Programming Problem 1. Rock, Paper, Scissors This is your traditional rock-paper-scissors game, where two players select one of the three options and a result is produced based on their selection. For reference: Rock beats scissors Paper beats rock Scissors beats paper For this problem, you are to assume that two human players will play against each other and will input their choices accordingly. The input for each player will be in the form of buttons. Once a player clicks on a button, the rest of their buttons are locked in place (i.e. disabled). Once the other player selects their pick, the program outputs who won or if there was a draw. This isn’t exactly the most realistic way to code the game as it would be better if both players made their choice at the same time, but we’ll just have to chalk this up as a limitation for our exam. Aside from the game itself, the program should track the score of each player. Basically, if one wins, that player’s score goes up by one. When a draw occurs, neither players’ score increments.
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Computer Science
Godot Programming
Programming Problem 1. Rock, Paper, Scissors This is your traditional rock-paper-scissors game, where two players select one of the three options and a result is produced based on their selection. For reference: Rock beats scissors Paper beats rock Scissors beats paper For this problem, you are to assume that two human players will play against each other and will input their choices accordingly. The input for each player will be in the form of buttons. Once a player clicks on a button, the rest of their buttons are locked in place (i.e. disabled). Once the other player selects their pick, the program outputs who won or if there was a draw. This isn’t exactly the most realistic way to code the game as it would be better if both players made their choice at the same time, but we’ll just have to chalk this up as a limitation for our exam. Aside from the game itself, the program should track the score of each player. Basically, if one wins, that player’s score goes up by one. When a draw occurs, neither players’ score increments.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

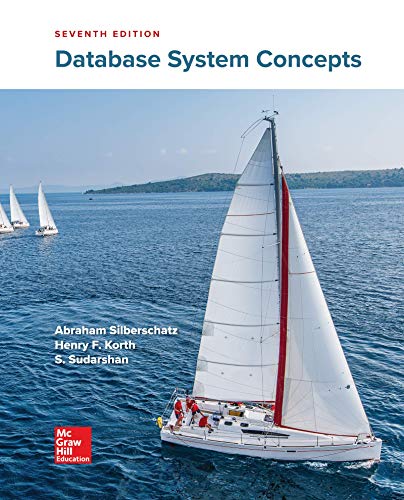
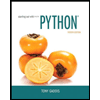
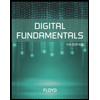
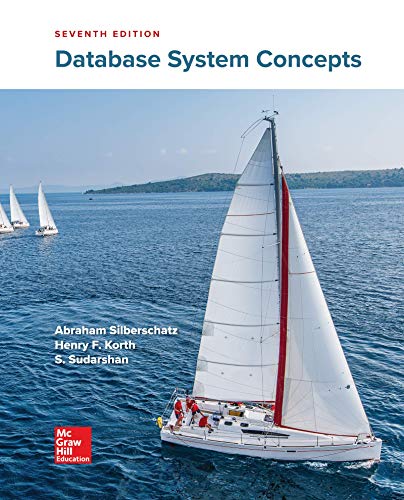
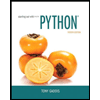
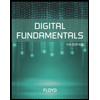
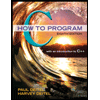
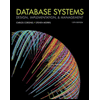
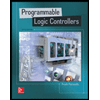