Class: Desk Data members: weight (integer) Write all external function definition required to make the following code compile: Desk d1{30}, d2{}; cin >> d2; if( d1 > d2 ) cout << (++d2) << "\n"; Include a comment for each function indicating if it is a memb
need help with c++
Class: Desk
Data members: weight (integer)
Write all external function definition required to make the following code compile:
Desk d1{30}, d2{};
cin >> d2;
if( d1 > d2 )
cout << (++d2) << "\n";
Include a comment for each function indicating if it is a member, non-member, or friend overload for clarity.

#include <iostream>
using namespace std;
class Desk{
public:
int weight;
Desk(int w){
weight=w;
}
Desk(){
}
//overloading gretarer then operator
int operator >(Desk d){
if(weight>d.weight)
return 1;
else
return 0;
}
//overloading preincrement operator
int operator++(){
++weight;
return weight;
}
//overloading >> operator
friend istream &operator>>(istream &in,Desk &d){
in>>d.weight;
return in;
}
};
int main()
{
Desk d1(30),d2;
cin>>d2;
if(d1>d2)
cout<<++d2;
return 0;
}
Step by step
Solved in 2 steps with 1 images

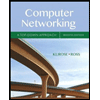
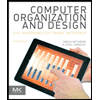
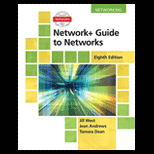
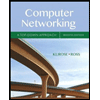
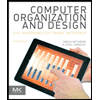
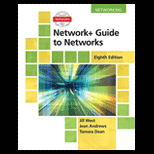
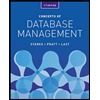
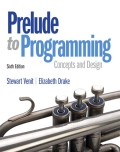
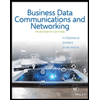