#include using namespace std; int main() { double hours, pay, gross, regular, OT, OT1; cout << "Enter pay rate:"; cin >> pay; cout << "Enter hours worked: "; cin >> hours; if (hours >= 40) { regular = hours * pay; OT = 0; OT1 = 0; gross = regular + OT + OT1; cout << "Regular hours: " << hours << "@" << pay << " $" << regular << endl; cout << "Ovetime hours: " << hours - 40 << "@" << pay << " $" << OT << endl;; cout << "Ovetime1 hours: " << "0" << "@" << pay << " $" << OT1 << endl;; cout << "Total gross pay: " << gross; } else if (hours < 40 && hours >= 54) { regular = 40 * pay; OT = regular + (hours - 40) * (pay * 1.5); OT1 = 0; gross = regular + OT + OT1; cout << "Regular hours: " << hours << "@" << pay << " $" << regular << endl; cout << "Ovetime hours: " << hours - 40 << "@" << pay << " $" << OT << endl; cout << "Ovetime1 hours: " << hours - 54 << "@" << pay << " $" << OT1 << endl; cout << "Total gross pay: " << gross; } else if (hours > 54) { regular = 40 * pay; OT = regular + (hours - 40) * (pay * 1.5); OT1 = OT + (hours - 54) * (pay * 2); gross = regular + OT + OT1; cout << "Regular hours: " << hours << "@" << pay << " $" << regular << endl; cout << "Ovetime hours: " << hours - 40 << "@" << pay << " $" << OT << endl; cout << "Ovetime1 hours: " << hours - 54 << "@" << pay << " $" << OT1 << endl; cout << "Total gross pay: " << gross; } return 0; I am wondering why my code only runs the first if statement even though I input hours greater than 40
#include <iostream>
using namespace std;
int main()
{
double hours, pay, gross, regular, OT, OT1;
cout << "Enter pay rate:";
cin >> pay;
cout << "Enter hours worked: ";
cin >> hours;
if (hours >= 40)
{
regular = hours * pay;
OT = 0;
OT1 = 0;
gross = regular + OT + OT1;
cout << "Regular hours: " << hours << "@" << pay << " $" << regular << endl;
cout << "Ovetime hours: " << hours - 40 << "@" << pay << " $" << OT << endl;;
cout << "Ovetime1 hours: " << "0" << "@" << pay << " $" << OT1 << endl;;
cout << "Total gross pay: " << gross;
}
else if (hours < 40 && hours >= 54)
{
regular = 40 * pay;
OT = regular + (hours - 40) * (pay * 1.5);
OT1 = 0;
gross = regular + OT + OT1;
cout << "Regular hours: " << hours << "@" << pay << " $" << regular << endl;
cout << "Ovetime hours: " << hours - 40 << "@" << pay << " $" << OT << endl;
cout << "Ovetime1 hours: " << hours - 54 << "@" << pay << " $" << OT1 << endl;
cout << "Total gross pay: " << gross;
}
else if (hours > 54)
{
regular = 40 * pay;
OT = regular + (hours - 40) * (pay * 1.5);
OT1 = OT + (hours - 54) * (pay * 2);
gross = regular + OT + OT1;
cout << "Regular hours: " << hours << "@" << pay << " $" << regular << endl;
cout << "Ovetime hours: " << hours - 40 << "@" << pay << " $" << OT << endl;
cout << "Ovetime1 hours: " << hours - 54 << "@" << pay << " $" << OT1 << endl;
cout << "Total gross pay: " << gross;
}
return 0;
I am wondering why my code only runs the first if statement even though I input hours greater than 40

Step by step
Solved in 3 steps with 3 images

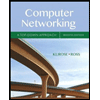
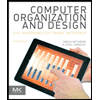
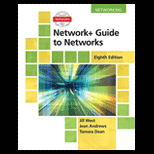
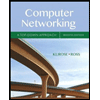
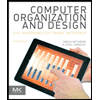
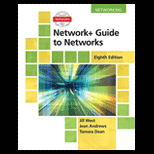
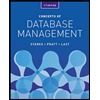
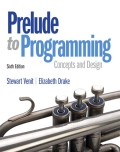
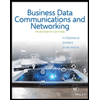