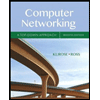
Brussel's choice
def brussels_choice_step(n, mink, maxk):
This problem is adapted from another jovial video "The Brussel's Choice" of Numberphile (a site so British that you just know there has to be a Trevor and a Colin somewhere in there) whose first five minutes you should watch to get an idea of what is going on. This function should compute and
return the list of all numbers that the positive integer n can be converted to by treating it as a string and replacing some substring m of its digits with the new substring of either 2*m or m/2, the latter
substitution allowed only when m is even so that dividing it by two produces an integer.
This function should return the list of numbers that can be produced from n in a single step. To keep the results more manageable, we also impose an additional constraint that the number of digits in the chosen substring m must be between mink and maxk, inclusive. The returned list must contain the numbers in ascending sorted order.
![mink maxk Expected result
42
1
2
[21, 22, 41, 44, 82, 84]
1234
1
1
[1134, 1232, 1238, 1264, 1434, 2234]
1234
[634, 1117, 1217, 1268, 1464, 1468,
2434, 2464]
88224
2
3
[44124, 44224, 84114, 84124, 88112,
88114, 88212, 88248, 88444, 88448,
176224, 176424, 816424,816444]
123456789 |7
[61728399, 111728399, 126913578,
146913569, 146913578, 246913489,
246913569, 246913578]
(a list with 65 elements, the last three of which are
[1234567169, 1234567178, 1234567818 ])
123456789 |1](https://content.bartleby.com/qna-images/question/b9ec6203-7441-453e-8993-3e6380986f00/bdb2f9a3-a59e-432f-9587-482a0a8e1f52/d2l2lue_thumbnail.png)

Step by stepSolved in 2 steps with 3 images

- In pythonarrow_forwardPlease help. This is Python I feel so lostarrow_forwardThe function list_combination takes two lists as arguments, list_one and list_two. Return from the function the combination of both lists - that is, a single list such that it alternately takes elements from both lists starting from list_one. You can assume that both list_one and list_two will be of equal lengths. As an example if list_one = [1,3,5] and if list_two = [2,4,6]. The function must return [1,2,3,4,5,6].arrow_forward
- program- python Write a function called “missing”. Ask the user for a series of integers from 1 to N. Send that list to missing and have it return the list of what numbers are missing. For example, if they enter 1 3 7 10, then you would call missing on that list which returns the list of missing numbers and then you can let them know by printing out the list that they are missing 2 4 5 6 8 9. Test it out on this and show it works. PLEASE USE SET SUBTRACTIONarrow_forwardCreate a function that determines whether each seat can "see" the front-stage. A number can "see" the front-stage if it is strictly greater than the number before it. Everyone can see the front-stage in the example below: # FRONT STAGE [[1, 2, 3, 2, 1, 1], [2, 4, 4, 3, 2, 2], [5, 5, 5, 5, 4, 4], [6, 6, 7, 6, 5, 5]] # Starting from the left, the 6 > 5 > 2 > 1, so all numbe # 6 > 5 > 4 > 2 - so all numbers can see, etc. Not everyone can see the front-stage in the example below: # FRONT STAGE [[1, 2, 3, 2, 1, 1], [2, 4, 4, 3, 2, 2], [5, 5, 5, 10, 4, 4], [6, 6, 7, 6, 5, 5]] # The 10 is directly in front of the 6 and blocking its varrow_forwardCan you use Python programming language to to this question? Thanksarrow_forward
- Complete the rotate_text() function that takes 2 parameters, a string data and an integer n. If n is positive, then the function will shift all the characters in data forward by n positions, with characters at the end of the string being moved to the start of the string. If n is 0 then the text remains the same. For example: rotate_text('abcde', rotate_text('abcde', rotate_text('abcde', 1) would return the string 'eabcd' 3) would return the string 'cdeab' 5) would return the string 'abcde' rotate_text('abcde', 6) would return the string 'eabcd' ... and so on. If n is negative, then the function will shift the characters in data backward by n positions, with characters at the start of the string being moved to the end of the string. For example: rotate text('abcde', -1) would return the string 'bcdea'arrow_forwardPYTHON Complete the function below, which takes two arguments: data: a list of tweets search_words: a list of search phrases The function should, for each tweet in data, check whether that tweet uses any of the words in the list search_words. If it does, we keep the tweet. If it does not, we ignore the tweet. data = ['ZOOM earnings for Q1 are up 5%', 'Subscriptions at ZOOM have risen to all-time highs, boosting sales', "Got a new Mazda, ZOOM ZOOM Y'ALL!", 'I hate getting up at 8am FOR A STUPID ZOOM MEETING', 'ZOOM execs hint at a decline in earnings following a capital expansion program'] Hint: Consider the example_function below. It takes a list of numbers in numbers and keeps only those that appear in search_numbers. def example_function(numbers, search_numbers): keep = [] for number in numbers: if number in search_numbers(): keep.append(number) return keep def search_words(data, search_words):arrow_forwardIn this problem, you have available to you a working version of the function round_up described in part (a). It is located in a module named foo. Using the given round_up function, write a function round_up_all that takes a list of integers and MODIFIES the list so that each number is replaced by the result of calling round_up_ on that number. For example, if you run the code, 1st = [43, 137, 99, 501, 300, 275] round_up_all(1st) print(1st) the list would contain the values [100, 200, 100, 600, 300, 300] Do not re-implement the round_up function, just import the food module and use it. Here is the code for the round_up function that can be imported using foo: def round_up(num): if num % 100 == 0 : #if number is complete divisible return num #return orginal num else: return num + (100-(num % 100)) #else add 100-remainder to num, if __name__ == '__main__': print(round_up(234)) print(round_up(465)) print(round_up(400)) print(round_up(89))arrow_forward
- Instructions There are four clubs in Tesset University South, North, West, and East. A Tesset student can choose to join any number of clubs. Your task is to determine how many students are enrolled in exactly two clubs. Write a function/method named dualClubCounter that will accept a string containing the student number and his desired club. Return a set containing the name of students are enrolled exactly in two clubs. Use SET in your solution Example #1 INPUT: "12300:East,12301:West, 12302:South, 12300:West, 123 Expected Content of Set = [12300] Example #2 INPUT: "12300:East,12301:West, 12302:South, 12300:West,123 Expected Content of Set = [12300, 12301]arrow_forwardcode in python The function print_reverse takes one parameter, L, which is a list. The function then prints the items in the list in reverse order, one item per line, WITHOUT changing the value of L. Write the function definition. Note 1: This function does NOT get anything from standard input. Note 2: This function DOES, however, print to standard output. Note 3: You should NOT return anything from this function (let it return None by default). Hint: Some ideas for iterating over a list in reverse order are given here. For example: Test Result L = ['apple', 'banana', 'cherry'] Lcopy = L[:] return_value = print_reverse(L) if return_value != None or L != Lcopy: print("Error") cherry banana apple L = ['happy'] Lcopy = L[:] return_value = print_reverse(L) if return_value != None or L != Lcopy: print("Error") happy L = [] Lcopy = L[:] return_value = print_reverse(L) if return_value != None or L != Lcopy: print("Error")arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
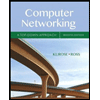
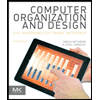
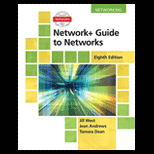
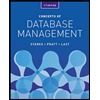
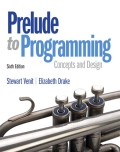
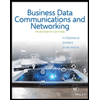