Check this code then covert it to c++ import java.io.*; import java.util.Scanner; class Main { public static void main(String[] args) { Scanner sc = new Scanner(System.in); try { System.out.println("Please enter the obtained and total grade: "); int[] integers = new int[2]; for(int i = 0; i < 2; i++) { integers[i] = sc.nextInt(); } double percent = (double)integers[0]/(double)integers[1]*100.00; System.out.println("Percentage: " + percent); if (integers[0] < 0 || integers[1]<=0) { // this gets caught in the catch block throw new IllegalArgumentException("Only Positive Grades allowed"); } if (integers[0]>integers[1]) { // this gets caught in the catch block throw new IllegalArgumentException("Obtained grade must be less than total grade"); } } catch (ArithmeticException e) { System.out.println( "Zero cannot divide any number"); } catch (ArrayIndexOutOfBoundsException e) { System.out.println( "Index out of size of the array"); } } }
Check this code then covert it to c++
import java.io.*;
import java.util.Scanner;
class Main {
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
try {
System.out.println("Please enter the obtained and total grade: ");
int[] integers = new int[2];
for(int i = 0; i < 2; i++)
{
integers[i] = sc.nextInt();
}
double percent = (double)integers[0]/(double)integers[1]*100.00;
System.out.println("Percentage: " + percent);
if (integers[0] < 0 || integers[1]<=0)
{
// this gets caught in the catch block
throw new IllegalArgumentException("Only Positive Grades allowed");
}
if (integers[0]>integers[1])
{
// this gets caught in the catch block
throw new IllegalArgumentException("Obtained grade must be less than total grade");
}
}
catch (ArithmeticException e) {
System.out.println(
"Zero cannot divide any number");
}
catch (ArrayIndexOutOfBoundsException e) {
System.out.println(
"Index out of size of the array");
}
}
}

Step by step
Solved in 2 steps with 1 images

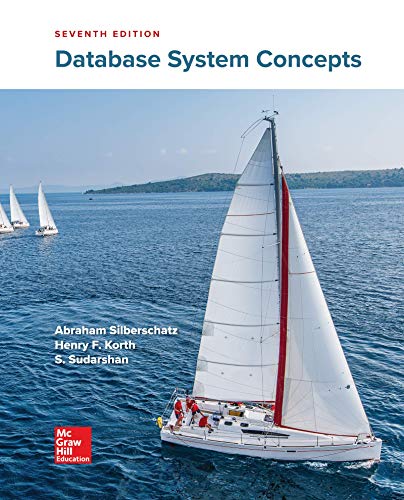
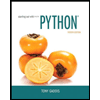
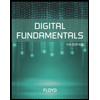
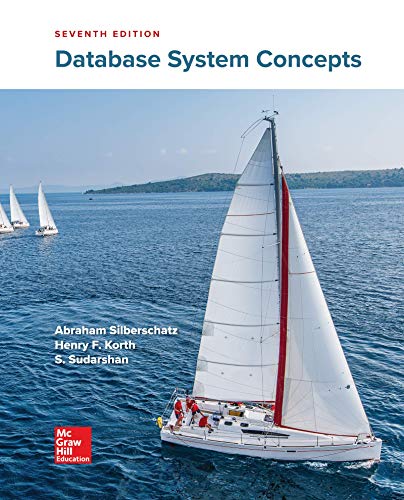
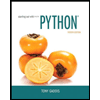
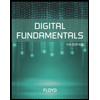
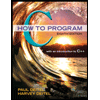
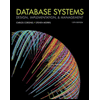
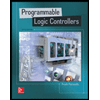