Can someone please help me with the following coding given based on the instructions (//*** in bold)? I am not sure on how to go about it here. Just as a side note, this is coding that is going to be used for a working Yahtzee program. I am also using the IntelliJ IDEA application for this code. Thanks for the help! public static int calculateUpperSectionCategory(int dieNumber) { int score = 0; //*** //*** INSTRUCTIONS FOR CODE FOR YOU TO WRITE //*** //*** 1) Replace these five if-statement structures with the code in Steps 2 & 3: //*** //*** if (die1 == dieNumber) //*** score += die1; //*** //*** if (die2 == dieNumber) //*** score += die2; //*** //*** if (die3 == dieNumber) //*** score += die3; //*** //*** if (die4 == dieNumber) //*** score += die4; //*** //*** if (die5 == dieNumber) //*** score += die5; //*** //*** 2) In place of the code in Step 1, write a for-loop that iterates over every element in the array variable "dice". //*** //*** 3) Code block for the for-loop in Step 2: //*** A) Using the for-loop's control variable as an index into array variable "dice", write an if-statement that checks the value of each element in the array variable "dice" for "equality to" parameter variable "dienumber". //*** B) If the condition in Step 3A evaluates to true, then accumulate the value of that die into variable "score". //*** //*** NOTE: 1) Array variable "dice" replaces all of the individual die variables (e.g. die1, die2, etc). //*** 2) You are replacing the five if-statement structures that use the individual die variables with a for-loop that contains a nested if-statement structure that references into array variable "dice". //*** //*** This can be as few as three lines of code not including any curly braces. //***
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
Can someone please help me with the following coding given based on the instructions (//*** in bold)? I am not sure on how to go about it here. Just as a side note, this is coding that is going to be used for a working Yahtzee program. I am also using the IntelliJ IDEA application for this code. Thanks for the help!
public static int calculateUpperSectionCategory(int dieNumber) {
int score = 0;
//***
//*** INSTRUCTIONS FOR CODE FOR YOU TO WRITE
//***
//*** 1) Replace these five if-statement structures with the code in Steps 2 & 3:
//***
//*** if (die1 == dieNumber)
//*** score += die1;
//***
//*** if (die2 == dieNumber)
//*** score += die2;
//***
//*** if (die3 == dieNumber)
//*** score += die3;
//***
//*** if (die4 == dieNumber)
//*** score += die4;
//***
//*** if (die5 == dieNumber)
//*** score += die5;
//***
//*** 2) In place of the code in Step 1, write a for-loop that iterates over every element in the array variable "dice".
//***
//*** 3) Code block for the for-loop in Step 2:
//*** A) Using the for-loop's control variable as an index into array variable "dice", write an if-statement that checks the value of each element in the array variable "dice" for "equality to" parameter variable "dienumber".
//*** B) If the condition in Step 3A evaluates to true, then accumulate the value of that die into variable "score".
//***
//*** NOTE: 1) Array variable "dice" replaces all of the individual die variables (e.g. die1, die2, etc).
//*** 2) You are replacing the five if-statement structures that use the individual die variables with a for-loop that contains a nested if-statement structure that references into array variable "dice".
//***
//*** This can be as few as three lines of code not including any curly braces.
//***

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

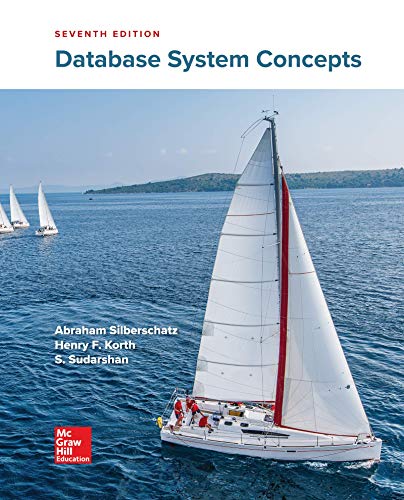
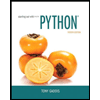
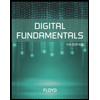
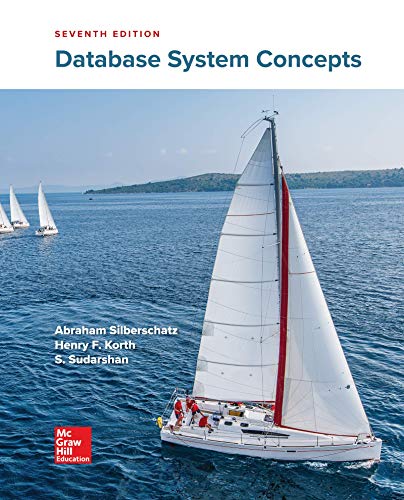
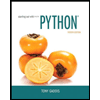
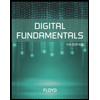
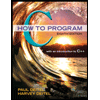
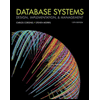
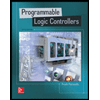