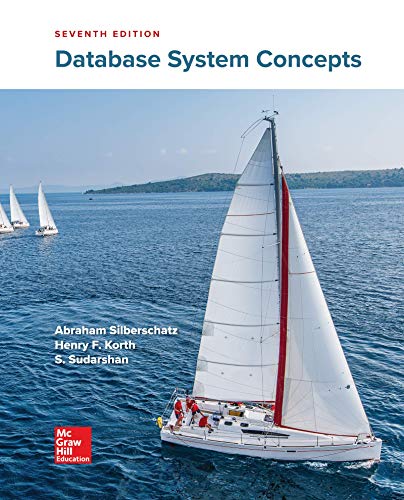
Concept explainers
C++
This program will store roster and rating information for a soccer team. Coaches rate players during tryouts to ensure a balanced team.
(1) Prompt the user to input five pairs of numbers: A player's jersey number (0 - 99) and the player's rating (1 - 9). Store the jersey numbers in one int vector and the ratings in another int vector. Output these
Ex:
Enter player 1's jersey number: 84 Enter player 1's rating: 7
Enter player 2's jersey number: 23 Enter player 2's rating: 4
Enter player 3's jersey number: 4 Enter player 3's rating: 5
Enter player 4's jersey number: 30 Enter player 4's rating: 2
Enter player 5's jersey number: 66 Enter player 5's rating: 9
ROSTER Player 1 -- Jersey number: 84, Rating: 7
Player 2 -- Jersey number: 23, Rating: 4 ...
(2) Implement a menu of options for a user to modify the roster. Each option is represented by a single character. Following the initial 5 players' input and roster output, the program outputs the menu. The program should also output the menu again after a user chooses an option. The program ends when the user chooses the option to Quit. For this step, the other options do nothing.
Ex:
MENU
a - Add player
d - Remove player
u - Update player rating
r - Output players above a rating
o - Output roster
q - Quit
Choose an option:
(3) Implement the "Output roster" menu option. Be sure to write a separate function for each menu option.
Ex:
ROSTER Player 1 -- Jersey number: 84, Rating: 7 Player 2 -- Jersey number: 23, Rating: 4 ...
(4) Implement the "Add player" menu option. Prompt the user for a new player's jersey number and rating. Append the values to the two vectors.
Ex:
Enter another player's jersey number: 49 Enter another player's rating: 8
(5) Implement the "Delete player" menu option. Prompt the user for a player's jersey number. Remove the player from the roster (delete the jersey number and rating). Be sure to keep the same relative order of all values in the vectors.
Ex:
Enter a jersey number: 4
(6) Implement the "Update player rating" menu option. Prompt the user for a player's jersey number. Prompt again for a new rating for the player, and then change that player's rating.
Ex:
Enter a jersey number: 23 Enter a new rating for player: 6
(7) Implement the "Output players above a rating" menu option. Prompt the user for a rating. Print the jersey number and rating for all players with ratings above the entered value.
Ex:
Enter a rating: 5 ABOVE 5 Player 1 -- Jersey number: 84, Rating: 7 ...
using functions :
void outputRoster(const vector<int> &jerseyNum, const vector<int> &rating)
void addPlayer(vector<int> &jerseyNum, vector<int> &rating)
void removePlayer(vector<int> &jerseyNum, vector<int> &rating)
void updatePlayerRating(const vector<int> &jerseyNum, vector<int> &rating)
void outputPlayersAboveRating(const vector<int> &, const vector<int> &);

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- C languagearrow_forwardCity Location (definition of simple cell array) The city of Istanbul, Turkey is located at a latitude of 41.0082 degrees north and longitude of 28.9784 degrees east. Write a script to do the following: 1. Define a character array variable named CityName and assign the value 'Istanbul, Turkey' to it. 2. Define a single precision two-element row vector named LatLong and assign the latitude of Istanbul to the first element and the longitude of Istanbul to the second element. 3. Define a 1 x 2 cell array named CityLocation. Assign the character array 'Istanbul, Turkey' to the first cell. Assign the single precision row vector of Istanbul's latitude and longitude to the second cell. Script> 1% defining character array variable 2 CityName = 'Istanbul, Turkey'; 3 4 % defining 2 element row vector 5 LatLong = [41.0082 28.9784]; 6 7% defining 1 x 2 cell array 8 CityLocation = {CityName; Lat Long}; 9 end Save My Solutions > C Reset MATLAB Documentation ?arrow_forwardPlease written by computer source (java)arrow_forward
- COMPUTER PROGRAMMING Data Validation Assignmentarrow_forwardGame of Hunt in C++ language Create the 'Game of Hunt'. The computer ‘hides’ the treasure at a random location in a 10x10 matrix. The user guesses the location by entering a row and column values. The game ends when the user locates the treasure or the treasure value is less than or equal to zero. Guesses in the wrong location will provide clues such as a compass direction or number of squares horizontally or vertically to the treasure. Using the random number generator, display one of the following in the board where the player made their guess: U# Treasure is up ‘#’ on the vertical axis (where # represents an integer number). D# Treasure is down ‘#’ on the vertical axis (where # represents an integer number) || Treasure is in this row, not up or down from the guess location. -> Treasure is to the right. <- Treasure is to the left. -- Treasure is in the same column, not left or right. +$ Adds $50 to treasure and no $50 turn loss. -$ Subtracts…arrow_forwardR4arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
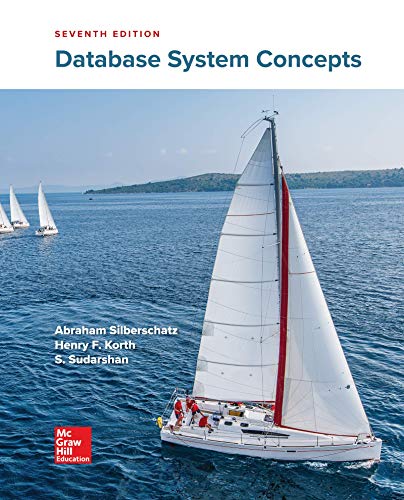
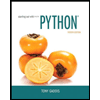
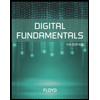
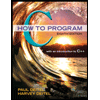
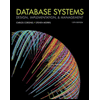
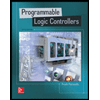