Can someone help me with this code, I not really understanding how to do this?
Can someone help me with this code, I not really understanding how to do this?
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
/**
* @version Spring 2019
* @author Kyle
*/
public class MapProblems {
/**
* Modify and return the given map as follows: if the key "a" has a value, set the key "b" to
* have that value, and set the key "a" to have the value "". Basically "b" is confiscating the
* value and replacing it with the empty string.
*
* @param map to be edited
* @return map
*/
public Map<String, String> confiscate(Map<String, String> map) {
if (map.containsKey("a") && map.containsValue("a")) {
}
}
/**
* Modify and return the given map as follows: if the key "duck" has a value, set the key
* "goose" to have that same value. In all cases remove the key "swan", the rest of the map
* should not change.
*
* @param map to be edited
* @return map
*/
public Map<String, String> mapBird1(Map<String, String> map) {
throw new UnsupportedOperationException("Not supported yet");
}
/**
* Given a map of bird keys and topping values, modify and return the map as follows: if the key
* "turkey" has a value, set that as the value for the key "chicken". If the key "vulture" has a
* value, set that as the value for the key "buzzard".
*
* @param map to be edited
* @return map
*/
public Map<String, String> mapBird2(Map<String, String> map) {
throw new UnsupportedOperationException("Not supported yet");
}
/**
* Given an array of strings, return a Map<String, Integer> with a key for each different
* string, with the value the number of times that string appears in the array.
*
* @param strings array
* @return map
*/
public Map<String, Integer> wordCount(ArrayList<String> strings) {
throw new UnsupportedOperationException("Not supported yet");
}
/**
* Given an ArrayList of Strings, create a new map that creates a key for each String by
* multiplying it's length by 7. If a key is already being used do not add the new value.
*
* @param str ArrayList of Strings
* @return new Map
*/
public Map<Integer, String> intMap(ArrayList<String> str) {
throw new UnsupportedOperationException("Not supported yet");
}
/**
* Caiomhe has tons of Student Records that need to be organized. She wants you to put all these
* Records into a Map. The StudentRecord class already has a hashCode method that should be used
* to create keys. Store all the StudentRecord objects in a new map and then return it.
*
* @param students ArrayList of StudentRecord objects
* @return map
*/
public Map<Integer, StudentRecord> recordMap(ArrayList<StudentRecord> students) {
throw new UnsupportedOperationException("Not supported yet");
}
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

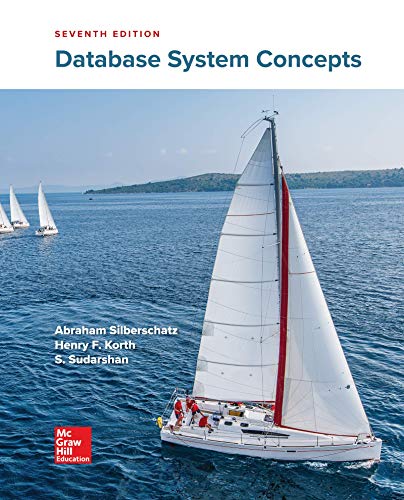
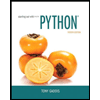
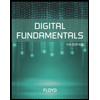
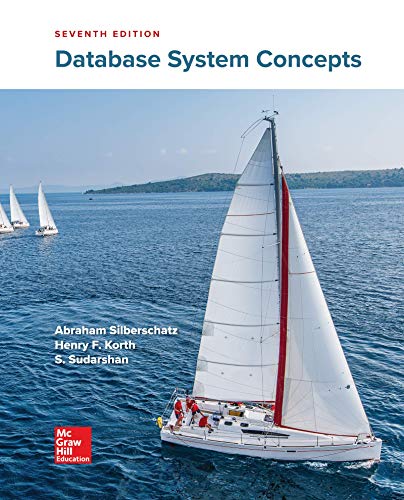
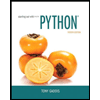
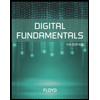
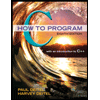
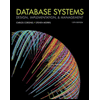
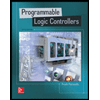