1.Use inheritance and classes to represent a deck playing cards. Create a Card class that stores the suit (e.g., Clubs, Diamonds, Hearts, Spades) and name (e.g., Ace, 2, 10, Jack) of each card along with appropriate accessors, constructors, and mutators.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
1.Use inheritance and classes to represent a deck playing cards. Create a Card class that stores the suit (e.g., Clubs, Diamonds, Hearts, Spades) and name (e.g., Ace, 2, 10, Jack) of each card along with appropriate accessors, constructors, and mutators.
2.Next, create a Deck class that stores an ArrayList of Card objects. The default constructor should create objects that represent the standard 52 cards and store them in the ArrayList. The Deck class should have methods to do the following:
-
-
Print every card in the deck.
-
Shuffle the cards in the deck. You can implement this by randomly swapping
every card in the deck. -
Add a new card to the deck. This method should take a Card object as a parameter and add it to the ArrayList.
-
Remove a card from the deck. This removes the first card stored in the ArrayList and returns it.
-
Sort the cards in the deck ordered by name.
-
3.Next, create a Hand class that represents the cards in a hand. Hand should be derived from Deck. This is because a hand is like a more specialized version of a deck; we can print, shuffle, add, remove, and sort cards in a hand just like cards in a deck. The default constructor should set the hand to an empty set of cards.
4.Finally, write a main method that creates a deck of cards, shuffles the deck, and creates two hands of 5 cards each. The cards should be removed from the deck and added to the hand. Test the sort and print functions for the hands and the deck. Finally, return the cards in the hand to the deck and test to ensure that the cards have been properly returned.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

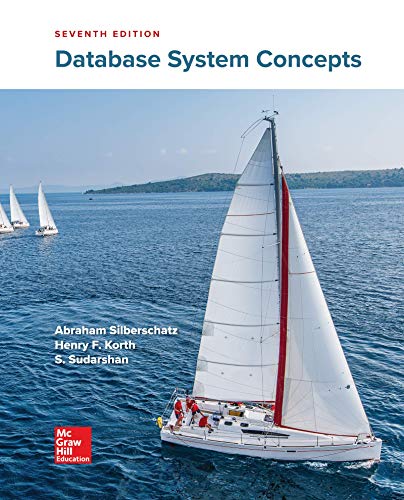
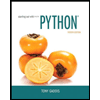
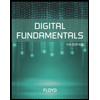
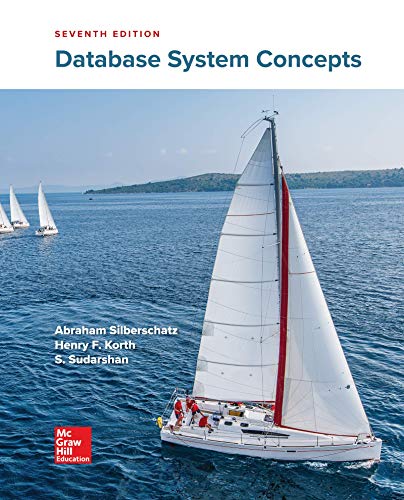
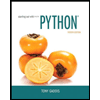
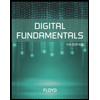
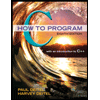
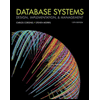
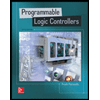