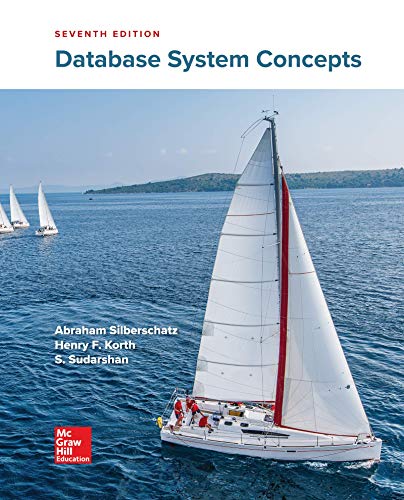
Write a C++ program that will:
5.1 Accept student’s information into three parallel arrays. The capture of the students’ marks
must be a sentinel-controlled mark that is below zero for either the continuous assessment
(term) mark or the final mark. Use a sentinel-controlled loop that will exit when the user
types in the word ’Done’ instead of a student’s name. For each mark, use a function: int
validateData(string,int) that receives a message to be displayed to the user in case the
mark is not acceptable, and also the captured mark. Marks can only be between 0 and 100.
5.2 The program should be menu driven, with the options: Capture Student Marks, Sort the Class List, Display the Class List, Exit.
5.3 Use any sort
of the list. The Display option should display the values of the array whether sorted or not
yet sorted, neatly formatting the columns of data.
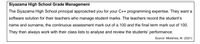

Step by stepSolved in 2 steps with 2 images

- For this project the program should be made in C++ Write a menu driven program that reads an array of 10 integer from standard keyboard (user) utilizing a loop and outputs the following depending on user's choice Choice 1 - Prints the elements of array Choice 2 - Prints the minimum value of the array Choice 3 - Prints the maximum value of the array Choice 4 - Prints the product of all the numbers utilizing a loop. Choice 5 - Prints the sum of all the numbers utilizing a loop Choice 6- Print the elements of array in a reverse order When the program is done, your program should ask the user if they would like to enter another set of 10 numbers and the program should repeat itself. Input validation: Your program should only accepts integers.arrow_forwardWrite a program that reads movie data from a CSV (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the CSV file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator ('|') in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44 characters, output the first 44 characters only. Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the CSV file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Hints: Use the find() function to find the index of a comma in each row of…arrow_forwardC++arrow_forward
- in the C++ version please suppose to have a score corresponding with probabilities at the end and do not use the count[] function. Please explain the detail when coding. DO NOT USE ARRAY. The game of Pig The game of Pig is a dice game with simple rules: Two players race to reach 100 points. Each turn, a player repeatedly rolls a die until either a 1 ("pig") is rolled or the player holds and scores the sum of the rolls (i.e. the turn total). At any time during a player's turn, the player is faced with two decisions: roll - if the player rolls 1: the player scores nothing and it becomes the opponents turn. 2 - 6: the number is added to the player's turn total and the player's turn continues. hold - The turn total is added to the player's score and it becomes the opponent's turn. This game is a game of probability. Players can use their knowledge of probabilities to make an educated game decision. Assignment specifications Hold-at-20 means that the player will choose to roll…arrow_forwardGiven an array of integers of size 10. Write a C++ function that takes the array as a parameter and returns the numbers of elements in the array that are small. A number is called "small" if it is in the range 1..10 inclusive. int ele[10] = {12, 56, -18, 1, 6, 4, 92, 2, 34, 78};; Expected Output: 4arrow_forwardpost in python please and a coding editor too You are asked to help write a program that will help users purchase cookies. A user will input types of cookies and the number of cookies that they want, and your program will suggest how many boxes that they should order. There are 30 cookies per box, so your goal is to make sure that the user will buy enough boxes so that they will get the cookies that they want. Write a function cookie_order that takes a dictionary as a parameter containing cookie names as keys and numbers of those cookies as values. Have this function return a new dictionary that has cookie names and the number of boxes that should be purchased of each type. Hint: This means if someone wants 31 cookies, they need to buy 2 boxes of cookies of that type. You may want to consider using math.ceil for this problem. Examples: >>> cookie_order({'thin mints': 97}) {'thin mints': 4} >>> cookie_order({'thin mints':34, 'shortbread': 60, 'caramel delites': 71,…arrow_forward
- Imagine that you are a biomedical engineer analyzing DNA sequences. You have numerical measurements from two different measurement sources, m1 and m2, both of which are arrays. Write a function named dna that takes these two arrays as inputs. It should return a character array string of nucleotides (represented by the letters A, C, G and T). For a given index, i, the nucleotide in the string is: - ‘A’ if m1(i) >= 0 and m2(i) >= 0 - ‘C’ if m1(i) < 0 and m2(i) >= 0 - ‘G’ if m1(i) >= 0 and m2(i) < 0 - ‘T’ if m1(i) < 0 and m2(i) < 0 code to call the function m1 = [-2 -3 2.5 0.3]; m2 = [1.1 2.1 -0.8 0.1]; sequecne = dna(m1,m2); function dna Please use MATLABarrow_forwardWrit a program with c++ language to do the following tasks : The user can input one original image and target scales . According to the user’s requirement, this image can resized to the target scale. The program can show the original image and the resized image. *The core function should be Bilinear_interpolation(original image, target height, target width) The output of this function is the resized image and then the user can save it. *You can use image processing libraries to assist your codes, such as Opencv. The functions included in the libraries which are off‐the‐shelf can be used to read images, operate images and save images.arrow_forwardin c++ 1. Write a function that takes a 1 Dimensional array and an integer n andreturns the number of times ‘n’ appears in the array. If ‘n’ does not appearin the array, return -1.2. Write a function that takes a 2 Dimensional array and returns the positionof the first row with an odd sum. Assume that the column size is fixed at 4.If no sum is odd, return -13. Write a class, “pie”, that has a number of slices (int slices) as a privateproperty. Construct the pie with a number of slices and remove a slice witha function. Tell the user how many slices are in the pie.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
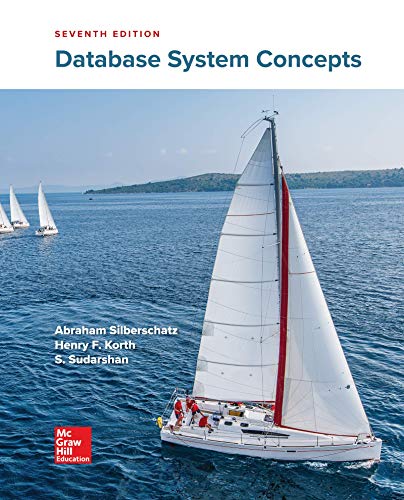
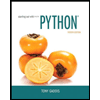
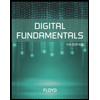
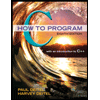
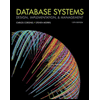
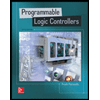