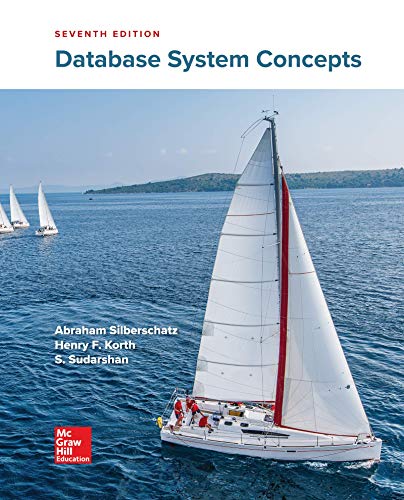
1. Display Function
- Implement a function to display the contents of the patient_list array.
- Add code to call this function. Note that there are multiple places where this function needs to be called. Look for the // TODO comments to find the correct locations.
2. Sorting the array by Age
- Implement the code to sort the contents of the patient_list array based on the value stored in the age field. To do this you will need to implement code that relies on the qsort function from the C Standard library
- A function that compares two patient elements, based on the value stored in the age field.
- A call to the qsort function, which includes the array to be sorted, the number of elements in the array, the size of each array element and the function used to compare the array elements.
- Add code to call the qsort function, using the age comparison function that you implemented. This code should be placed just under the appropriate // TODO comment in main().
Sorting the array by Balance Due
- Implement the code to sort the contents of the patient_list array based on the value stored in the balance field. To do this you will need to implement code that relies on the qsort function from the C Standard library
(see http://www.cplusplus.com/reference/cstdlib/qsort/). As shown in the reference, this code requires two parts: - A function that compares two patient elements, based on the value stored in the balance field.
- A call to the qsort function, which includes the array to be sorted, the number of elements in the array, the size of each array element and the function used to compare the array elements.
- Add code to call the qsort function, using the balance comparison function that you implemented. This code should be placed just under the appropriate // TODO comment in main().
Sorting the array by Patient Name
- Implement the code to sort the contents of the patient_list array based on the value stored in the name field. To do this you will need to implement code that relies on the qsort function from the C Standard library
(see http://www.cplusplus.com/reference/cstdlib/qsort/). As shown in the reference, this code requires two parts: - A function that compares two patient elements, based on the value stored in the name field. Because name data is stored in an array of characters, you cannot use the relational operators (<, >, <=, … ) to do the comparison, instead you should use a function from the C Standard Library to determine the order of the names, see (http://www.cplusplus.com/reference/cstring/strncmp/).
- A call to the qsort function, which includes the array to be sorted, the number of elements in the array, the size of each array element and the function used to compare the array elements.
- Add code to call the qsort function, using the name comparison function that you implemented. This code should be placed just under the appropriate // TODO comment in main().
OUTPUT IN IMAGES
#include <iostream>
#include <cstdlib>
#include <cstring>
using namespace std;
// Declaring a new struct to store patient data
struct patient {
int age;
char name[20];
float balance;
};
// TODO:
// IMPLEMENT A FUNCTION THAT COMPARES TWO PATIENTS BY AGE
// THE FUNCTION RETURNS AN INTEGER AS FOLLOWS:
// -1 IF THE AGE OF THE FIRST PATIENT IS LESS
// THAN THE SECOND PATIENT'S AGE
// 0 IF THE AGES ARE EQUAL
// 1 OTHERWISE
// TODO:
// IMPLEMENT A FUNCTION THAT COMPARES TWO PATIENTS BY BALANCE DUE
// THE FUNCTION RETURNS AN INTEGER AS FOLLOWS:
// -1 IF THE BALANCE FOR THE FIRST PATIENT IS LESS
// THAN THE SECOND PATIENT'S BALANCE
// 0 IF THE BALANCES ARE EQUAL
// 1 OTHERWISE
// TODO:
// IMPLEMENT A FUNCTION THAT COMPARES TWO PATIENTS BY NAME
// THE FUNCTION RETURNS AN INTEGER AS FOLLOWS:
// -1 IF THE NAME OF THE FIRST PATIENT GOES BEFORE
// THE SECOND PATIENT'S NAME
// 0 IF THE AGES ARE EQUAL
// 1 OTHERWISE
//
// HINT: USE THE strncmp FUNCTION
// (SEE http://www.cplusplus.com/reference/cstring/strncmp/)
// The main program
int main()
{
int total_patients = 5;
// Storing some test data
struct patient patient_list[5] = {
{25, "Juan Valdez ", 1250},
{15, "James Morris ", 2100},
{32, "Tyra Banks ", 750},
{62, "Maria O'Donell", 375},
{53, "Pablo Picasso ", 615}
};
cout << "Patient List: " << endl;
// TODO:
// IMPLEMENT THE CODE TO DISPLAY THE CONTENTS
// OF THE ARRAY BEFORE SORTING
cout << endl;
cout << "Sorting..." << endl;
// TODO:
// CALL THE qsort FUNCTION TO SORT THE ARRAY BY PATIENT AGE
cout << "Patient List - Sorted by Age: " << endl;
// TODO:
// DISPLAY THE CONTENTS OF THE ARRAY
// AFTER SORTING BY AGE
cout << endl;
cout << "Sorting..." << endl;
// TODO:
// CALL THE qsort FUNCTION TO SORT THE ARRAY BY PATIENT BALANCE
cout << "Patient List - Sorted by Balance Due: " << endl;
// TODO:
// DISPLAY THE CONTENTS OF THE ARRAY
// AFTER SORTING BY BALANCE
cout << endl;
cout << "Sorting..." << endl;
// TODO:
// CALL THE qsort FUNCTION TO SORT THE ARRAY BY PATIENT NAME
cout << "Patient List - Sorted by Name: " << endl;
// TODO:
// DISPLAY THE CONTENTS OF THE ARRAY
// AFTER SORTING BY NAME
cout << endl;
return 0;
}
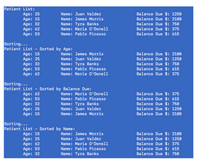

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- QUESTION 7 1) Write a maxConsecutiveDiff function which returns the maximum difference between any two consecutive values in the array. Consecutive values are next to each other in an array (+/- 1 index) This function should accept all required data as parameters. Examples If the array is 17 15 16 11 14 the maximum consecutive difference is 5 which is the difference between 16 and 11. If the array is 10 13 11 12 19 the maximum consecutive difference is 7 which is the difference between 12 and 19.arrow_forwardMatrix Multiplication• Write a multiplication function that accepts two 2D numpy arrays,and returns the product of the two. This function should test that thearrays are compatible (recall that the number of columns in the firstmatrix must equal the number of rows in the second matrix). Thisfunction should test the array sizes before attempting themultiplication. If the arrays are not compatible, the function shouldreturn -1. solve in pythonarrow_forward1- Write a user-defined function that accepts an array of integers. The function should generate the percentage each value in the array is of the total of all array values. Store the % value in another array. That array should also be declared as a formal parameter of the function. 2- In the main function, create a prompt that asks the user for inputs to the array. Ask the user to enter up to 20 values, and type -1 when done. (-1 is the sentinel value). It marks the end of the array. A user can put in any number of variables up to 20 (20 is the size of the array, it could be partially filled). 3- Display a table like the following example, showing each data value and what percentage each value is of the total of all array values. Do this by invoking the function in part 1.arrow_forward
- Computer Science csv file "/dsa/data/all_datasets/texas.csv" Task 6: Write a function "county_locator" that allows a user to enter in a name of a spatial data frame and a county name and have a map generated that shows the location of that county with respect to other counties (by using different colors and/or symbols). Hint: function(); $county ==; plot(); add=TRUE.arrow_forwardC code which returns the largest of the 6 integer number array according to integer array sent into it and the array length. Also include minimum, sum and average functions.arrow_forward/* (name header) #include // Function declaration int main() { int al[] int a2[] = { 7, 2, 10, 9 }; int a3[] = { 2, 10, 7, 2 }; int a4[] = { 2, 10 }; int a5[] = { 10, 2 }; int a6[] = { 2, 3 }; int a7[] = { 2, 2 }; int a8[] = { 2 }; int a9[] % { 5, 1, б, 1, 9, 9 }; int al0[] = { 7, 6, 8, 5 }; int all[] = { 7, 7, 6, 8, 5, 5, 6 }; int al2[] = { 10, 0 }; { 10, 3, 5, 6 }; %3D std::cout « ((difference (al, 4) « ((difference(a2, 4) <« ((difference (a3, 4) « ((difference(a4, 2) <« ((difference (a5, 2) <« ((difference (a6, 2) <« ((difference (a7, 2) <« ((difference(a8, 1) « ((difference (a9, 6) « ((difference(a10, 4) " <« ((difference(al1, 7) « ((difference (al2, 2) == 10) ? "OK\n" : "X\n"); << "al: << "a2: %3D 7)? "OK" : "X") << std::endl == 8) ? "OK\n" : "X\n") 8) ? "OK\n" : "X\n") == 8) ? "OK\n" : "X\n") == 8) ? "OK\n" : "X\n") == 1) ? "OK\n" : "X\n") == 0) ? "OK\n" : "X\n") 0) ? "OK\n" : "X\n") == 8) ? "OK\n" : "X\n") == 3) ? "OK\n" : "X\n") == 3) ? "OK\n" : "X\n") == << "a3: == << "a4: <<…arrow_forward
- # dates and times with lubridateinstall.packages("nycflights13") library(tidyverse)library(lubridate)library(nycflights13) Qustion: Create a function called date_quarter that accepts any vector of dates as its input and then returns the corresponding quarter for each date Examples: “2019-01-01” should return “Q1” “2011-05-23” should return “Q2” “1978-09-30” should return “Q3” Etc. Use the flight's data set from the nycflights13 package to test your function by creating a new column called quarter using mutate()arrow_forwarduse c++ Programming language Write a program that creates a two dimensional array initialized with test data. Use any data type you wish . The program should have following functions: .getAverage: This function should accept a two dimensional array as its argument and return the average of each row (each student have their average) and each column (class test average) all the values in the array. .getRowTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The function should return the total of the values in the specified row. .getColumnTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The function should return the total of the values in the specified column. .getHighestInRow: This function should accept a two…arrow_forwardc language and use int main format pleasearrow_forward
- Write code to declare array that contains names of 7 stores. Then write a statement that will call a function that will return the name of the store randomly.arrow_forwardGame of Hunt in C++ language Create the 'Game of Hunt'. The computer ‘hides’ the treasure at a random location in a 10x10 matrix. The user guesses the location by entering a row and column values. The game ends when the user locates the treasure or the treasure value is less than or equal to zero. Guesses in the wrong location will provide clues such as a compass direction or number of squares horizontally or vertically to the treasure. Using the random number generator, display one of the following in the board where the player made their guess: U# Treasure is up ‘#’ on the vertical axis (where # represents an integer number). D# Treasure is down ‘#’ on the vertical axis (where # represents an integer number) || Treasure is in this row, not up or down from the guess location. -> Treasure is to the right. <- Treasure is to the left. -- Treasure is in the same column, not left or right. +$ Adds $50 to treasure and no $50 turn loss. -$ Subtracts…arrow_forwardc++ programming Write a function named maxCols which stores the maximum value in each row of a 3x3 2D array into a 1D array. This function should accept a 3x3 2D array, a 1D array and the size of each dimension as parameters. Example Calling maxCols with this 2D array:3 7 2 9 3 6 8 5 6 Results in the 1D array storing the values 7,9,8.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
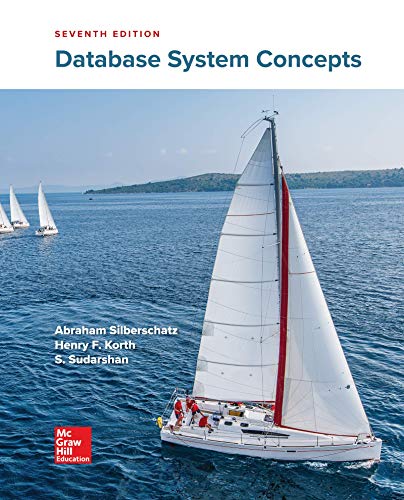
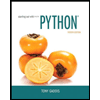
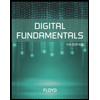
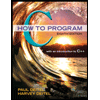
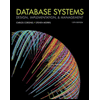
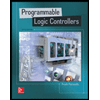