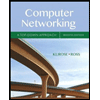
ample code for the reader-writer:
#include <pthread.h>
#include <semaphore.h>
#include <stdio.h>
/*
This program provides a possible solution for first readers writers problem using mutex and semaphore.
I have used 10 readers and 5 producers to demonstrate the solution. You can always play with these values.
*/
sem_t wrt;
pthread_mutex_t mutex;
int cnt = 1;
int numreader = 0;
void *writer(void *wno)
{
sem_wait(&wrt);
cnt = cnt*2;
printf("Writer %d modified cnt to %d\n",(*((int *)wno)),cnt);
sem_post(&wrt);
}
void *reader(void *rno)
{
// Reader acquire the lock before modifying numreader
pthread_mutex_lock(&mutex);
numreader++;
if(numreader == 1) {
sem_wait(&wrt); // If this id the first reader, then it will block the writer
}
pthread_mutex_unlock(&mutex);
// Reading Section
printf("Reader %d: read cnt as %d\n",*((int *)rno),cnt);
// Reader acquire the lock before modifying numreader
pthread_mutex_lock(&mutex);
numreader--;
if(numreader == 0) {
sem_post(&wrt); // If this is the last reader, it will wake up the writer.
}
pthread_mutex_unlock(&mutex);
}
int main()
{
pthread_t read[10],write[5];
pthread_mutex_init(&mutex, NULL);
sem_init(&wrt,0,1);
int a[10] = {1,2,3,4,5,6,7,8,9,10}; //Just used for numbering the producer and consumer
for(int i = 0; i < 10; i++) {
pthread_create(&read[i], NULL, (void *)reader, (void *)&a[i]);
}
for(int i = 0; i < 5; i++) {
pthread_create(&write[i], NULL, (void *)writer, (void *)&a[i]);
}
for(int i = 0; i < 10; i++) {
pthread_join(read[i], NULL);
}
for(int i = 0; i < 5; i++) {
pthread_join(write[i], NULL);
}
pthread_mutex_destroy(&mutex);
sem_destroy(&wrt);
return 0;
}
Consider the sample code for the reader-writer problem below. Change this code by adding counting semaphores to implement the scenario where the number of active readers is upper-bounded by N.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Determine all the output from the following program as it would appear on the screen. void func1(int); void func2(int = 4, int = 5, int = 2); int func3(int &, int, int); %3D int main() { int x = 0, z = 0, y = 2; func1(y); cout << y << endl; func2(x, y, z); func2(); func3(x, y, z); = Z func1(x); cout << x << " " << y << " " << z << endl; return 0; } void func1(int b) { static int a;arrow_forwardThis assignment is not graded, I just need to understand how to do it. Please help, thank you! Language: C++ Given: Main.cpp #include #include "Shape.h" using namespace std; void main() { /////// Untouchable Block #1 ////////// Shape* shape; /////// End of Untouchable Block #1 ////////// /////// Untouchable Block #2 ////////// if (shape == nullptr) { cout << "What shape is this?! Good bye!"; return; } cout << "The perimeter of your " << shape->getShapeName() << ": " << shape->getPerimeter() << endl; cout << "The area of your " << shape->getShapeName() << ": " << shape->getArea() << endl; /////// End of Untouchable Block #2 //////////} Shape.cpp string Shape::getShapeName() { switch (mShapeType) { case ShapeType::CIRCLE: return "circle"; case ShapeType::SQUARE: return "square"; case ShapeType::RECTANGLE: return "rectangle"; case…arrow_forwardc++arrow_forward
- Ruby Programming: Write a function that takes a number x as a parameter and returns the value of x3 Test the function for values x= 2 and x= 3. Output should be 8 and 27 respectively.arrow_forwardComputer Science Part C: Interactive Driver Program Write an interactive driver program that creates a Course object (you can decide the name and roster/waitlist sizes). Then, use a loop to interactively allow the user to add students, drop students, or view the course. Display the result (success/failure) of each add/drop.arrow_forwardIn Python, grades_dict = {'Wally': [87,96,70], 'Eva': [100,87,90], 'Sam': [94,77,90], 'Katie': [100,81,82], 'Bob': [83, 65, 85]} write your own describe function that produces thesame 8 statistical results, for each one of the columns, that the built-in describe() function does.Note 1: Use the sample standard deviation formula (that is, the denominator is: N-1)Note 2: Your algorithm should work for any number of columns not just for 5Note 3: You can use the np.percentile() for the 25% and 75% percentile as well as the sort()built-in functionsarrow_forward
- Integer userValue is read from input. Assume userValue is greater than 1000 and less than 99999. Assign tensDigit with userValue's tens place value. Ex: If the input is 15876, then the output is: The value in the tens place is: 7 2 3 public class ValueFinder { 4 5 6 7 8 9 10 11 12 13 GHE 14 15 16} public static void main(String[] args) { new Scanner(System.in); } Scanner scnr int userValue; int tensDigit; int tempVal; userValue = scnr.nextInt(); Your code goes here */ 11 System.out.println("The value in the tens place is: + tensDigit);arrow_forwardFind errors / syntax error. Write line numberarrow_forwardC++ code. Please describe what EACH line means/does. Ignore code that is commented out.arrow_forward
- Welcome Assignment ### welcome_assignment_answers ### Input - All eight questions given in the assignment. ### Output - The right answer for the specific question. def welcome_assignment_answers(question): # The student doesn't have to follow the skeleton for this assignment. # Another way to implement it is using "case" statements similar to C. if question == "Are encoding and encryption the same? - Yes/No": answer = "The student should type the answer here" elif question == "Is it possible to decrypt a message without a key? - Yes/No": answer = "The student should type the answer here" return (answer) # Complete all the questions. if __name__ == "__main__": # use this space to debug and verify that the program works debug_question = "Are encoding and encryption the same? - Yes/No" print(welcome_assignment_answers(debug_question)) As you can see, the first two questions are already in the skeleton code. Please follow the first two questions…arrow_forwardInstructions: In the code editor, you are provided with the definition of a struct Person. This struct needs an integer value for its age and a character value for its gender. Furthermore, you are provided with a displayPerson() function which accepts a struct Person as its parameter. In the main(), there are two Persons already created: one Male Person and one Female Person. Your task is to first ask the user for the age of the Male Person and the age of the Female Person. Then, define and declare a function called createKidPerson() which has the following definition: Return type - Person Name - createKidPerson Parameters Person father - the father of the kid to be created Person mother - the mother of the kid to be created Description - creates a new Person and returns this. The age of this Person will be set to 1 while its gender will be set based on the rules mentioned above. Finally, create a new Person and call this createKidPerson() in the main and then pass this newly…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
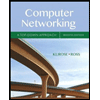
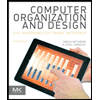
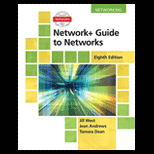
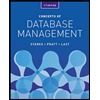
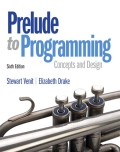
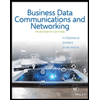