Translator Write a program that deals a number of cards (their numerical values) to a player's hand. To begin, the program asks the player for the values of the deck of cards to realize the program in c language. Then, the program deals the first two cards of the deck to a player's hand, and asks him if he wants another card. If he does not want a card, the program ends. If he wants a card, the program deals the next available card in the deck, adds it to the player's hand, and asks the player if he wants another card. The dealing process repeats as long as the user continues to accept cards, or until the cards in the deck run out. Card dealing involves copying the available card from the deck vector to another vector, which stores the player's hand, at the next available position. Assume that the deck of cards consists of only 10 cards with numerical values from 1 to 10, which the player will enter by keyboard before the program deals. The following functions must be implemented/used: enterDeckValues. - This function will receive two parameters: a vector that will store the numerical values of the deck of cards, and the number of cards in the deck. The function will fill the deck vector with the numerical values to be entered by keyboard. dealCards. - This function will receive three parameters: a vector that will store the numerical values of the deck of cards; the total number of cards in the deck; and, the vector that will store the numerical values of the player's final hand. The function will assign the first two cards of the deck to the player's hand and then deal cards to the player, assigning numerical values of the available cards in the deck, as explained in the approach. printVector - This function will receive two parameters: a vector and its dimension. The function will print the vector, and will serve to print the values (the vector) of the initial hand and the final hand. I attach screenshots of how it should be executed to realize the program in c language
Write a program that deals a number of cards (their numerical values) to a player's hand. To begin, the program asks the player for the values of the deck of cards to realize the program in c language.
Then, the program deals the first two cards of the deck to a player's hand, and asks him if he wants another card. If he does not want a card, the program ends. If he wants a card, the program deals the next available card in the deck, adds it to the player's hand, and asks the player if he wants another card. The dealing process repeats as long as the user continues to accept cards, or until the cards in the deck run out.
Card dealing involves copying the available card from the deck
Assume that the deck of cards consists of only 10 cards with numerical values from 1 to 10, which the player will enter by keyboard before the program deals.
The following functions must be implemented/used:
enterDeckValues. - This function will receive two parameters: a vector that will store the numerical values of the deck of cards, and the number of cards in the deck. The function will fill the deck vector with the numerical values to be entered by keyboard.
dealCards. - This function will receive three parameters: a vector that will store the numerical values of the deck of cards; the total number of cards in the deck; and, the vector that will store the numerical values of the player's final hand. The function will assign the first two cards of the deck to the player's hand and then deal cards to the player, assigning numerical values of the available cards in the deck, as explained in the approach.
printVector - This function will receive two parameters: a vector and its dimension. The function will print the vector, and will serve to print the values (the vector) of the initial hand and the final hand.
I attach screenshots of how it should be executed
to realize the program in c language


Step by step
Solved in 4 steps with 4 images

Using as a basis the program that allows to deal cards from a deck to a player's hand, add functionality that allows to update the deck of cards. Updating the deck of cards involves moving the cards from the deck to the beginning of the
The following functions must be implemented additionally:
updateDeck. - This function receives two arguments: a vector with the deck of cards to update and the current size of the deck of cards. It will move the cards in the deck to the beginning of the vector.
printVector - This function will receive two parameters: a vector and its dimension. The function will print the vector, and will print the values (the vector) of the initial hand, the updated deck, and the final hand.
to make the code in c language
Expected output :
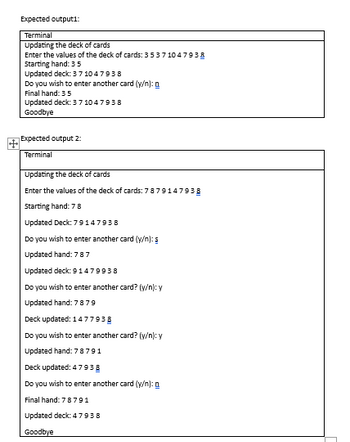
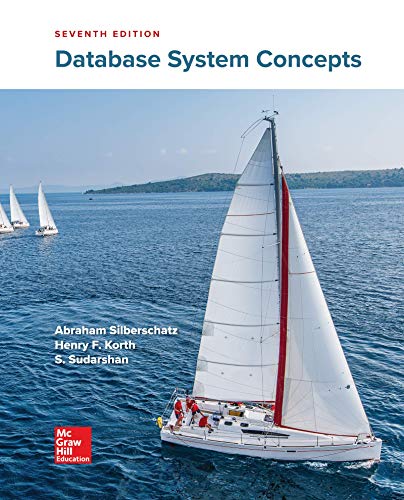
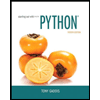
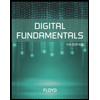
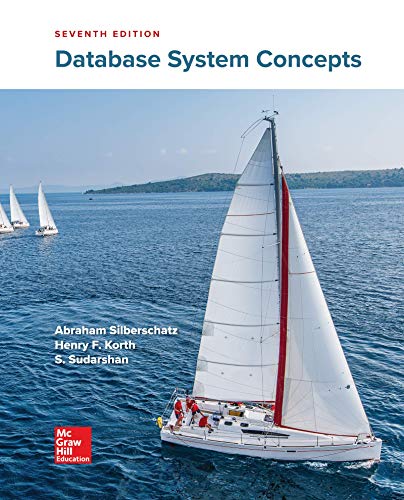
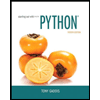
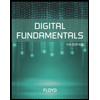
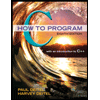
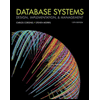
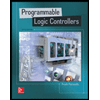