1. Add new patient 2. Add new physician record to a patient 3. Find patient by name 4. Find patient by birth date 5. Find the patients visit history 6. Display all patients 7. Print invoice that includes details of the visit and cost of each item done 8. Exit
Can someone help me complete what the image is asking using the code below as reference(You can change it a bit if you want) also using stacks, queues, linked lists, and Binary Search Trees.
#include<iostream>
using namespace::std;
class ERPHMS{
private:
int patient;
int visits; int turns;
int diagnostics;
int treatments;
int observation;
public:
void add_patient();
void new_physician_history();
void find_patient();
void find_pyhsician();
void patient_history();
void patient_registered();
void display_invoice();
};
int main() {
ERPHMS check; int choice;
cout<<endl<<"----------------------------------------------------------"<<endl; cout<<"This is an Emergency Room Patients Health Managment system "<<endl; cout<<"-----------------------------------------------------------"<<endl;
cout<<"1:For adding patient select"<<endl;
cout<<"2:For new physician History"<<endl;
cout<<"3:For finding patient"<<endl;
cout<<"4:For finding physician"<<endl;
cout<<"5:For patient History"<<endl;
cout<<"6:For patient registered"<<endl;
cout<<"7:To display Invoice"<<endl<<endl;
cout<<"Please pick the service : ";
cin>>choice;
switch(choice) {
case 1: check.add_patient(); break;
case 2: check.new_physician_history();
case 3: check.find_patient();
case 4: check.find_pyhsician();
case 5: check.patient_history();
case 6: check.patient_registered();
case 7: check.display_invoice();
}
}


Step by step
Solved in 2 steps with 1 images

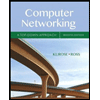
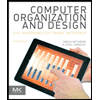
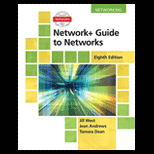
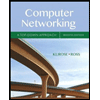
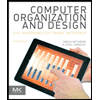
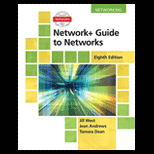
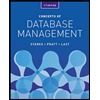
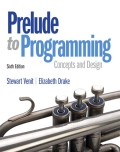
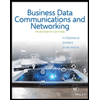