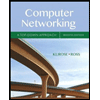
Assignment 7 B: Battle! Language is in C++ (pictures include the sample output)
Recreate a basic battle system from a video game. You will create a character and then use it to
battle a computer generated opponent. There are three possible classes, with the
following attributes:
Sword Fighter Dance Battler
HP: 120
Attack: 40
Defense: 0.20
ClassID: 1
Unicorn Sorcerer
HP: 80
Attack: 35
Defense: 0.60
ClassID: 2
Dance Battler
HP: 100
Attack: 20
Defense: 0.42
ClassID: 3
You will create a NPC class that has these three attribute. It should also have an
attribute for Name.
You will then create the following methods in the NPC class:
A constructor that takes in a Name string and a ClassID int. It should use the
value of the ClassID to assign values to the other attributes (using the chart
above)
Getter methods for all five attributes, and a Setter method for HP that subtracts
the object’s HP by the value passed in.
calculateAttack
◦ This method returns a float value. It takes in a float that represents the
opponent’s defense percentage. It should multiply the object’s attack by the
inverse of the opponent’s defense percentage, and return that value. For
example:
100 * (1 – 0.60) = 40
calculateDefense
◦ This method returns nothing. It takes in a float that represents the opponent’s
attack power. It should multiply the opponent’s attack by the inverse of the
object’s defense percentage, then subtract 6 additional points from the result.
This value should then be subtracted from the object’s HP.
isStillAlive
◦ This method returns a boolean value. It should check if the object’s HP is less
than or equal to 0. If it is, then return FALSE. Otherwise, return TRUE.
You may add other “helper” methods to reduce redundant code, although this is
not required.
You should then create a driver class, Assignment7B. This program should do the
following:
Prompt the user for a custom name and what class they want their character to
be. You should use this information to create an NPC object.
Randomly generate another NPC object to represent the opponent. You can do
this by randomly generating the two primitive values, then using them to
construct the opponent NPC.
Create a loop that runs until one of the opponents runs out of HP. You will first
prompt the player to either attack or defend. Then, you will have the opponent
randomly choose to either attack or defend.
◦ If both the human and computer player choose to defend, do nothing.
◦ If both of them attack, then call the calculateAttack function on both objects.
◦ If one attacks and one defends, call the calculateDefense function on the
defending object.
After both choices are made, display messages indicating the actions take and
the remaining HP for both sides.
When one or both players are defeated, stop the loop and print the results
Sample Output:
[Generic RPG Battle System]
Enter your name: Morgan
Enter your battle class: Unicorn Sorcerer
Morgan the Unicorn Sorcerer, your opponent is Brad the Battle Dancer!
-Round 1-
Do you (a)ttack or (d)efend? a
Morgan the Unicorn Sorcerer attacked Brad the Battle Dancer!
Brad now has 79.7 HP.
Brad the Battle Dancer attacked Morgan the Unicorn Sorcerer!
Morgan now has 72 HP.
-Round 2-
Do you (a)ttack or (d)efend? d
Morgan the Unicorn Sorcerer is on guard.
Brad the Battle Dancer is on guard.
-Round 3-
Do you (a)ttack or (d)efend? d
Morgan the Unicorn Sorcerer is on guard.
Brad the Battle Dancer attacked Morgan the Unicorn Sorcerer!
Morgan now has 70 HP.
//Skipping ahead to the end of this epic battle (THIS IS NOT PART OF
//THE OUTPUT
Brad the Battle Dancer was defeated...
Morgan the Unicorn Sorcerer wins!
lang in C++

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 7 images

- QUESTION 7 Final data/code constructs are only inheritable once. O True O False QUESTION 8 You can include code in an interface by using the keywordarrow_forwardAssignment:The BankAccount class models an account of a customer. A BankAccount has the followinginstance variables: A unique account id sequentially assigned when the Bank Account is created. A balance which represents the amount of money in the account A date created which is the date on which the account is created.The following methods are defined in the BankAccount class: Withdraw – subtract money from the balance Deposit – add money to the balance Inquiry on:o Balanceo Account ido Date createdarrow_forwardclass Player { protected: string name; double weight; double height; public: Player(string n, double w, double h) { name = n; weight = w; height = h; } string getName() const { return name; } virtual void printStats() const = 0; }; class BasketballPlayer : public Player { private: int fieldgoals; int attempts; public: BasketballPlayer(string n, double w, double h, int fg, int a) : Player(n, w, h) { fieldgoals = fg; attempts = a; } void printStats() const { cout << name << endl; cout << "Weight: " << weight; cout << " Height: " << height << endl; cout << "FG: " << fieldgoals; cout << " attempts: " << attempts; cout << " Pct: " << (double) fieldgoals / attempts << endl; } }; a. What does = 0 after function printStats() do? b. Would the following line in main() compile: Player p; -- why or why not? c. Could…arrow_forward
- A class object can encapsulate more than one [answer].arrow_forwardint x //x coord of the center -int y // y coord of the center -int radius -static int count // static variable to keep count of number of circles created + Circle() //default constructor that sets origin to (0,0) and radius to 1 +Circle(int x, int y, int radius) // regular constructor +getX(): int +getY(): int +getRadius(): int +setX(int newX: void +setY(int newY): void +setRadius(int newRadius):void +getArea(): double // returns the area using formula pi*r^2 +getCircumference // returns the circumference using the formula 2*pi*r +toString(): String // return the circle as a string in the form (x,y) : radius +getDistance(Circle other): double // *** returns the distance between the center of this circle and the other circle +moveTo(int newX,int newY):void // *** move the center of the circle to the new coordinates +intersects(Circle other): bool //*** returns true if the center of the other circle lies inside this circle else returns false +resize(double…arrow_forwardNutritional information (classes/constructors) PYTHON ONLY Complete the FoodItem class by adding a constructor to initialize a food item. The constructor should initialize the name (a string) to "None" and all other instance attributes to 0.0 by default. If the constructor is called with a food name, grams of fat, grams of carbohydrates, and grams of protein, the constructor should assign each instance attribute with the appropriate parameter value. The given program accepts as input a food item name, fat, carbs, and protein and the number of servings. The program creates a food item using the constructor parameters' default values and a food item using the input values. The program outputs the nutritional information and calories per serving for both food items. Ex: If the input is: M&M's10.034.02.01.0 where M&M's is the food name, 10.0 is the grams of fat, 34.0 is the grams of carbohydrates, 2.0 is the grams of protein, and 1.0 is the number of servings, the output is:…arrow_forward
- Write a program that will contain an array of person class objects. The program should include two classes: 1) One class will be the person class. 2) The second class will be the team class, which contain the main method and the array or array list. The person class should include the following data fields; Name Phone number Birth Date Jersey Number Be sure to include get and set methods for each data field.The team class should contain the data fields for the team, such as: Team name Coach name Conference name The program should include the following functionality. Add person objects to the array; Find a specific person object in the array; (find a person using any data field you choose such as name or jersey number) Output the contents of the array, including all data fields of each person object. (display roster)arrow_forwardCarDemo C# Programmingarrow_forwardQuadratic equations The form of a quadratic equation is ax2 + bx + c = 0. Write a program that solves a quadratic equation in all cases, including when both roots are complex numbers. Remember that the solutions are x = (-b + sqrt(b2 -4ac))/2a and (-b - sqrt(b2 -4ac))/2a For this you will need to set up the following classes: Complex: Encapsulates a complex number. ComplexPair: Encapsulates a pair of complex numbers. Quadratic: Encapsulates a quadratic equation. – Assume the coefficients are all of type int for this example. SolveEquation: Contains the main method. (I give this to you it is shown below) Along with the usual constructors, accessors and mutators you will need the following additional methods: In the Complex class a method called isReal to determine whether a complex object is real or not returning a boolean value. In the ComplexPair class a method bothIdentical that determines if both complex numbers are identical, returning a boolean value. In the…arrow_forward
- Convert the pseudocode into Python. 1. Pet ClassDesign a class named Pet, which should have the following fields:■ name: The name field holds the name of a pet.■ type: The type field holds the type of animal that a pet is. Example values are "Dog", "Cat", and "Bird".■ age: The age field holds the pet’s age.2. The Pet class should also have the following methods:■ setName: The setName method stores a value in the name field.■ setType: The setType method stores a value in the type field.■ setAge: The setAge method stores a value in the age field.■ getName: The getName method returns the value of the name field.■ getType: The getType method returns the value of the type field.■ getAge: The getAge method returns the value of the age field.3. Once you have designed the class, design a program that creates an object of the class and prompts the user to enter the name, type, and age of his or her pet. This data should be stored in the object. Use the…arrow_forwardJAVA CODE Class Description: Your need to create an instantiable class that represents a parcel of raw land that can be sold by a real estate company. This class should be named Land. It will contain three fields: a string named location and a width and length, measured in feet, each of which can accept numbers with decimal points. You may assume that every parcel of land is rectangular and that 'width' describes the size of the property along the road and 'length' is how far back it goes from the road. The class needs one constructor that sets the values all the fields. The class needs the following public methods: • Getters and setters for all fields. • A method called getArea that calculates and returns the area of the parcel of land in square feet. (Area is calculated as length x width.) • Standard methods: toString, equals, and hashCode where equals and hashCode define two equivalent parcels of land as each having the same length and each having the same width. • Implementation…arrow_forwardOZ PROGRAMMING LANGAUGE Write a class that specify the characteristics of a car, like type (sedan, jeep, mini, SUV, etc), gear (auto, manual), maximum speed (mph), average fuel consumption (mpg), etc. Create few objects to illustrate your desired cars.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
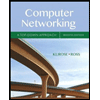
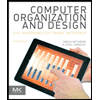
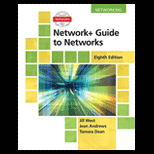
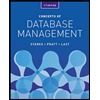
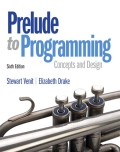
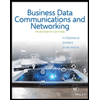