C ++ Program Exception -1 in int at, int operator [] (int x). In void reserve : (size is smaller than current size) functions I tried to put exception in void reserve but I need to put in the int main () using:try, catch and throw, when this print the message of the three functions where I should put exception . Help please #include #include #include using namespace std; class Vec { private: int *arr; int sz = 0; int cap = 0; public: Vec() { arr = new int[1]; this->cap = 1; this->sz = 0; } Vec(int *array) { int n = sizeof(array) / sizeof(array[0]) + 1; arr = new int[n]; for (int i = 0; i < n; i++) arr[i] = array[i]; sz = n; cap = n; } int size() { return this->sz; } int capacity() { return this->cap; } void reserve(int n) { if (n < sz) throw "Size is smaller than curent size"; if (n > sz) { int *newarr = new int[n]; int i; for (i = 0; i < sz; i++) { newarr[i] = this->arr[i]; } int *oldarr = this->arr; this->arr = newarr; delete[] oldarr; cap = n; arr = newarr; } } void push_back(int v) { if (sz == cap) { int *t = new int[cap + 1]; for (int i = 0; i < cap; i++) { t[i] = arr[i]; } cap *= 2; reserve(cap); } this->arr[sz] = v; sz++; } void print() { for (int i = 0; i < sz; i++) { cout << arr[i] << " "; } cout << endl; } int at(int idx) { return this->arr[idx]; } int findHighestIterative() { int highest = arr[0]; for (int i = 1; i < sz; i++) { if (arr[i] > highest) { highest = arr[i]; } } return highest; } int helperRecursive(int *arr, int n) { if (n == 1) return arr[0]; return max(arr[n - 1], helperRecursive(arr, n - 1)); } int findHighestRecursive() { return helperRecursive(arr, sz); } friend Vec operator+(Vec &, Vec &); friend Vec operator*(Vec &, int s); friend Vec operator*(int s, Vec &); friend int operator*(Vec &, Vec &); int operator[](int x); friend ostream &operator<<(ostream &out, const Vec &op2); }; Vec operator+(Vec &a, Vec &b) { Vec c; for (int i = 0; i < a.sz; i++) c.push_back(a.arr[i] + b.arr[i]); return c; } Vec operator*(Vec &a, int s) { Vec c; for (int i = 0; i < a.sz; i++) c.push_back(a.arr[i] * s); return c; } Vec operator*(int d, Vec &a) { Vec c; for (int i = 0; i < a.sz; i++) c.push_back(d * a.arr[i]); return c; } int operator*(Vec &a, Vec &b) { int y = 0; for (int i = 0; i < a.sz; i++) y += (a.arr[i] * b.arr[i]); return y; } int Vec::operator[](int x) { if (x < sz) return arr[x]; else return 0; } ostream &operator<<(ostream &out, const Vec &ope2) { int n = ope2.sz; out << "["; for (int i = 0; i < n; i++) out << ope2.arr[i] << ", "; out << "\b\b]"; return out; } int main() { Vec v; v.reserve(10); v.push_back(3); v.push_back(2); cout << "v.size = " << v.size() << endl; // 2 cout << "v.capacity = " << v.capacity() << endl; // 10 v.push_back(3); v.push_back(2); v.push_back(3); v.push_back(4); v.push_back(34); v.push_back(7); v.push_back(3); v.push_back(8); v.push_back(2); cout << v << endl; cout << "v.size = " << v.size() << endl; // 11 cout << "v.capacity = " << v.capacity() << endl; // 20 cout << "Highest iterative = " << v.findHighestIterative() << endl; cout << "highest Recursive = " << v.findHighestRecursive() << endl; v.newLoad("newLoad.txt"); v.newSave("newSave.txt"); cout << "after loading from file in v" << endl; cout << v << endl; cout << "Printing using at function" << endl; for (int i = 0; i < v.size(); i++) { cout << v.at(i) << endl; } cout << endl; int arr1[] = {3, 7, 8}; Vec obj1(arr1); int arr2[] = {5, 9, 6}; Vec obj2(arr2); int arr4[] = {1, 4, 2}; Vec obj3(arr4); Vec obj4, obj5; obj3 = obj1 + obj2; cout << obj3 << endl; obj4 = obj1 * 4; cout << obj4 << endl; int a = obj1 * obj2; cout << a << endl; int b = obj2[1]; cout << b << endl; return 0; }
C ++ Program Exception -1 in int at, int operator [] (int x). In void reserve : (size is smaller than current size) functions I tried to put exception in void reserve but I need to put in the int main () using:try, catch and throw, when this print the message of the three functions where I should put exception . Help please
#include <fstream>
#include <iostream>
#include <string>
using namespace std;
class Vec {
private:
int *arr;
int sz = 0;
int cap = 0;
public:
Vec() {
arr = new int[1];
this->cap = 1;
this->sz = 0;
}
Vec(int *array) {
int n = sizeof(array) / sizeof(array[0]) + 1;
arr = new int[n];
for (int i = 0; i < n; i++)
arr[i] = array[i];
sz = n;
cap = n;
}
int size() {
return this->sz;
}
int capacity() {
return this->cap;
}
void reserve(int n) {
if (n < sz)
throw "Size is smaller than curent size";
if (n > sz) {
int *newarr = new int[n];
int i;
for (i = 0; i < sz; i++) {
newarr[i] = this->arr[i];
}
int *oldarr = this->arr;
this->arr = newarr;
delete[] oldarr;
cap = n;
arr = newarr;
}
}
void push_back(int v) {
if (sz == cap) {
int *t = new int[cap + 1];
for (int i = 0; i < cap; i++) {
t[i] = arr[i];
}
cap *= 2;
reserve(cap);
}
this->arr[sz] = v;
sz++;
}
void print() {
for (int i = 0; i < sz; i++) {
cout << arr[i] << " ";
}
cout << endl;
}
int at(int idx) {
return this->arr[idx];
}
int findHighestIterative() {
int highest = arr[0];
for (int i = 1; i < sz; i++) {
if (arr[i] > highest) {
highest = arr[i];
}
}
return highest;
}
int helperRecursive(int *arr, int n) {
if (n == 1)
return arr[0];
return max(arr[n - 1], helperRecursive(arr, n - 1));
}
int findHighestRecursive() {
return helperRecursive(arr, sz);
}
friend Vec operator+(Vec &, Vec &);
friend Vec operator*(Vec &, int s);
friend Vec operator*(int s, Vec &);
friend int operator*(Vec &, Vec &);
int operator[](int x);
friend ostream &operator<<(ostream &out, const Vec &op2);
};
Vec operator+(Vec &a, Vec &b) {
Vec c;
for (int i = 0; i < a.sz; i++)
c.push_back(a.arr[i] + b.arr[i]);
return c;
}
Vec operator*(Vec &a, int s) {
Vec c;
for (int i = 0; i < a.sz; i++)
c.push_back(a.arr[i] * s);
return c;
}
Vec operator*(int d, Vec &a) {
Vec c;
for (int i = 0; i < a.sz; i++)
c.push_back(d * a.arr[i]);
return c;
}
int operator*(Vec &a, Vec &b) {
int y = 0;
for (int i = 0; i < a.sz; i++)
y += (a.arr[i] * b.arr[i]);
return y;
}
int Vec::operator[](int x) {
if (x < sz)
return arr[x];
else
return 0;
}
ostream &operator<<(ostream &out, const Vec &ope2) {
int n = ope2.sz;
out << "[";
for (int i = 0; i < n; i++)
out << ope2.arr[i] << ", ";
out << "\b\b]";
return out;
}
int main() {
Vec v;
v.reserve(10);
v.push_back(3);
v.push_back(2);
cout << "v.size = " << v.size() << endl; // 2
cout << "v.capacity = " << v.capacity() << endl; // 10
v.push_back(3);
v.push_back(2);
v.push_back(3);
v.push_back(4);
v.push_back(34);
v.push_back(7);
v.push_back(3);
v.push_back(8);
v.push_back(2);
cout << v << endl;
cout << "v.size = " << v.size() << endl; // 11
cout << "v.capacity = " << v.capacity() << endl; // 20
cout << "Highest iterative = " << v.findHighestIterative() << endl;
cout << "highest Recursive = " << v.findHighestRecursive() << endl;
v.newLoad("newLoad.txt");
v.newSave("newSave.txt");
cout << "after loading from file in v" << endl;
cout << v << endl;
cout << "Printing using at function" << endl;
for (int i = 0; i < v.size(); i++) {
cout << v.at(i) << endl;
}
cout << endl;
int arr1[] = {3, 7, 8};
Vec obj1(arr1);
int arr2[] = {5, 9, 6};
Vec obj2(arr2);
int arr4[] = {1, 4, 2};
Vec obj3(arr4);
Vec obj4, obj5;
obj3 = obj1 + obj2;
cout << obj3 << endl;
obj4 = obj1 * 4;
cout << obj4 << endl;
int a = obj1 * obj2;
cout << a << endl;
int b = obj2[1];
cout << b << endl;
return 0;
}

Step by step
Solved in 3 steps with 8 images

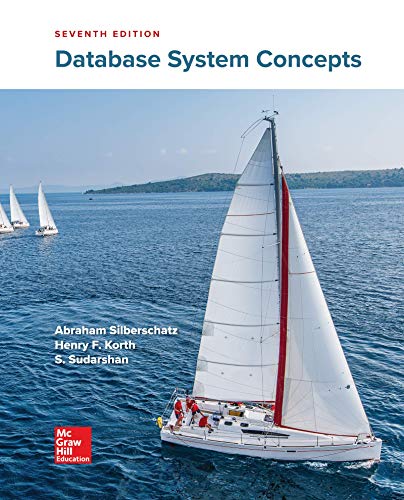
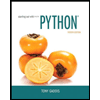
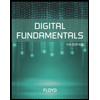
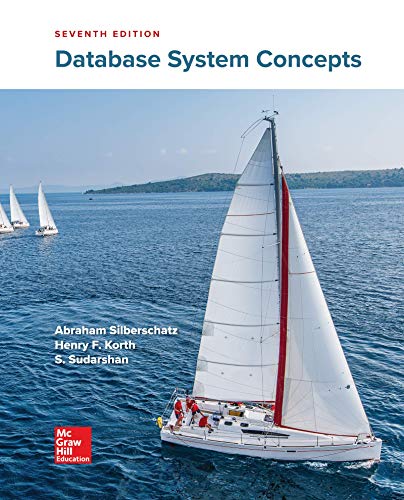
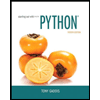
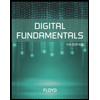
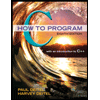
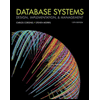
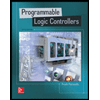