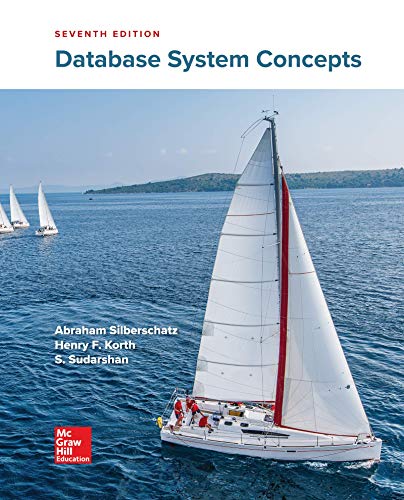
Concept explainers
C++ part 4: I have these error i am missing the ExecuteMenu Function
This is my code so far.
#include <iostream>
#include <string>
using namespace std;
int GetNumOfNonWSCharacters(const string text);
int GetNumOfWords(const string text);
int FindText(string text, string sample_text);
string ReplaceExclamation(string text);
string ShortenSpace(string text);
char PrintMenu(string sample_text){
char option;
cout << "\nMENU"<<endl;
cout << "c - Number of non-whitespace characters"<<endl;
cout << "w - Number of words"<<endl;
cout << "f - Find text"<<endl;
cout << "r - Replace all !'s"<<endl;
cout << "s - Shorten spaces"<<endl;
cout << "q - Quit"<<endl;
cout<<endl;
cout << "Choose an option:"<<endl;
cin >> option;
if(option == 'c'){
int nonWSCharacters = GetNumOfNonWSCharacters(sample_text);
cout << "Number of non-whitespace characters: "<<nonWSCharacters<<endl;
}else if(option == 'w'){
int numOfWords = GetNumOfWords(sample_text);
cout << "Number of words: "<<numOfWords<<endl;
}else if(option == 'f'){
cout<<"Enter a word or phrase to be found:"<<endl;
string phrase;
cin.ignore();
getline(cin, phrase, '\n');
int instances = FindText(phrase,sample_text);
cout << "\""<< phrase << "\" instances: "<<instances<<endl;
}else if(option == 'r'){
string edited_text = ReplaceExclamation(sample_text);
cout << "Edited text: "<<edited_text<<endl;
}else if(option == 's'){
string edited_text = ShortenSpace(sample_text);
cout << "Edited text: "<<edited_text<< endl;
}
return option;
}
int GetNumOfNonWSCharacters(const string text){
int len = text.length(), nonWSCharacters = 0;
for(int i = 0; i < len; i++){
if(text[i] != ' ' && text[i] != '\n' && text[i] != '\t')
nonWSCharacters++;
}
return nonWSCharacters;
}
int GetNumOfWords(const string text){
int len = text.length(), numOfWords = 0;
for(int i = 0; i < len; i++){
if(text[i] == ' ' || text[i] == '\n' || text[i] == '\t'){
numOfWords++;
++i;
while(i < len && (text[i] == ' ' || text[i] == '\n' || text[i] == '\t'))
++i;
--i;
}
}
if(text[len-1] != ' ' || text[len-1] != '\n' || text[len-1] != '\t')
numOfWords++;
return numOfWords;
}
int FindText(string text, string sample_text){
int sample_length = sample_text.length();
int text_length = text.length();
int instances = 0;
for(int i=0;i<=sample_length-text_length; i++){
int j = 0;
for(; j<text_length; j++){
if(text[j]!=sample_text[i+j])
break;
}
if(j==text_length){
instances++;
}
}
return instances;
}
string ReplaceExclamation(string sample_text){
int len = sample_text.length();
int i = 0;
string edited_text = "";
while(i < len)
{
if(sample_text[i] == '!'){
edited_text += '.';
}else{
edited_text += sample_text[i];
}
i++;
}
return edited_text;
} string ShortenSpace(string sample_text){
int len = sample_text.length();
int i = 0;
string edited_text = "";
while(i < len-1)
{
if(sample_text[i] == ' ' && sample_text[i+1] == ' ')
{
i++;
continue;
}else{
edited_text += sample_text[i];
}
i++;
}
if(sample_text[len-1] != ' '){
edited_text += sample_text[len-1];
}
return edited_text;
}
int main(){
string sample_text;
cout << "Enter a sample text:"<<endl;
getline(cin, sample_text);
cout<<endl;
cout << "You entered: ";
cout<<sample_text<<endl;
while(true){
char option = PrintMenu(sample_text);
if(option=='q')
break;
}
return 0;
}
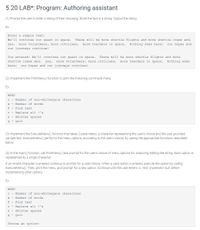
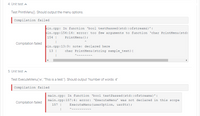

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 5 images

- #include <iostream>#include <string.h>using namespace std;int index1(char *T,char *P){int t=0,p=0,i,j,r;t = strlen(T);p = strlen(P);i=0;int max = t - p + 1;while(i<max){for(j=0;j<p;j++){if(P[j]==T[i+j]){r = i;break;}else r = -1;}i++;}return r;} int main(){ int l;char c[100];char d[100];cout<<"Enter First String\n"; cin>>c;cout<<"Enter Second String\n";cin>>d;l = index1(c,d);cout<<l; return 0;} Note: Remove Funcationarrow_forwardC++ Please Help!arrow_forwardIn C++Using the code provided belowDo the Following: Modify the Insert Tool Function to ask the user if they want to expand the tool holder to accommodate additional tools Add the code to the insert tool function to increase the capacity of the toolbox (Dynamic Array) USE THE FOLLOWING CODE and MODIFY IT: #define _SECURE_SCL_DEPRECATE 0 #include <iostream> #include <string> #include <cstdlib> using namespace std; class GChar { public: static const int DEFAULT_CAPACITY = 5; //constructor GChar(string name = "john", int capacity = DEFAULT_CAPACITY); //copy constructor GChar(const GChar& source); //Overload Assignment GChar& operator=(const GChar& source); //Destructor ~GChar(); //Insert a New Tool void insert(const std::string& toolName); private: //data members string name; int capacity; int used; string* toolHolder; }; //constructor GChar::GChar(string n, int cap) { name = n; capacity = cap; used = 0; toolHolder = new…arrow_forward
- >> IN C PROGRAMMING LANGUAGE ONLY << COPY OF DEFAULT CODE, ADD SOLUTION INTO CODE IN C #include <stdio.h>#include <stdlib.h>#include <string.h> #include "GVDie.h" int RollSpecificNumber(GVDie die, int num, int goal) {/* Type your code here. */} int main() {GVDie die = InitGVDie(); // Create a GVDie variabledie = SetSeed(15, die); // Set the GVDie variable with seed value 15int num;int goal;int rolls; scanf("%d", &num);scanf("%d", &goal);rolls = RollSpecificNumber(die, num, goal); // Should return the number of rolls to reach total.printf("It took %d rolls to get a \"%d\" %d times.\n", rolls, num, goal); return 0;}arrow_forwardProblem DNA: Subsequence markingA common task in dealing with DNA sequences is searching for particular substrings within longer DNA sequences. Write a function mark_dna that takes as parameters a DNA sequence to search, and a shorter target sequence to find within the longer sequence. Have this function return a new altered sequence that is the original sequence with all non-overlapping occurrences of the target surrounded with the characters >> and <<. Hints: ● String slicing is useful for looking at multiple characters at once. ● Remember that you cannot modify the original string directly, you’ll need to build a copy. Start with an empty string and concatenate onto it as you loop. Constraints: ● Don't use the built-in replace string method. All other string methods are permitted. >>> mark_dna('atgcgctagcatg', 'gcg') 'at>>gcg<<ctagcatg' >>> mark_dna('atgcgctagcatg', 'gc')…arrow_forwardhere is a and b part plz solve c part #include <iostream>#include <string.h>#include <stdlib.h>#include <sstream>#include <cmath>using namespace std; int main(){ /*Variable declarations*/int higestDegree,higestDegree1;int diffDegree,smallDegree;std::stringstream polynomial,addpolynomial;/*prompt for degree*/std::cout<<"Please enter higest degree of polynomial"<<std::endl;std::cin>>higestDegree; std::cout<<"Please Enter highest degree of 2nd polynomial"<<std::endl;std::cin>>higestDegree1;int *cofficient=new int[higestDegree+1];//cofficient array creation for 1st polynomailint *cofficient1=new int[higestDegree1+1];//cofficient array creation for 2st polynomail /*taking cofficient for 1st polynomial*/std::cout<<"Please enter cofficient of each term from higest degree to lowest for 1st polynomial"<<std::endl;for(int i=0;i<=higestDegree;i++){std::cin>>cofficient[i];}/*taking cofficient for 2st…arrow_forward
- please use DEQUE #include <iostream>#include <string>#include <deque> using namespace std; const int AIRPORT_COUNT = 12;string airports[AIRPORT_COUNT] = {"DAL","ABQ","DEN","MSY","HOU","SAT","CRP","MID","OKC","OMA","MDW","TUL"}; int main(){// define stack (or queue ) herestring origin;string dest;string citypair;cout << "Loading the CONTAINER ..." << endl;// LOAD THE STACK ( or queue) HERE// Create all the possible Airport combinations that could exist from the list provided.// i.e DALABQ, DALDEN, ...., ABQDAL, ABQDEN ...// DO NOT Load SameSame - DALDAL, ABQABQ, etc .. cout << "Getting data from the CONTAINER ..." << endl;// Retrieve data from the STACK/QUEUE here } Using the attached shell program (AirportCombos.cpp), create a list of strings to process and place on a STL DEQUE container. Using the provided 3 char airport codes, create a 6 character string that is the origin & destination city pair. Create all the possible…arrow_forwardC++ Program: #include <iostream>#include <string> using namespace std; const int AIRPORT_COUNT = 12;string airports[AIRPORT_COUNT] = {"DAL","ABQ","DEN","MSY","HOU","SAT","CRP","MID","OKC","OMA","MDW","LAX"}; int main(){ // define stack (or queue ) here string origin; string dest; string citypair; cout << "Loading the CONTAINER ..." << endl; // LOAD THE STACK ( or queue) HERE // Create all the possible Airport combinations that could exist from the list provided. // i.e DALABQ, DALDEN, ...., ABQDAL, ABQDEN ... // DO NOT Load SameSame - DALDAL, ABQABQ, etc .. cout << "Getting data from the CONTAINER ..." << endl;// Retrieve data from the STACK/QUEUE here } Using the attached program (AirportCombos.cpp), create a list of strings to process and place on a STL STACK container. The provided code is meant to be generic. Using the provided 3 char airport codes, create a 6 character string that is the origin &…arrow_forwardIn C++ Assume that a 5 element array of type string named boroughs has been declared and initialized. Write the code necessary to switch (exchange) the values of the first and last elements of the array.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
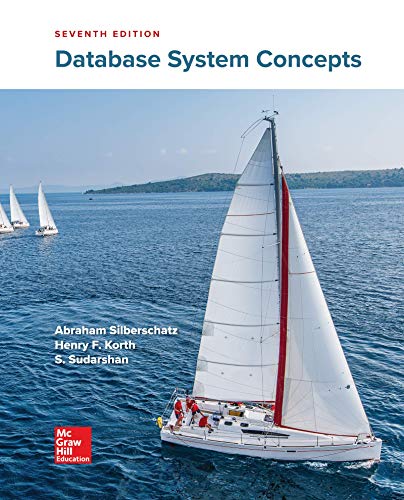
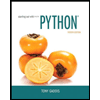
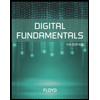
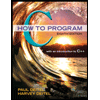
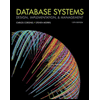
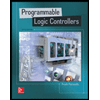