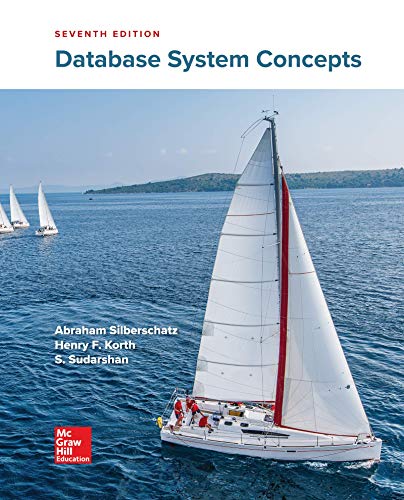
Using c++
Check if vector is sorted
Write the InOrder() function, which receives a vector of integers as a parameter, and returns true if the numbers are sorted (in order from low to high) or false otherwise. The
Ex: If the vector passed to the InOrder() function is [5, 6, 7, 8, 3], then the function returns false and the program outputs:
Not in orderEx: If the vector passed to the InOrder() function is [5, 6, 7, 8 , 10], then the function returns true and the program outputs:
In order#include <iostream>
#include <vector>
using namespace std;
bool InOrder(vector<int> nums) {
/* Type your code here */
}
int main() {
vector<int> nums1(5);
nums1.at(0) = 5;
nums1.at(1) = 6;
nums1.at(2) = 7;
nums1.at(3) = 8;
nums1.at(4) = 3;
if (InOrder(nums1)) {
cout << "In order" << endl;
}
else {
cout << "Not in order" << endl;
}
vector<int> nums2(5);
nums2.at(0) = 5;
nums2.at(1) = 6;
nums2.at(2) = 7;
nums2.at(3) = 8;
nums2.at(4) = 10;
if (InOrder(nums2)) {
cout << "In order" << endl;
}
else {
cout << "Not in order" << endl;
}
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- Please note global variables are not allowed to use in this assignment except of global constants. Find Median. In statistics, the median of a set of values is the value that lies in the middle when the values are arranged in sorted order. If the set has an even number of values, then the median is taken to be the average of the two middle values. Write a C++ program that determines the median of a sorted array. In the main function, Declare and initialize two arrays. One array must contain an even number of values, for example {91, 71, 67, 78, 76, 82, 100, 89}. The other array must contain an odd number of values, for example {76, 71, 78, 67, 82}. Call a function named sortArray to sort the values in each array in ascending order. should take an array of numbers and an integer indicating the size of the array as the arguments, and sort the array in ascending order. This function does not return any value. Call another function named findMedian to find the median of the values in…arrow_forwardJupyter Notebook Fixed Income - Certicificate of Deposit (CD) - Compound Interest Schedule An interest-at-maturity CD earns interest at a compounding frequency, and pays principal plus all earned interest at maturity. Write a function, called CompoundInterestSchedule, that creates and returns a pandas DataFrame, where each row has: time (in years, an integer starting at 1), starting balance, interest earned, and ending balance, for an investment earning compoundedinterest. Use a for(or while) loop to create this table. The equation for theith year's ending balance is given by: Ei =Bi (1+r/f)f where: Ei is year i's ending balance Bi is year i's beginning balance (note: B1 is the amount of the initial investment (principal) r is the annual rate of interest (in decimal, e.g., 5% is .05) f is the number of times the interest rate compounds (times per year) The interest earned for a given year is Ei - Bi Note the term of the investment (in years) is not in the above equation; it is used…arrow_forwardIn C++, define an integer vector and ask the user to give you values for the vector. Because you used a vector, so you don't need to know the size. user should be able to enter a number until he wants(you can use while loop for termination. for example if the user enters any negative number, but until he enters a positive number, your program should read and push it inside of the vector). the calculate the average of all numbers he entered.arrow_forward
- Question 5 Write the code for the function printRoster, it should print out the contacts in a format similar to the example. You can use a range-based for-loop and auto to simplify the solution, if you wish. #include #include #include #include #include using namespace std; void printRoster(map>>& roster) { // Fill code here int main() { map>> roster; roster["ELCO"]["CS-30"]. emplace_back("Anthony Davis"); roster["ElCo"]"cS-30"j. emplace_back("Talen Horton-Tucker"); roster["ElCo"Ï"BUS-101"].emplace_back("LeBron James"); roster["SMC"]["CHEM-101"].emplace_back("Russell Westbrook"); printRoster(roster); return 0; Here is the sample output: ElCo BUS-101: LeBron James CS-30: Anthony Davis Talen Horton-Tucker SMC CHEM-101: Russell Westbrookarrow_forwardAnswer in C++ code please! (Duplicate Elimination with vector) Use a vector to solve the following problem. Read in 20 numbers, each of which is between 10 and 100, inclusive. As each number is read, validate it and store it in the vector only if it isn't a duplicate of a number already read. After reading all the values, display only the unique values that the user entered. Begin with an empty vector and use its push_back function to add each unique value to the vector.arrow_forward(_9.). Given an array of integers, write a PHP function to find the maximum element in the array. PHP Function Signature: phpCopy code function findMaxElement($arr) { // Your code here } Example: Input: [10, 4, 56, 32, 7] Output: 56 You can now implement the findMaxElement function to solve this problem. Expect.arrow_forward
- c++arrow_forwardYou may insert an element into an arbitrary position inside a vector using an iterator. Write a function, insert(vector, value) which inserts value into its sorted position inside the vector. The function returns the vector after it is modified. #include <vector>using namespace std; vector<int>& insert(vector<int>& v, int value){ ................ return v; }arrow_forwardC++arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
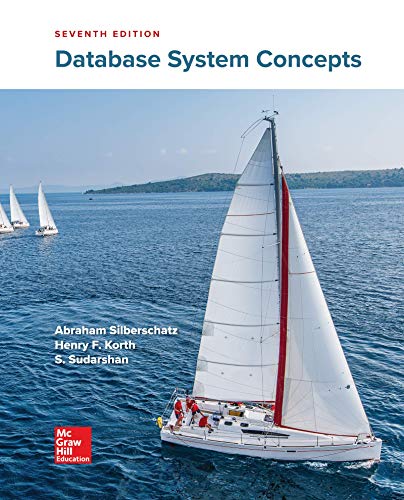
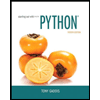
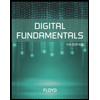
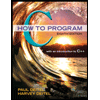
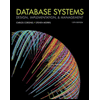
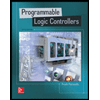