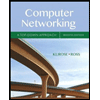
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
PLZ help
In Java
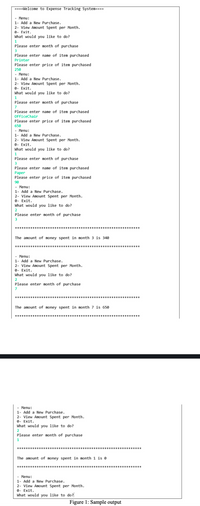
Transcribed Image Text:====Welcome to Expense Tracking System===-
- Menu:
1- Add a New Purchase.
2- View Amount Spent per Month.
e- Exit.
What would you like to do?
1
Please enter month of purchase
3
Please enter name of item purchased
Printer
Please enter price of item purchased
250
- Menu:
1- Add a New Purchase.
2- View Amount Spent per Month.
0- Exit.
What would you like to do?
1
Please enter month of purchase
7
Please enter name of item purchased
OfficeChair
Please enter price of item purchased
650
- Menu:
1- Add a New Purchase.
2- View Amount Spent per Month.
0- Exit.
What would you like to do?
1
Please enter month of purchase
3
Please enter name of item purchased
Paper
Please enter price of item purchased
90
- Menu:
1- Add a New Purchase.
2- View Amount Spent per Month.
0- Exit.
What would you like to do?
2
Please enter month of purchase
3
The amount of money spent in month 3 is 340
**
- Menu:
1- Add a New Purchase.
2- View Amount Spent per Month.
0- Exit.
What would you like to do?
Please enter month of purchase
7
The amount of money spent in month 7 is 650
***
- Menu:
1- Add a New Purchase.
2- View Amount Spent per Month.
0- Exit.
What would you like to do?
2
Please enter month of purchase
1
****
The amount of money spent in month 1 is e
- Menu:
1- Add a New Purchase.
2- View Amount Spent per Month.
9- Exit.
What would you like to do?
Figure 1: Sample output
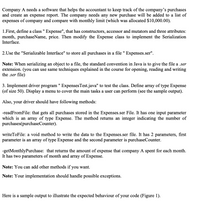
Transcribed Image Text:Company A needs a software that helps the accountant to keep track of the company's purchases
and create an expense report. The company needs any new purchase will be added to a list of
expenses of company and compare with monthly limit (which was allocated $10,000.00).
1.First, define a class " Expense", that has constructors, accessor and mutators and three attributes:
month, purchaseName, price. Then modify the Expense class to implement the Serialization
Interface.
2.Use the "Serializable Interface" to store all purchases in a file " Expenses.ser".
%3D
Note: When serializing an object to a file, the standard convention in Java is to give the file a .ser
extension. (you can use same techniques explained in the course for opening, reading and writing
the .ser file)
3. Implement driver program " ExpensesTest.java" to test the class. Define array of type Expense
(of size 50). Display a menu to cover the main tasks a user can perform (see the sample output).
Also, your driver should have following methods:
-readFromFile: that gets all purchases stored in the Expenses.ser File. It has one input parameter
which is an array of type Expense. The method returns an integer indicating the number of
purchases(purchaseCounter).
writeToFile: a void method to write the data to the Expenses.ser file. It has 2 parameters, first
parameter is an array of type Expense and the second parameter is purchaseCounter.
-getMonthlyPurchase: that returns the amount of expense that company A spent for each month.
It has two parameters of month and array of Expense.
Note: You can add other methods if you want.
Note: Your implementation should handle possible exceptions.
Here is a sample output to illustrate the expected behaviour of your code (Figure 1).
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps with 8 images

Knowledge Booster
Similar questions
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
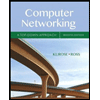
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
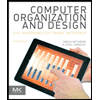
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
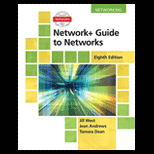
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
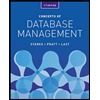
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
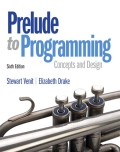
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
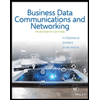
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY