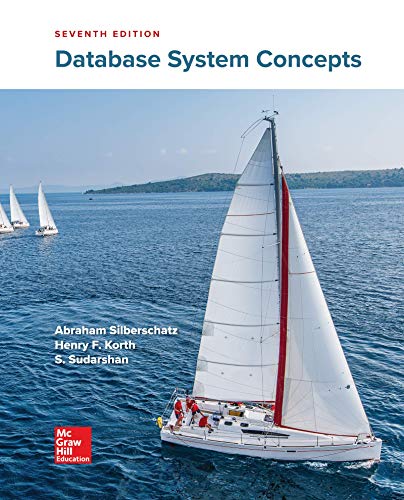
Concept explainers
Collapse positive integer intervals
def collapse_intervals(items):This function is the inverse of the previous problem of expanding positive integer intervals. Given a nonempty list of positive integer items guaranteed to be in sorted ascending order, create and return the unique description string where every maximal sublist of consecutive integers has been condensed to the notation first-last. Such encoding doesn’t actually save any characters when first and last differ by only one. However, it is usually more important for the encoding to be uniform than to be pretty. As a general principle, uniform and consistent encoding of data allows the processing of that data to also be uniform in the tools down the line.
If some maximal sublist consists of a single integer, it must be included in the result string all by itself without the minus sign separating it from the now redundant last number. Make sure that the string returned by your function does not contain any whitespace characters, and that it does not have a silly redundant comma hanging at the end.
items |
Expected result |
[1, 2, 4, 6, 7, 8, 9, 10, 12, 13]
|
'1-2,4,6-10,12-13'
|
[42] |
'42' |
[3, 5, 6, 7, 9, 11, 12, 13]
|
'3,5-7,9,11-13'
|
[] |
'' |
range(1, 1000001)
|
'1-1000000' |

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- A map is a container that stores a collection of ordered pairs, each pair consists of a key and a value, <key, value>. Keys must be unique. Values need not be unique, so several keys can map to the same values. The pairs in the map are sorted based on keys. Group of answer choices True Falsearrow_forwardFIX THIS CODE Using python Application CODE: import csv playersList = [] with open('Players.csv') as f: rows = csv.DictReader(f) for r in rows: playersList.append(r) teamsList = [] with open('Teams.csv') as f: rows = csv.DictReader(f) for r in rows: teamsList.append(r) plays=len(playersList) for i in range(plays): if playersList[i]['team']=='Argentina' and int(playerlist[i]['minutes played'])<200 and int(playersList[i] ['shots'])>20: print(playersList[i]['last name']) c0=0 c1=0 c2=0 for i in range(len(teamsList)): if int(teamsList[i]['redCards'])==0: c0=c0+1 if int(teamsList[i]['redCards'])==1: c1=c1+1 if int(teamsList[i]['redCards'])==2: c2=c2+1 print("Number of teams with zero redcards:",c0) print("Number of teams with zero redcards:",c1) print("Number of teams with zero redcards:",c2) ratio=0 for i in range(len(teamsList)): if int(teamsList[i]['games']>3) and if int(teamsList[i]['goalsFor'])/int(teamsList[i]['goalsAgainst'])<ratio:…arrow_forwarddef makeRandomList(size): lyst = [] for count in range(size): while True: number = random.randint(1, size) if not number in lyst: lyst.append(number) break return lyst give me proper analysis of this code and Big Oarrow_forward
- import numpy as np%matplotlib inlinefrom matplotlib import pyplot as pltimport mathfrom math import exp np.random.seed(9999)def is_good_peak(mu, min_dist=0.8): if mu is None: return False smu = np.sort(mu) if smu[0] < 0.5: return False if smu[-1] > 2.5: return False for p, n in zip(smu, smu[1:]): #print(abs(p-n)) if abs(p-n) < min_dist: return False return True maxx = 3ndata = 500nset = 10l = []answers = []for iset in range(1, nset): npeak = np.random.randint(2,4) xs = np.linspace(0,maxx,ndata) ys = np.zeros(ndata) mu = None while not is_good_peak(mu): mu = np.random.random(npeak)*maxx for ipeak in range(npeak): m = mu[ipeak] sigma = np.random.random()*0.3 + 0.2 height = np.random.random()*0.5 + 1 ys += height*np.exp(-(xs-m)**2/sigma**2) ys += np.random.randn(ndata)*0.07 l.append(ys) answers.append(mu) p6_ys = lp6_xs =…arrow_forwardDescription: Given a string, find the first non-repeating character in it and return its index. If it doesn't exist, return -1. Example 1 input: "leetcode" output: 0 Example 2 input: "loveleetcode" output: 2 The solution we are targeting here is a linear time solution since we have to go over the entire string to know which one is unique in it. A straightforward method we can easily think of is having a Dictionary record letter frequency. Subsequently, iterate over the input again and return the index that has a letter frequency of 1.arrow_forwardIntegers are read from input and stored into a vector until 0 is read. If the vector's last element is odd, output the odd elements in the vector. Otherwise, output the even elements in the vector. End each number with a newline. Ex: If the input is -9 12 -6 1 0, the vector's last element is 1. Thus, the output is: -9 1 Note: (x % 2 != 0) returns true if x is odd. int value; int i; bool isodd; 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 } cin >> value; while (value != 0) { } for (i = 0; i > value; Check return 0; 1 Next level 2 X 1: Compare output 3 4 For input -9 12 -6 1 0, the vector elements are -9, 12, -6, and 1. The last element, 1, is odd. The odd elements in the vector, -9 and 1, are output, each on a new line. Not all tests passed. 2 V 3arrow_forward
- Create class Test in a file named Test.java. This class contains a main program that performs the following actions: Instantiate a doubly linked list. Insert strings “a”, “b”, and “c” at the head of the list using three Insert() operations. The state of the list is now [“c”, “b”, “a”]. Set the current element to the second-to-last element with a call to Tail() followed by a call to Previous()Then insert string “d”. The state of the list is now [“c”, “d”, “b”, “a”]. Set the current element to past-the-end with a call to Tail() followed by a call to Next(). Then insert string “e”. The state of the list is now [“c”, “d”, “b”, “a”, “e”] . Print the list with a call to Print() and verify that the state of the list is correct.arrow_forwardIntegers are read from input and stored into a vector until 0 is read. If the vector's last element is odd, output the odd elements in the vector. Otherwise, output the even elements in the vector. End each number with a newline. Ex: If the input is -9 12 -6 1 0, the vector's last element is 1. Thus, the output is: -9 1 Note: (x % 2!= 0) returns true if x is odd. 4 5 int main() { 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20} vector vect1; int value; int i; bool isodd; cin >> value; while (value != 0) { vect1.push_back(value); cin >> value; } V* Your code goes here */ return 0; 2 3arrow_forwardPython Code Create a code that can plot a distance versus time graph by importing matplotlib and appending data from a text file to a list. Follow the algorithm: Import matplotlib. Create two empty lists: Time = [ ] and Distance = [ ] Open text file named Motion.txt (content attached). Append data from Motion.txt such that the first column is placed in Time list and the second column is placed in Distance list. Plot the lists (Distance vs Time Graph). You may use this following link as a source for matplotlib functions: https://datatofish.com/line-chart-python-matplotlib/ Show Plot.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
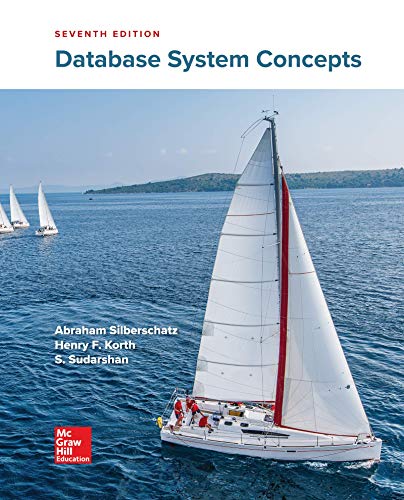
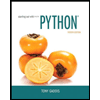
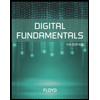
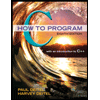
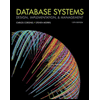
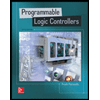