Bubble Sort - Selection Sort - Insertion Sort - Shell Sort - Merge Sort - Quick Sort - Heap sort You can get source codes of sorting programs from lectures notes. By using the files that you generated in Question I, read each file and sort file by using each of sorting algorithms. Record the execution time of each sorting algorithm into a file named “sortStats.txt”. The “sortStats.txt” file should contain entries for execution times as follows:
Perform a benchmark analysis of the following sorting algorithms:
- Bubble Sort
- Selection Sort
- Insertion Sort
- Shell Sort
- Merge Sort
- Quick Sort
- Heap sort
You can get source codes of sorting programs from lectures notes. By using the files that you generated in Question I, read each file and sort file by using each of sorting algorithms. Record the execution time of each sorting
Bubble_Sort n1, n2, n3, n4, n5, n6, n7
Selection_Sort n1, n2, n3, n4, n5, n6, n7
Insertion_Sort n1, n2, n3, n4, n5, n6, n7
Shell_Sort n1, n2, n3, n4, n5, n6, n7
Merge_Sort n1, n2, n3, n4, n5, n6, n7
Quick_Sort n1, n2, n3, n4, n5, n6, n7
Heap_Sort n1, n2, n3, n4, n5, n6, n7
where n1, n2, n3, n4, n5, n6 and n7 are execution times for file sizes 1000, 5000, 10000, 25000, 50000, 100000, 20000.
Submit your program code and sortStats.txt in ZIP file together with the solutions of other programs

Step by step
Solved in 2 steps

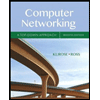
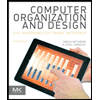
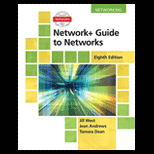
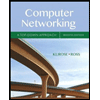
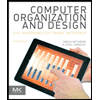
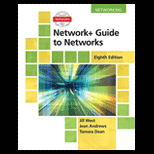
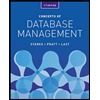
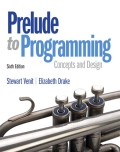
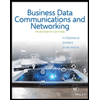