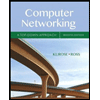
Task. Instead of using the default hashCode method to each key, create a hash function applying the folding method.
Within your hash function
Split the string into 3 sub-strings, based from the reg-ex "-"
Parse your sub-strings to Integer and add (folding method)
Parse the summed value to String
Initialize an int variable for your hash to 0
Loop to each character index of the String
Apply the general formula for hashing: hash=31*hash+character in that index position;
Append & 0xFFFFFFF to it to make the resulting hash positive
Convert the hash to index of tablesize=20 using the % operator
Return hash
What index has the most occurrence of collisions?

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- import numpy as npimport matplotlib.pyplot as pltfrom sklearn.cluster import KMeansfrom sklearn.cluster import DBSCAN from sklearn.datasets import make_blobs n_samples = [750,750,750] cluster_std = 1 random_state = 200 X, Y_true = make_blobs(n_samples=n_samples, random_state=random_state, cluster_std=cluster_std) plt.scatter(X[:, 0], X[:, 1], marker='.', c=Y_true) #DBSCAN model, setting up required parameters eps = 0.4min_Samples = 10db = DBSCAN(eps=eps, min_samples=min_Samples).fit(X)labels = db.labels_labels # To count number of clusters in labels, ignoring noise if present.n_clusters_ = len(set(labels)) - (1 if -1 in labels else 0)n_noise_ = list(labels).count(-1) print('Estimated number of clusters: %d' % n_clusters_)print('Estimated number of noise points: %d' % n_noise_) # Plot result# Use black to label noise points.unique_labels = set(labels)colors = plt.cm.Spectral(np.linspace(0, 1, len(unique_labels))) core_samples_mask = np.zeros_like(db.labels_,…arrow_forwarddef upgrade_stations(threshold: int, num_bikes: int, stations: List["Station"]) -> int: """Modify each station in stations that has a capacity that is less than threshold by adding num_bikes to the capacity and bikes available counts. Modify each station at most once. Return the total number of bikes that were added to the bike share network. Precondition: num_bikes >= 0 >>> handout_copy = [HANDOUT_STATIONS[0][:], HANDOUT_STATIONS[1][:]] >>> upgrade_stations(25, 5, handout_copy) 5 >>> handout_copy[0] == HANDOUT_STATIONS[0] True >>> handout_copy[1] == [7001, 'Lower Jarvis St SMART / The Esplanade', \ 43.647992, -79.370907, 20, 10, 10] True """arrow_forwardWrite a script that creates a dictionary of name-age pair. Add some entries to your dictionary. Add a menu to support ADD, REMOVE, SERACH, AGE INCREASE, PRINT operations.The “Age Increase” option increases the age by 1 for all the entries in the dictionary.Test the four operationsarrow_forward
- For Quick Sort, and when selecting a partition element via the "median of N elements" method, which of the following values for N would result in a better partition? 7 15 9. O 0O Oarrow_forwardA date-to-event dictionary is a dictionary in which the keys are dates (as strings in the format 'YYYY-MM-DD'), and the values are lists of events that take place on that day. Complete the following function according to its docstring. Your code must not mutate the parameter! def group_by_year (events: Dict[str, List[str]]) -> Dict [str, Dict [str, List[str]]]: """Return a dictionary in which in the keys are the years that appear in the dates in events, and the values are the date-to-event dictionaries for all dates in that year. >>> date_to_event = {'2018-01-17': ['meeting', 'lunch'], '2018-03-15': ['lecture'], '2017-05-04': ['gym', 'dinner']} >>> group_by_year (date_to_event) {'2018': {'2018-01-17': ['meeting', 'lunch'], '2018-03-15': ['lecture']}, '2017': {'2017-05-04': ['gym', 'dinner']} } || || ||arrow_forward( aList. insert ( i, item ) ).remove (i) = aListarrow_forward
- Please don't use answer already posted on other websites. will leave you feedback!! Thank you!arrow_forwardPlease answer the ques in python with showing the codesarrow_forwardWhat will be displayed after the following code executes? (Note: the order of the display of entries in a dictionary are not in a specific order.)arrow_forward
- This question is for Java, and in the picture provided.arrow_forwardPython question please include all steps and screenshot of code. Also please provide a docstring, and comments throughout the code, and test the given examples below. Thanks. Suppose that you are given a list of strings representing a deck of 52 cards. Write afunction called bridgeHands() which takes the list as its only parameter. It shuffles the listand divides it into 4 hands (tuples) of 13 cards each. It returns a dictionary in which thekeys are the strings 'North', 'South', 'East', and 'West', with each key corresponding to adifferent one of the hands.arrow_forwardJava - Given list: ( 4, 7, 18, 48, 49, 56, 66, 68, 79 )arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
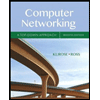
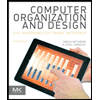
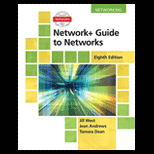
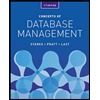
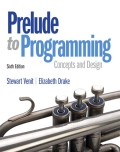
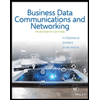