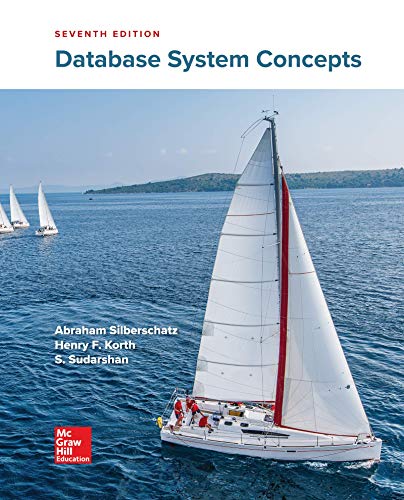
Concept explainers
Complete the given Car class with set, get,and display functions as well as a constructor that accepts all the attributes for all five data members and demonstrate that you can create a second object of the class Car.
Attributes: Object: Member Functions:
string make VW Turn on, accelerate, brake, turn off
string model PASSAT
string color Red
integer year 2020
integer mileage 0 Miles
Here is the code that is given
#include <iostream>
#include<string>
using namespace std;
class Car
{
public:
Car(string carMake, string carModel)
{
make = carMake;
model = carModel;
}
void setMake(string carMake)
{
make = carMake;
}
string getMake()
{
return make;
}
void setModel(string carModel)
{
model = carModel;
}
string getModel()
{
return model;
}
void displayCar()
{
cout << "Make: " << make << endl;
cout << "Model:" << model << endl;
}
private:
string make;
string model;
string color;
int year;
int mileage;
};
int main()
{
Car myCar("VW", "Passat");
// myCar.setMake("VW");
// myCar.setModel("Passat");
// cout << "Make: " << myCar.getMake() << endl;
// cout << "Model: " << myCar.getModel() << endl;
myCar.displayCar();
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- An Inventory structure is declared as follows: struct Inventory{int itemCode; int qtyOnHand;}; Write a definition statement that creates an Inventory variable named trivet and initializes it with an initialization list so that its code is 555 and its quantity is110.arrow_forward// CLASS PROVIDED: sequence (part of the namespace CS3358_FA2022)//// TYPEDEFS and MEMBER CONSTANTS for the sequence class:// typedef ____ value_type// sequence::value_type is the data type of the items in the sequence.// It may be any of the C++ built-in types (int, char, etc.), or a// class with a default constructor, an assignment operator, and a// copy constructor.//// typedef ____ size_type// sequence::size_type is the data type of any variable that keeps// track of how many items are in a sequence.//// static const size_type DEFAULT_CAPACITY = _____// sequence::DEFAULT_CAPACITY is the default initial capacity of a// sequence that is created by the default constructor.//// CONSTRUCTOR for the sequence class:// sequence(size_type initial_capacity = DEFAULT_CAPACITY)// Pre: initial_capacity > 0// Post: The sequence has been initialized as an empty sequence.// The insert/attach functions will work efficiently (without// allocating…arrow_forwardfunctions return the value of a data member. A. Accessor OB. Member C. Mutator OD. Utilityarrow_forward
- INVENTORY CLASS You need to create an Inventory class containing the private data fields, as well as the methods for the Inventory class (object). Be sure your Inventory class defines the private data fields, at least one constructor, accessor and mutator methods, method overloading (to handle the data coming into the Inventory class as either a String and/or int/float), as well as all of the methods (methods to calculate) to manipulate the Inventory class (object). The data fields in the Inventory class include the inventory product number (int), inventory product description (String), inventory product price (float), inventory quantity on hand (int), inventory quantity on order (int), and inventory quantity sold (int). The Inventory class (java file) should also contain all of the static data fields, as well as static methods to handle the logic in counting all of the objects processed (counter), as well as totals (accumulators) for the total product inventory and the total product…arrow_forwardclass Book: book_belongs_to = 'Schulich School of Engineering' def _init_(self, pages = 0, title = 'Unknown', author = 'Unknown', isbn = self.pages = pages self.title = title e): self.author = author self.isbn = isbn book1 = Book(255, 'Black Beauty', 'Anna Sewell', 9780001840423) book2 = Book(208, 'The Chrysalids', 'John Wyndham', 9780140013085) Book.book_belongs_to = 'Emily Marasco' book3 = Book()arrow_forwardChallenge 3: Vehicle.java, Automobile.java, Tank.java, Truck.java, Car.java, and TestVehicle.java Implement the classes that are given in the class diagram. Also implement a TestVehicle class where you will create three objects and execute their toString() methods. >> Automobile assignment2 a licensePlate: String make: String Automobile() Automobile(int,double, String, String) getLicensePlate(): String setLicense Plate(String):void ●getMake(): String setMake(String):void .toString(): String >> Ⓒ Truck assignment2 a towCapacity: double Truck() Truck(int, double, String, String, double) getTowCapacity():double setTowCapacity (double):void toString(): String Sample output > Vehicle assignment2 □ year: int weight: double Vehicle() Vehicle(int, double) getYear(): int setYear(int):void getWeight():double setWeight(double):void .toString(): String > Car assignment2 a maxPassengers: int Car() Car(int,double, String, String,int) getMaxPassengers() int setMaxPassengers (int):void toString():…arrow_forward
- CIST 1305 UNIT 10 DROP BOX ASSIGNMENT Create the class diagram and write the pseudocode for a class named Computer that holds the make, model, and amount of memory of a computer. Include methods to set the values for each data field, and include a method that displays all the values for each field.arrow_forward// NOTE: Two separate versions of the sequence (one for a sequence of real numbers and another for a sequence of characters are specified, in two separate namespaces in this header file. For both versions, the same documentation applies.// TYPEDEFS and MEMBER functions for the sequence class:// typedef ____ value_type// sequence::value_type is the data type of the items in the sequence.// It may be any of the C++ built-in types (int, char, etc.), or a// class with a default constructor, an assignment operator, and a// copy constructor.// typedef ____ size_type// sequence::size_type is the data type of any variable that keeps// track of how many items are in a sequence.// static const size_type CAPACITY = _____// sequence::CAPACITY is the maximum number of items that a// sequence can hold.//// CONSTRUCTOR for the sequence class:// sequence()// Pre: (none)// Post: The sequence has been initialized as an empty sequence.//// MODIFICATION…arrow_forward/ CONSTANT// static const int DEFAULT_CAPACITY = ____// IntSet::DEFAULT_CAPACITY is the initial capacity of an// IntSet that is created by the default constructor (i.e.,// IntSet::DEFAULT_CAPACITY is the highest # of distinct// values "an IntSet created by the default constructor"// can accommodate).//// CONSTRUCTOR// IntSet(int initial_capacity = DEFAULT_CAPACITY)// Post: The invoking IntSet is initialized to an empty// IntSet (i.e., one containing no relevant elements);// the initial capacity is given by initial_capacity if// initial_capacity is >= 1, otherwise it is given by// IntSet:DEFAULT_CAPACITY.// Note: When the IntSet is put to use after construction,// its capacity will be resized as necessary.//// CONSTANT MEMBER FUNCTIONS (ACCESSORS)// int size() const// Pre: (none)// Post: Number of elements in the invoking IntSet is returned.// bool isEmpty() const// Pre: (none)//…arrow_forward
- // CONSTANT// static const int DEFAULT_CAPACITY = __10__// IntSet::DEFAULT_CAPACITY is the initial capacity of an// IntSet that is created by the default constructor (i.e.,// IntSet::DEFAULT_CAPACITY is the highest # of distinct// values "an IntSet created by the default constructor"// can accommodate).//// CONSTRUCTOR// IntSet(int initial_capacity = DEFAULT_CAPACITY)// Post: The invoking IntSet is initialized to an empty// IntSet (i.e., one containing no relevant elements);// the initial capacity is given by initial_capacity if// initial_capacity is >= 1, otherwise it is given by// IntSet:DEFAULT_CAPACITY.// Note: When the IntSet is put to use after construction,// its capacity will be resized as necessary.//// CONSTANT MEMBER FUNCTIONS (ACCESSORS)// int size() const// Pre: (none)// Post: Number of elements in the invoking IntSet is returned.// bool isEmpty() const// Pre: (none)//…arrow_forwardPersonal Information Class: Design a class called Employee that holds the following data about an employee: name ID number Department Job Title Class. Store your class in a separate file called employee.py. Your class will have an initializer method that will be passed the information entered by the user as arguments. Write appropriate accessor and mutator methods for each data attribute. Write a __str__ method to print the contents of the class (see example of __str__ on p. 523). Main program: Your main program should create three instances of the class. Your program should get the information from the user and pass it as parameters to the initializer method. Using the __str__ method invoked by the print function, the program should display the personal information for the three individuals. Output and Sample Dialog: Enter employee name: Mary Smith Enter employee ID: 123456 Enter department: Accounting Enter position: Accountant Enter employee name: Joe Morales Enter…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
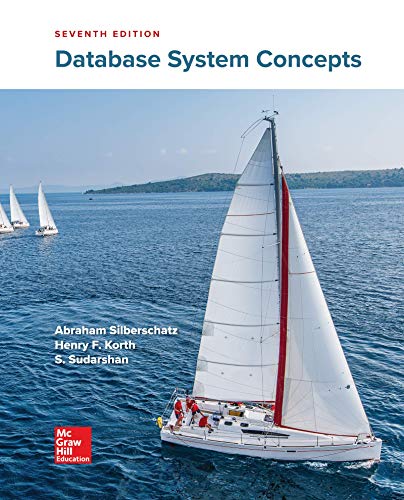
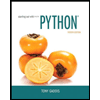
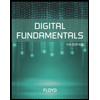
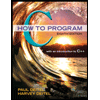
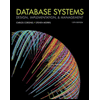
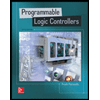