MiniMac MiniMac is a virtual processor with a tiny but extendable memory and a tiny but extendable instruction set. Here’s a screenshot of the MiniMac user interface: A screenshot of a computer Description automatically generated The view panel on the right contains two lists (javax.swing.JList). The top list shows the contents of memory. The bottom list shows the program currently being executed. The control panel on the left contains three buttons (javax.swing.JButton). The first button prompts the user for a program to parse: A screenshot of a computer program Description automatically generated The second button executes the program. The third button resets all memory cells to 0. These commands are duplicated under the Edit menu. The Help menu explains each command. The File menu contains the items: New, Save, Open, and Quit. The MiniMax memory is an array of integers: int size = 32; Integer[] memory = new Integer[size]; A MiniMax program is a list of instructions. An instruction pointer (ip) indicates the position in the list of the next instruction to be executed. Grammar Here’s the instruction set grammar (note: “~” means “followed by” and all unquoted tokens are integers): load ::= “load” ~ location ~ value // memory[location] = value halt ::= “halt” // terminates the program add ::= “add” ~ src1 ~ src2 ~ dest // memory[dest] = memory[src1] + memory[src2] mul ::= “mul” ~ src1 ~ src2 ~ dest // memory[dest] = memory[src1] * memory[src2] bgt ::= “bgt” ~ location ~ offset // if 0 < memory[location] ip += offset blt ::= “blt” ~ location ~ offset // if memory[location] < 0 ip += offset loop ::= “loop ~ count ~ instruction // executes instruction count times A block is a list of one or more instructions separated by semicolons and bracketed by curly braces: block ::= “{“ ~ instruction ~ (“;” ~ instruction)* ~ “}” Executing a block sequentially executes each instruction in the block’s body. For example, the program shown in the screenshot computes kn, where n is stored in memory[0]. The base, k, is stored in memory[2]. In this case k = 2, n was 7 and the result, 128, is stored in memory[1]. Memory[3] is the amount to decrement memory[0] each time the result is multiplied by k. Design Here’s the design of MiniMac: A diagram of a program Description automatically generated Parser Here’s a partial implementation of MiniMacParser.java. Note that it is a utility class (aka singleton), all of its methods are static. Testing Implement and test the following functions in MiniMac: Tri(n) = 1 + 2 + ... + n // stored in a file called tri Fib(n) = nth Fibonacci number // stored in a file called fib Less(n, m) = (n < m)?1:0 // 1 = true and 0 = false Log(n) = m where 2m <= n and n < 2m+1 In each case the output, f(n), should be stored in memory[0] and the inputs should be stored beginning in memory[1]. Hints 1. The MiniMac is a publisher and the view panel is its subscriber. Each time memory is updated or a new program is set, MiniMac notifies its subscribers. 2. The control panel is the action listener for its buttons. It navigates to the MiniMac and calls the appropriate procedure (execute and clear). 3. Parsing is more complicated. The control panel must prompt the user for a file name, read the file (as a string), then pass the string to MiniMaxParser.parse(program). It then sets the MiniMac program to the parser’s output, which causes a subscriber notification. Suggestion: ask ChatGPT how to read a text file in Java. 4. Ask ChatGPT for a simple example of how to use a JList.
MiniMac MiniMac is a virtual processor with a tiny but extendable memory and a tiny but extendable instruction set. Here’s a screenshot of the MiniMac user interface: A screenshot of a computer Description automatically generated The view panel on the right contains two lists (javax.swing.JList). The top list shows the contents of memory. The bottom list shows the program currently being executed. The control panel on the left contains three buttons (javax.swing.JButton). The first button prompts the user for a program to parse: A screenshot of a computer program Description automatically generated The second button executes the program. The third button resets all memory cells to 0. These commands are duplicated under the Edit menu. The Help menu explains each command. The File menu contains the items: New, Save, Open, and Quit. The MiniMax memory is an array of integers: int size = 32; Integer[] memory = new Integer[size]; A MiniMax program is a list of instructions. An instruction pointer (ip) indicates the position in the list of the next instruction to be executed. Grammar Here’s the instruction set grammar (note: “~” means “followed by” and all unquoted tokens are integers): load ::= “load” ~ location ~ value // memory[location] = value halt ::= “halt” // terminates the program add ::= “add” ~ src1 ~ src2 ~ dest // memory[dest] = memory[src1] + memory[src2] mul ::= “mul” ~ src1 ~ src2 ~ dest // memory[dest] = memory[src1] * memory[src2] bgt ::= “bgt” ~ location ~ offset // if 0 < memory[location] ip += offset blt ::= “blt” ~ location ~ offset // if memory[location] < 0 ip += offset loop ::= “loop ~ count ~ instruction // executes instruction count times A block is a list of one or more instructions separated by semicolons and bracketed by curly braces: block ::= “{“ ~ instruction ~ (“;” ~ instruction)* ~ “}” Executing a block sequentially executes each instruction in the block’s body. For example, the program shown in the screenshot computes kn, where n is stored in memory[0]. The base, k, is stored in memory[2]. In this case k = 2, n was 7 and the result, 128, is stored in memory[1]. Memory[3] is the amount to decrement memory[0] each time the result is multiplied by k. Design Here’s the design of MiniMac: A diagram of a program Description automatically generated Parser Here’s a partial implementation of MiniMacParser.java. Note that it is a utility class (aka singleton), all of its methods are static. Testing Implement and test the following functions in MiniMac: Tri(n) = 1 + 2 + ... + n // stored in a file called tri Fib(n) = nth Fibonacci number // stored in a file called fib Less(n, m) = (n < m)?1:0 // 1 = true and 0 = false Log(n) = m where 2m <= n and n < 2m+1 In each case the output, f(n), should be stored in memory[0] and the inputs should be stored beginning in memory[1]. Hints 1. The MiniMac is a publisher and the view panel is its subscriber. Each time memory is updated or a new program is set, MiniMac notifies its subscribers. 2. The control panel is the action listener for its buttons. It navigates to the MiniMac and calls the appropriate procedure (execute and clear). 3. Parsing is more complicated. The control panel must prompt the user for a file name, read the file (as a string), then pass the string to MiniMaxParser.parse(program). It then sets the MiniMac program to the parser’s output, which causes a subscriber notification. Suggestion: ask ChatGPT how to read a text file in Java. 4. Ask ChatGPT for a simple example of how to use a JList.
Chapter16: Graphics
Section: Chapter Questions
Problem 3GZ
Related questions
Question
MiniMac
MiniMac is a virtual processor with a tiny but extendable memory and a tiny but extendable instruction set.
Here’s a screenshot of the MiniMac user interface:
A screenshot of a computer
Description automatically generated
The view panel on the right contains two lists (javax.swing.JList). The top list shows the contents of memory. The bottom list shows the program currently being executed. The control panel on the left contains three buttons (javax.swing.JButton). The first button prompts the user for a program to parse:
A screenshot of a computer program
Description automatically generated
The second button executes the program. The third button resets all memory cells to 0.
These commands are duplicated under the Edit menu. The Help menu explains each command. The File menu contains the items: New, Save, Open, and Quit.
The MiniMax memory is an array of integers:
int size = 32;
Integer[] memory = new Integer[size];
A MiniMax program is a list of instructions. An instruction pointer (ip) indicates the position in the list of the next instruction to be executed.
Grammar
Here’s the instruction set grammar (note: “~” means “followed by” and all unquoted tokens are integers):
load ::= “load” ~ location ~ value // memory[location] = value
halt ::= “halt” // terminates the program
add ::= “add” ~ src1 ~ src2 ~ dest // memory[dest] = memory[src1] + memory[src2]
mul ::= “mul” ~ src1 ~ src2 ~ dest // memory[dest] = memory[src1] * memory[src2]
bgt ::= “bgt” ~ location ~ offset // if 0 < memory[location] ip += offset
blt ::= “blt” ~ location ~ offset // if memory[location] < 0 ip += offset
loop ::= “loop ~ count ~ instruction // executes instruction count times
A block is a list of one or more instructions separated by semicolons and bracketed by curly braces:
block ::= “{“ ~ instruction ~ (“;” ~ instruction)* ~ “}”
Executing a block sequentially executes each instruction in the block’s body.
For example, the program shown in the screenshot computes kn, where n is stored in memory[0]. The base, k, is stored in memory[2]. In this case k = 2, n was 7 and the result, 128, is stored in memory[1]. Memory[3] is the amount to decrement memory[0] each time the result is multiplied by k.
Design
Here’s the design of MiniMac:
A diagram of a program
Description automatically generated
Parser
Here’s a partial implementation of MiniMacParser.java. Note that it is a utility class (aka singleton), all of its methods are static.
Testing
Implement and test the following functions in MiniMac:
Tri(n) = 1 + 2 + ... + n // stored in a file called tri
Fib(n) = nth Fibonacci number // stored in a file called fib
Less(n, m) = (n < m)?1:0 // 1 = true and 0 = false
Log(n) = m where 2m <= n and n < 2m+1
In each case the output, f(n), should be stored in memory[0] and the inputs should be stored beginning in memory[1].
Hints
1. The MiniMac is a publisher and the view panel is its subscriber. Each time memory is updated or a new program is set, MiniMac notifies its subscribers.
2. The control panel is the action listener for its buttons. It navigates to the MiniMac and calls the appropriate procedure (execute and clear).
3. Parsing is more complicated. The control panel must prompt the user for a file name, read the file (as a string), then pass the string to MiniMaxParser.parse(program). It then sets the MiniMac program to the parser’s output, which causes a subscriber notification. Suggestion: ask ChatGPT how to read a text file in Java.
4. Ask ChatGPT for a simple example of how to use a JList.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
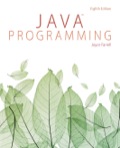
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
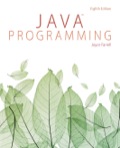
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT