Assume a linked list contains following integers: 7, 2, 9, 5, 8, 3, 15 and the pointer head is pointing to the first node of the list. What will be the value of variable a after the following statements are executed: Node *curNode=head; int a; while(curNode->getNext()!=NULL){ { curNode=curNode->getNext(); } a=curNode->getItem(); A. 15 B. 7 C. 49 D. 3
Assume a linked list contains following integers: 7, 2, 9, 5, 8, 3, 15 and the pointer head is pointing to the first node of the list. What will be the value of variable a after the following statements are executed:
Node<int> *curNode=head;
int a;
while(curNode->getNext()!=NULL){
{
curNode=curNode->getNext();
}
a=curNode->getItem();
A. 15
B. 7
C. 49
D. 3

In the given Linked list, the pointer variable curNode initially points to the head of the linked list.
The while loop will execute until the last element of the linked list.
The statement curNode = curNode->getnext() retrieves the next element of the linked list and the pointer points to this node each time when the loop executes.
Finally the pointer curNode points to the last node with the help of the statement a = curNode->getItem();
Step by step
Solved in 2 steps

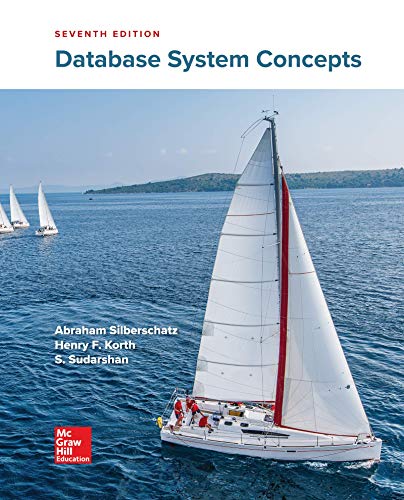
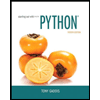
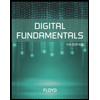
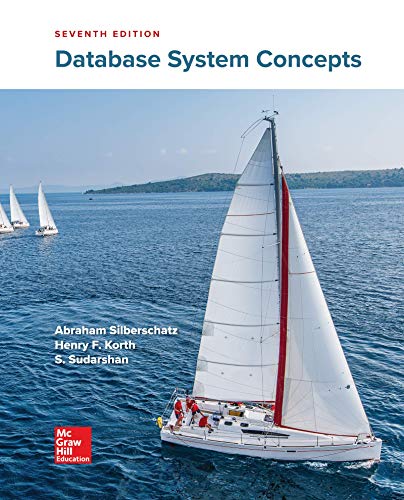
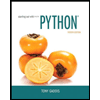
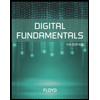
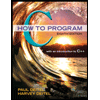
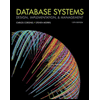
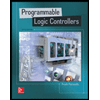