Algortimhs Recursive Thinking) Carefully read the comment of the following function, then complete the function so that it correctly solves the problem. Verify your answer by writing down the three questions rule and answer the questions for your completed algorithm. /* Return true if there exist duplicate values in sublist a[first...last], i.e., there are a[i]==a[j] for some i,j where i is not equal to j @param a: the vector/list @param first, last: two integer specifying the starting and ending indices of list @return return true if there are duplicate values in a[first...last], otherwise return false */ bool ContainDuplicate (vector a, int first, int last) { //1. what should be the base case? What should be returned? // general case if (ContainDuplicate (a, first+1, last)==true) _______________; //Finish the rest based upon comment: // if a[first] does not equal to ANY value in a[first+1...last], // then return false; // else return true } (Recursive Thinking) For the problem, think of a different way to solve it recursively. Write your algorithm in the space below, and verify your algorithm using the three-questions rule.
Algortimhs
Recursive Thinking) Carefully read the comment of the following function, then complete the function so that it correctly solves the problem. Verify your answer by writing down the three questions rule and answer the questions for your completed
/* Return true if there exist duplicate values in sublist a[first...last], i.e., there are a[i]==a[j] for some i,j where i is not equal to j
@param a: the
indices of list
@return return true if there are duplicate values in a[first...last],
otherwise return false */ bool ContainDuplicate (vector<int> a, int first, int last) {
//1. what should be the base case? What should be returned?
// general case if (ContainDuplicate (a, first+1, last)==true)
_______________;
//Finish the rest based upon comment:
// if a[first] does not equal to ANY value in a[first+1...last], // then return false;
// else return true
}
(Recursive Thinking) For the problem, think of a different way to solve it recursively. Write your algorithm in the space below, and verify your algorithm using the three-questions rule.

Answer:
I have done code and also I have attached code as well as output
Step by step
Solved in 4 steps with 1 images

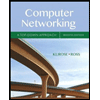
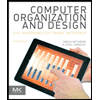
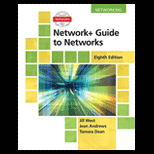
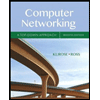
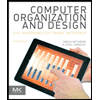
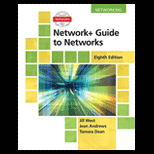
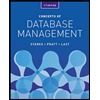
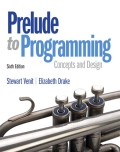
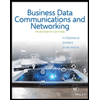