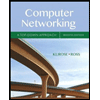
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
Can you complete?
public class Student {
// TODO: Build Student class with private fields and methods listed above
// TODO: Define two private member fields
public Student() {
// TODO: Define default constructor
}
public void setName(String n) {
// TODO: Assign parameter to instance field
}
// TODO: Add three more methods
public static void main(String[] args) {
Student student = new Student();
System.out.println(student.getName() + "/" + student.getGPA());
student.setName("Felix");
student.setGPA(3.7);
System.out.println(student.getName() + "/" + student.getGPA());
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Similar questions
- 2. The MyInteger Class Problem Description: Design a class named MyInteger. The class contains: n [] [] A private int data field named value that stores the int value represented by this object. A constructor that creates a MyInteger object for the specified int value. A get method that returns the int value. Methods isEven () and isOdd () that return true if the value is even or odd respectively. Static methods isEven (int) and isOdd (int) that return true if the specified value is even or odd respectively. Static methods isEven (MyInteger) and isOdd (MyInteger) that return true if the specified value is even or odd respectively. Methods equals (int) and equals (MyInteger) that return true if the value in the object is equal to the specified value. Implement the class. Write a client program that tests all methods in the class.arrow_forwardGiven the following class definition: class employee{public: employee(); employee(string, int, double); employee(int, double); employee(string); void setData(string, int, double); void print() const; void updatePay(double); int getNumOfServiceYears() const; double getPay() const;private: string name; int numOfServiceYears; double pay;}; Create a class parttime derived from the above class that includes private members payRate and hoursWorked of type double along with a constructor and the definition of a print function that prints out all information from the base and derived class. Provide a test program that would demonstrate the working parttime class. Note: You can assume all code is stored in a single file.arrow_forwardStockList Class • This class will contain the methods necessary to display various information about the stock data. • This class shall be a subclass of ArrayList. • This class shall not contain any data fields. (You don't need them for this class if done correctly). • This class shall have a default constructor with no parameters. o No other constructors are allowed. • This class shall have the following public, non-static, methods: • printAllStocks: This method shall take no parameters and return nothing. ▪ This method should print each stock in the StockList to an output file. ▪ NOTE: This method shall NOT print the data to the console. The output file shall be called all_stock_data.txt. The data should be printed in a nice and easy to read format. o displayFirstTenStocks: This method shall take no parameters and return nothing. ▪ This method should display only the first 10 stocks in the StockList in a nice and easy to read format. ▪ This method should display the data in the…arrow_forward
- public class Artwork { // TODO: Declare private fields - title, yearCreated // TODO: Declare private field artist of type Artist // TODO: Define default constructor // TODO: Define get methods: getTitle(), getYearCreated() // TODO: Define second constructor to initialize // private fields (title, yearCreated, artist) // TODO: Define printInfo() method // Call the printInfo() method in Artist.java to print an artist's information }} public class ArtworkLabel { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String userTitle, userArtistName; int yearCreated, userBirthYear, userDeathYear; userArtistName = scnr.nextLine(); userBirthYear = scnr.nextInt(); scnr.nextLine(); userDeathYear = scnr.nextInt(); scnr.nextLine(); userTitle = scnr.nextLine(); yearCreated =…arrow_forwardWritten in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forwardpublic class Plant { protected String plantName; protected String plantCost; public void setPlantName(String userPlantName) { plantName = userPlantName; } public String getPlantName() { return plantName; } public void setPlantCost(String userPlantCost) { plantCost = userPlantCost; } public String getPlantCost() { return plantCost; } public void printInfo() { System.out.println(" Plant name: " + plantName); System.out.println(" Cost: " + plantCost); }} public class Flower extends Plant { private boolean isAnnual; private String colorOfFlowers; public void setPlantType(boolean userIsAnnual) { isAnnual = userIsAnnual; } public boolean getPlantType(){ return isAnnual; } public void setColorOfFlowers(String userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } public String getColorOfFlowers(){ return colorOfFlowers; } @Override public void printInfo(){…arrow_forward
- Classes: Write a Person class that has these attributes: person_ID, first and last names, and age Default and overloaded constructors Accessors and mutators equals method toString method (make this virtual if C++, don't forget to prep the class for polymorphism) Inheritance: Create a child class to Person called Student: Attributes: GPA and status (freshman, sophomore, junior, senior, graduate, graduated). Make sure you have appropriate accessor/mutator methods Create another child class to represent Faculty. This class will have faculty rank and length of service as attributes along with an office location. Again, add methods as needed. Application Create an application that displays a menu that allows users to add students or faculty, or print either one or exit. Deliverable: Submit your source code and classes on Github (you will be supplied an account) You will also submit a Word document and your code on Canvas. In the document you iwll write a summary of your design…arrow_forwardQuestion 6 Consider: class Bike { } private String color; public Bike() { } public Bike(String newColor) { color = newColor; } public Bike (Bike bike) { color = } bike.color; public String getColor() { } } return color; public void setColor(String newColor) { color = newColor; Bike bike1= new Bike(); = Bike bike2 new Bike("blue"); Bike bike3 = new Bike(bike2); bike2.setColor("green"); Bike bike4 = new Bike(bike2); Which of the following are correct? bike4.getColor() returns null bike4.getColor() return "blue" bike3.getColor() return "green" bike2.getColor() returns "blue" bike4.getColor() return "green" bike1.getColor() returns bike3.getColor() return null bike3.getColor() returns "blue" bike2.getColor() returns "green" bike1.getColor() returns nullarrow_forwardComputer programmingarrow_forward
- 2. Student (extend the class Person) that has the following: Data Fields: private int ID prvate double grade Constructor: Constructor to create a Student object with specifiedname address, ID & grade. Methods: Accessor and mutator methods for all data fields.arrow_forwardIncorrect Question 4 Consider public class Species { } // fields private String name; private int population; private double growthRate; // constructor public Species () { } name = "No Name Yet"; population = 0; growthRate = 33.3; Which of the following is the best setter method for the population instance variable? public boolean setPopulation(int newPopulation) { } if (newPopulation>= 0) { } population = newPopulation; return false; } return true; public public void setPopulation (int newPopulation) if (newPopulation>= 0) { } population newPopulation; public void setPopulation (int newPopulation) { population } = newPopulation;arrow_forwardCode the StudentAccount class according to the class diagramMethods explanation:pay(double): doubleThis method is called when a student pays an amount towards outstanding fees. The balance isreduced by the amount received. The updated balance is returned by the method.addFees(double): doubleThis method is called to increase the balance by the amount received as a parameter. The updatedbalance is returned by the method.refund(): voidThis method is called when any monies owned to the student is paid out. This method can onlyrefund a student if the balance is negative. It displays the amount to be refunded and set thebalance to zero. (Use Math.abs(double) in your output message). If there is no refund, display anappropriate message.Test your StudentAccount class by creating objects and calling the methods addFees(), pay() andrefund(). Use toString() to display the object’s data after each method calledarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
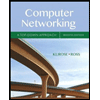
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
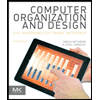
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
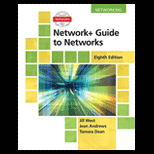
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
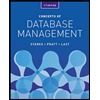
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
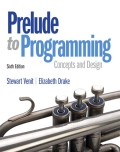
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
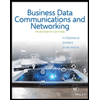
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY