Code Example 18-2 1. import tkinter as tk 2. from tkinter import ttk 3. class TipCalcFrame(ttk.Frame): 4. def __init__(self, parent): 5. ttk.Frame.__init__(self, parent, padding="20 20 20 20") 6. self.pack() 7. self.mealCost = tk.StringVar() 8. self.tipPercent = tk.StringVar() 9. self.tipAmount = tk.StringVar() 10. ttk.Label(self, text="Cost of your meal:").grid( column=0, row=0, sticky=tk.E) 11. ttk.Entry(self, width=30, textvariable=self.mealCost).grid( column=1, row=0) 12. ttk.Label(self, text="Percent to leave as a tip:").grid( column=0, row=1, sticky=tk.E) 13. ttk.Entry(self, width=30, textvariable=self.tipPercent).grid( column=1, row=1) 14. ttk.Label(self, text="Tip amount:").grid( column=0, row=2, sticky=tk.E) 15. ttk.Entry(self, width=30, textvariable=self.tipAmount, state="readonly").grid(column=1, row=2) 16. ttk.Button(self, text="Calculate", command=self.calculate).grid(column=1,row=3, sticky=tk.E) 17. for child in self.winfo_children(): 18. child.grid_configure(padx=5, pady=3) 19. def calculate(self): 20. mealCost = float(self.mealCost.get()) 21. tipPercent = float(self.tipPercent.get()) 22. tipPercent = tipPercent/100 23. tip = mealCost * tipPercent 24. yourTip = "$" + str(round(tip, 2)) 25. self.tipAmount.set(yourTip) 26. if __name__ == "__main__": 27. root = tk.Tk() 28. root.title("Tip Calculator") 29. TipCalcFrame(root) 30. root.mainloop() Refer to Code Example 18-2: How many visible components does this code display in the window? a. 8 b. 5 c. 7 d. 6
Code Example 18-2 1. import tkinter as tk 2. from tkinter import ttk 3. class TipCalcFrame(ttk.Frame): 4. def __init__(self, parent): 5. ttk.Frame.__init__(self, parent, padding="20 20 20 20") 6. self.pack() 7. self.mealCost = tk.StringVar() 8. self.tipPercent = tk.StringVar() 9. self.tipAmount = tk.StringVar() 10. ttk.Label(self, text="Cost of your meal:").grid( column=0, row=0, sticky=tk.E) 11. ttk.Entry(self, width=30, textvariable=self.mealCost).grid( column=1, row=0) 12. ttk.Label(self, text="Percent to leave as a tip:").grid( column=0, row=1, sticky=tk.E) 13. ttk.Entry(self, width=30, textvariable=self.tipPercent).grid( column=1, row=1) 14. ttk.Label(self, text="Tip amount:").grid( column=0, row=2, sticky=tk.E) 15. ttk.Entry(self, width=30, textvariable=self.tipAmount, state="readonly").grid(column=1, row=2) 16. ttk.Button(self, text="Calculate", command=self.calculate).grid(column=1,row=3, sticky=tk.E) 17. for child in self.winfo_children(): 18. child.grid_configure(padx=5, pady=3) 19. def calculate(self): 20. mealCost = float(self.mealCost.get()) 21. tipPercent = float(self.tipPercent.get()) 22. tipPercent = tipPercent/100 23. tip = mealCost * tipPercent 24. yourTip = "$" + str(round(tip, 2)) 25. self.tipAmount.set(yourTip) 26. if __name__ == "__main__": 27. root = tk.Tk() 28. root.title("Tip Calculator") 29. TipCalcFrame(root) 30. root.mainloop() Refer to Code Example 18-2: How many visible components does this code display in the window? a. 8 b. 5 c. 7 d. 6
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
-
Code Example 18-2
1. import tkinter as tk
2. from tkinter import ttk
3. class TipCalcFrame(ttk.Frame):
4. def __init__(self, parent):
5. ttk.Frame.__init__(self, parent, padding="20 20 20 20")
6. self.pack()
7. self.mealCost = tk.StringVar()
8. self.tipPercent = tk.StringVar()
9. self.tipAmount = tk.StringVar()
10. ttk.Label(self, text="Cost of your meal:").grid(
column=0, row=0, sticky=tk.E)
11. ttk.Entry(self, width=30, textvariable=self.mealCost).grid(
column=1, row=0)
12. ttk.Label(self, text="Percent to leave as a tip:").grid(
column=0, row=1, sticky=tk.E)
13. ttk.Entry(self, width=30, textvariable=self.tipPercent).grid(
column=1, row=1)
14. ttk.Label(self, text="Tip amount:").grid(
column=0, row=2, sticky=tk.E)
15. ttk.Entry(self, width=30, textvariable=self.tipAmount,
state="readonly").grid(column=1, row=2)
16. ttk.Button(self, text="Calculate",
command=self.calculate).grid(column=1,row=3, sticky=tk.E)
17. for child in self.winfo_children():
18. child.grid_configure(padx=5, pady=3)
19. def calculate(self):
20. mealCost = float(self.mealCost.get())
21. tipPercent = float(self.tipPercent.get())
22. tipPercent = tipPercent/100
23. tip = mealCost * tipPercent
24. yourTip = "$" + str(round(tip, 2))
25. self.tipAmount.set(yourTip)
26. if __name__ == "__main__":
27. root = tk.Tk()
28. root.title("Tip Calculator")
29. TipCalcFrame(root)
30. root.mainloop()
Refer to Code Example 18-2: How many visible components does this code display in the window?a. 8b. 5c. 7d. 6
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
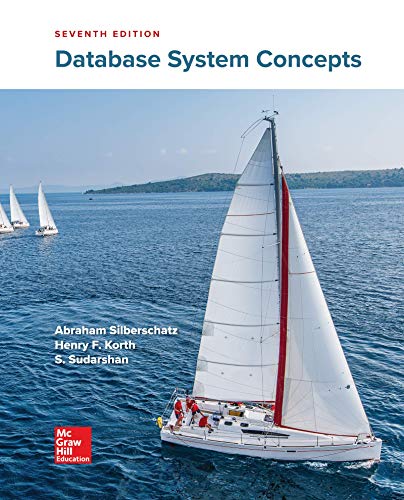
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
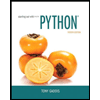
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
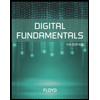
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
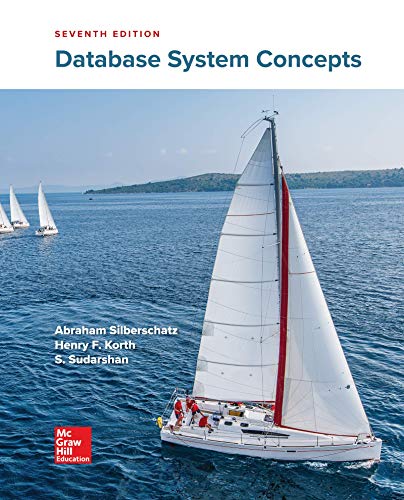
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
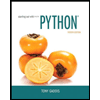
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
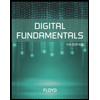
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
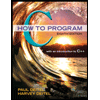
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
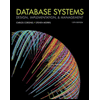
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
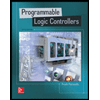
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education