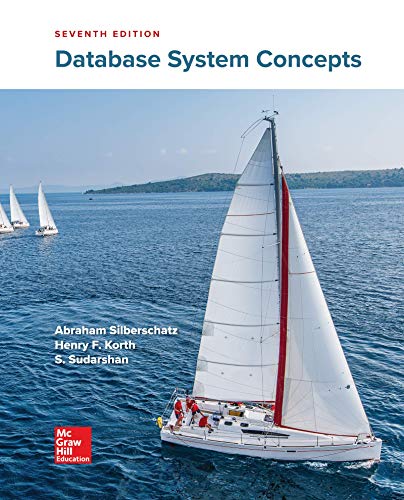
Revorse the vewels
def reverse_vowels(text):
Given a text string, create and return a new string constructed by finding all its vowels (for simplicity, in this problem vowels are the letters found in the string 'aeiouAEIOU') and reversing their order, while keeping all other characters exactly as they were in their original positions.
However, to make the result look prettier, the capitalization of each moved vowel must be the same as that of the vowel that was originally in the target position. For example, reversing the vowels of 'Ilkka' should produce 'Alkki' instead of 'alkkI'. Applying this operation to random English sentences seems to occasionally give them a curious pseudo-Mediterranean vibe.
Along with many possible other ways to perform this square dance, one straightforward way to reverse the vowels starts with collecting all vowels of text into a separate list, and initializing the result to an empty string. After that, iterate through all positions of the original text. Whenever
the current position contains a vowel, take one from the end of the list of the vowels. Convert that vowel to either upper- or lowercase depending on the case of the vowel that was originally in that position, and append it to result. Otherwise, append the character from the same position of the
original text to the result as it were.
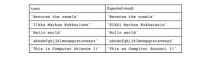

Step by stepSolved in 3 steps with 1 images

- %matplotlib inlineimport numpy as npfrom matplotlib import pyplot as pltimport math xs = np.array([0. , 0.01010101, 0.02020202, 0.03030303, 0.04040404, 0.05050505, 0.06060606, 0.07070707, 0.08080808, 0.09090909, 0.1010101 , 0.11111111, 0.12121212, 0.13131313, 0.14141414, 0.15151515, 0.16161616, 0.17171717, 0.18181818, 0.19191919, 0.2020202 , 0.21212121, 0.22222222, 0.23232323, 0.24242424, 0.25252525, 0.26262626, 0.27272727, 0.28282828, 0.29292929, 0.3030303 , 0.31313131, 0.32323232, 0.33333333, 0.34343434, 0.35353535, 0.36363636, 0.37373737, 0.38383838, 0.39393939, 0.4040404 , 0.41414141, 0.42424242, 0.43434343, 0.44444444, 0.45454545, 0.46464646, 0.47474747, 0.48484848, 0.49494949, 0.50505051, 0.51515152, 0.52525253, 0.53535354, 0.54545455, 0.55555556, 0.56565657, 0.57575758, 0.58585859, 0.5959596 , 0.60606061, 0.61616162, 0.62626263, 0.63636364, 0.64646465, 0.65656566, 0.66666667,…arrow_forwardStringBuilder objects should be used in place of String objects if O is more than 80 characters long. the string may grow. O the string is declared final. O the tring needs to be initialized.arrow_forwardChange this code to show strings that help you cast twelve dice. They should be shown one at a time like this:The first one was A. The second one was B. Afterward, the sum should be shown on a different line.arrow_forward
- zeroTriples.py: Write a program that reads a list of integers from the user, until they enter -12345 (the -12345 should not be considered part of the list). Then find all triples in the list that sum to zero. You can assume the list won’t contain any duplicates, and a triple should not use the same number more than once. For example:$ python3 zeroTriples.py124-123450 triples found $ python3 zeroTriples.py-3142-123451 triple found:1, 2, -3 $ python zeroTriples.py-91-3245-4-1-123454 triples found:-9, 4, 51, -3, 2-3, 4, -15, -4, -1arrow_forwardWrite a program that animates the linear search algorithm. Create a list that consists of 20 distinct numbers from 1 to 20 in a random order. The elements are displayed in a histogram, as shown in Figure 10.17. You need to enter a search key in the text field. Clicking the Step button causes the program to perform one comparison in the algorithm and repaints the histogram with a bar indicating the search position. When the algorithm is finished, display a dialog box to inform the user. Clicking the Reset button creates a new random list for a new start.arrow_forward1c) Average sentence length We will create a function ( avg_sentence_len ) to calculate the average sentence length across a piece of text. This function should take text as an input parameter. Within this function: 1. sentences : Use the split() string method to split the input text at every '.'. This will split the text into a list of sentences. Store this in the variable sentences . To keep things simple, we will consider every "." as a sentence separator. (This decision could lead to misleading answers. For example, "Hello Dr. Jacob." is actually a single sentence, but our function will consider this 2 separate sentences). 2. words : Use the split() method to split the input text into a list of separate words, storing this in words . Again, to limit complexity, we will assume that all words are separated by a single space (" "). (So, while "I am going.to see you later" actually has 7 words, since there is no space after the ".", so we will assume the this to contain 6 separate…arrow_forward
- For any element in keysList with a value greater than 50, print the corresponding value in itemsList, followed by a comma (no spaces). Ex: If the input is: 32 105 101 35 10 20 30 40 the output is: 20,30, 1 #include 2 3 int main(void) { const int SIZE_LIST = 4; int keysList[SIZE_LIST]; int itemsList[SIZE_LIST]; int i; 4 6 7 8 scanf("%d", &keysList[0]); scanf ("%d", &keysList[1]); scanf("%d", &keysList[2]); scanf("%d", &keysList[3]); 10 11 12 13 scanf ("%d", &itemsList[0]); scanf ("%d", &itemsList[1]); scanf("%d", &itemsList[2]); scanf ("%d", &itemsList[3]); 14 15 16 17 18 19 /* Your code goes here */ 20 21 printf("\n"); 22 23 return 0; 24 }arrow_forwardWrite a code in python for this exercise belowarrow_forwardplease name this cards.py. You may use the following code in your program: import random SUITS = "♠ ♡ ♢ ♣".split()RANKS = "2 3 4 5 6 7 8 9 10 J Q K A".split() def create_deck(shuffle=False): """Create a new deck of 52 cards""" deck = [(s, r) for r in RANKS for s in SUITS] if shuffle: random.shuffle(deck) return deck This code creates a random deck of cards (each card is a tuple consisting of a rank and suit). You are to ask the user how many 5-card hands to play and will keep track of the following poker hands. You will need to reshuffle the deck as many times as necessary to get the required number of hands. An example run of the program is as follows. Enter the number of hands to deal: 10000Hand % of Hands Hands of Type2 of a kind 41.290% 4129 3 of a kind 1.970% 197 4 of a kind 0.010% 1 2 pair 4.860% 486 Full house 0.150% 15 Flush 0.220% 22 If you deal enough…arrow_forward
- Please do not use string methods except to find methodarrow_forwardPython write a program in python that plays the game of Hangman. When the user plays Hangman, the computer first selects a secret word at random from a list built into the program. The program then prints out a row of dashes asks the user to guess a letter. If the user guesses a letter that is in the word, the word is redisplayed with all instances of that letter shown in the correct positions, along with any letters correctly guessed on previous turns. If the letter does not appear in the word, the user is charged with an incorrect guess. The user keeps guessing letters until either: * the user has correctly guessed all the letters in the word or * the user has made eight incorrect guesses. one for each letter in the secret word and Hangman comes from the fact that incorrect guesses are recorded by drawing an evolving picture of the user being hanged at a scaffold. For each incorrect guess, a new part of a stick-figure body the head, then the body, then each arm, each leg, and finally…arrow_forwardpython code easy way pleasethe one that i provide the image with code is just starting codearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
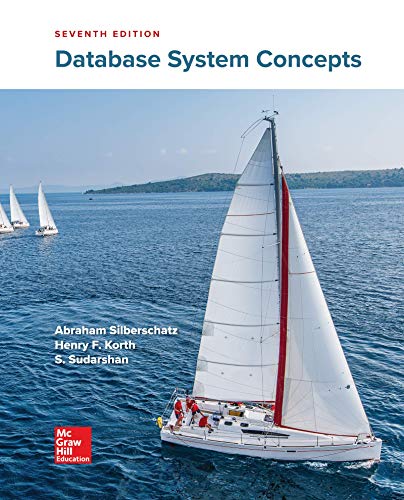
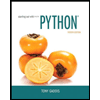
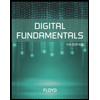
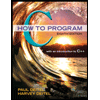
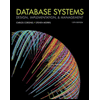
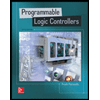