Document44
.docx
keyboard_arrow_up
School
Southern New Hampshire University *
*We aren’t endorsed by this school
Course
145
Subject
Computer Science
Date
Dec 6, 2023
Type
docx
Pages
20
Uploaded by LieutenantMinkPerson650
Joel Hernandez
IT-154
Project-2
//#1 Dog.java Class File
public class Dog extends RescueAnimal {
// Instance variable
private String breed;
// Constructor
public Dog(String name, String breed, String gender, String age,
String weight, String acquisitionDate, String acquisitionCountry,
String trainingStatus, boolean reserved, String inServiceCountry) {
setName(name);
setBreed(breed);
setGender(gender);
setAge(age);
setWeight(weight);
setAcquisitionDate(acquisitionDate);
setAcquisitionLocation(acquisitionCountry);
setTrainingStatus(trainingStatus);
setReserved(reserved);
setInServiceCountry(inServiceCountry);
}
// Accessor Method
public String getBreed() {
return breed;
}
// Mutator Method
public void setBreed(String dogBreed) {
breed = dogBreed;
}
}
//#2 Driver.java Class File
import java.util.ArrayList;
import java.util.Scanner;
public class Driver {
// Instance variables (if needed)
private static ArrayList<Dog> dogList = new ArrayList<Dog>();
private static ArrayList<Monkey> monkeyList = new ArrayList<Monkey>();
public static void main(String[] args) {
initializeDogList();
initializeMonkeyList();
// Controls the menu traversal
boolean acceptingInput = true;
Scanner input = new Scanner(System.in);
do {
displayMenu();
String option = input.nextLine().trim().toLowerCase();
switch(option) {
case "1":
// Input a new dog
intakeNewDog(input);
break;
case "2":
// Input a new monkey
intakeNewMonkey(input);
break;
case "3":
// Reserve an animal
reserveAnimal(input);
break;
case "4":
// Print all of the dogs
printAnimals("dog");
break;
case "5":
// Print all of the monkies
printAnimals("monkey");
break;
case "6":
// Print all non-reserved animals
printAnimals("available");
break;
case "q":
// Quit
acceptingInput = false;
break;
default:
System.out.println("Invalid option, please input a valid option");
break;
}
} while(acceptingInput);
System.out.println("Goodbye");
}
// This method prints the menu options
public static void displayMenu() {
System.out.println("\n\n");
System.out.println("\t\t\t\tRescue Animal System Menu");
System.out.println("[1] Intake a new dog");
System.out.println("[2] Intake a new monkey");
System.out.println("[3] Reserve an animal");
System.out.println("[4] Print a list of all dogs");
System.out.println("[5] Print a list of all monkeys");
System.out.println("[6] Print a list of all animals that are not reserved");
System.out.println("[q] Quit application");
System.out.println();
System.out.println("Enter a menu selection");
}
// Adds dogs to a list for testing
public static void initializeDogList() {
Dog dog1 = new Dog("Spot", "German Shepherd", "male", "1", "25.6", "05-12-2019", "United States",
"intake", false, "United States");
Dog dog2 = new Dog("Rex", "Great Dane", "male", "3", "35.2", "02-03-2020", "United States", "Phase I", false, "United States");
Dog dog3 = new Dog("Bella", "Chihuahua", "female", "4", "25.6", "12-12-2019", "Canada", "in service", true, "Canada");
dogList.add(dog1);
dogList.add(dog2);
dogList.add(dog3);
}
// Adds monkeys to a list for testing (optional)
public static void initializeMonkeyList() {
Monkey monkey1 = new Monkey("Cappy", "Capuchin", "male", "1", "5.6", "15", "10", "5", "05-21-
2021", "United States", "intake", false, "United States");
Monkey monkey2 = new Monkey("Max", "Macaque", "male", "3", "15.2", "63", "53", "10", "02-29-
2002", "United States", "Phase I", false, "United States");
Monkey monkey3 = new Monkey("Tammy", "Tamarin", "female", "4", "1.6", "9", "1", "0.6", "12-21-
2012", "Canada", "in service", false, "Canada");
monkeyList.add(monkey1);
monkeyList.add(monkey2);
monkeyList.add(monkey3);
}
// Adds a new dog to `dogList`
// intakeNewDog(scanner:Scanner) -> void
public static void intakeNewDog(Scanner scanner) {
System.out.println("What is the dog's name?");
String name = scanner.nextLine().trim();
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
public class Aircraft
{
// instance vairables
private String aircraftName;
private String regNumber;
private String manufacturer;
privateintmaxRange;
privateintcrewSize;
private LocalDate yearPutInService;
privateintmaxServiceWeight;
privateintnumberOfPassengers;
privateintcurrentAirMiles;
private LocalDate lastMaintenanceDate;
privateintlastMaintenanceMiles;
/**
* Default Constructor
*
* @param lastMaintenanceMiles
* @param lastMaintenanceDate
* @param totalMiles
* @param model
* @param manufacturer
* @param regNumber
* @param vehicleType
*/
public Aircraft(String aircraftName, String regNumber, String manufacturer, intmaxRange, intcrewSize,
LocalDate yearPutInService, int maxServiceWeight, int numberOfPassengers, int currentAirMiles,
LocalDate lastMaintenanceDate, int lastMaintenanceMiles)
{
{
this.aircraftName = aircraftName;
this.regNumber = regNumber;
this.manufacturer = manufacturer;
this.maxRange = maxRange;
this.crewSize = crewSize;
this.yearPutInService = yearPutInService;…
arrow_forward
class Player
{
protected:
string name;
double weight;
double height;
public:
Player(string n, double w, double h)
{ name = n; weight = w; height = h; }
string getName() const
{ return name; }
virtual void printStats() const = 0;
};
class BasketballPlayer : public Player
{
private:
int fieldgoals;
int attempts;
public:
BasketballPlayer(string n, double w,
double h, int fg, int a) : Player(n, w, h)
{ fieldgoals = fg; attempts = a; }
void printStats() const
{ cout << name << endl;
cout << "Weight: " << weight;
cout << " Height: " << height << endl;
cout << "FG: " << fieldgoals;
cout << " attempts: " << attempts;
cout << " Pct: " << (double) fieldgoals / attempts << endl; }
};
a. What does = 0 after function printStats() do?
b. Would the following line in main() compile: Player p; -- why or why not?
c. Could…
arrow_forward
public class Plant { protected String plantName; protected String plantCost;
public void setPlantName(String userPlantName) { plantName = userPlantName; }
public String getPlantName() { return plantName; }
public void setPlantCost(String userPlantCost) { plantCost = userPlantCost; }
public String getPlantCost() { return plantCost; }
public void printInfo() { System.out.println(" Plant name: " + plantName); System.out.println(" Cost: " + plantCost); }}
public class Flower extends Plant {
private boolean isAnnual; private String colorOfFlowers;
public void setPlantType(boolean userIsAnnual) { isAnnual = userIsAnnual; }
public boolean getPlantType(){ return isAnnual; }
public void setColorOfFlowers(String userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; }
public String getColorOfFlowers(){ return colorOfFlowers; } @Override public void printInfo(){…
arrow_forward
public class Person {
private String personID; private String firstName; private String lastName; private String birthDate; private String address;
public Person(){
personID = ""; firstName = ""; lastName = ""; birthDate = ""; address = "";
}
public Person(String id, String first, String last, String birth, String add){ setPerson(id,first,last,birth,add);
}
public void setPerson(String id, String first, String last, String birth, String add){
personID = id; firstName = first; lastName = last; birthDate = birth; address = add;
}
public String getFirstName(){
return firstName;
}
public String getLastName(){
return lastName;
}
public String getBirthdate(){
return birthDate;
}
public String getAddress(){ return address;
}
public void print(){
System.out.print("\nPerson ID = " + personID);
System.out.print("\nFirst Name = " +firstName);
System.out.print("\nLast Name = " +lastName);
System.out.print("\nBirth Date = " +birthDate); System.out.print("\nAddress = " +address);
}
public String…
arrow_forward
public class Person {
private String personID; private String firstName; private String lastName; private String birthDate; private String address;
public Person(){
personID = ""; firstName = ""; lastName = ""; birthDate = ""; address = "";
}
public Person(String id, String first, String last, String birth, String add){ setPerson(id,first,last,birth,add);
}
public void setPerson(String id, String first, String last, String birth, String add){
personID = id; firstName = first; lastName = last; birthDate = birth; address = add;
}
public String getFirstName(){
return firstName;
}
public String getLastName(){
return lastName;
}
public String getBirthdate(){
return birthDate;
}
public String getAddress(){ return address;
}
public void print(){
System.out.print("\nPerson ID = " + personID);
System.out.print("\nFirst Name = " +firstName);
System.out.print("\nLast Name = " +lastName);
System.out.print("\nBirth Date = " +birthDate); System.out.print("\nAddress = " +address);
}
public String…
arrow_forward
Instrument.java
public class Instrument {
protectedStringinstrumentName;
protectedStringinstrumentManufacturer;
protectedintyearBuilt, cost;
publicvoidsetName(StringuserName) {
instrumentName = userName;
}
publicStringgetName() {
return instrumentName;
}
publicvoidsetManufacturer(StringuserManufacturer) {
instrumentManufacturer = userManufacturer;
}
publicStringgetManufacturer(){
return instrumentManufacturer;
}
publicvoidsetYearBuilt(intuserYearBuilt) {
yearBuilt = userYearBuilt;
}
publicintgetYearBuilt() {
return yearBuilt;
}
publicvoidsetCost(intuserCost) {
cost = userCost;
}
publicintgetCost() {
return cost;
}
publicvoidprintInfo() {
System.out.println("Instrument Information: ");
System.out.println(" Name: " + instrumentName);
System.out.println(" Manufacturer: " + instrumentManufacturer);
System.out.println(" Year built: " + yearBuilt);
System.out.println(" Cost: " + cost);
}
}
StringInstrument.java
// TODO: Define a class: StringInstrument that is derived from…
arrow_forward
2
arrow_forward
public class Book {
protected String title; protected String author; protected String publisher; protected String publicationDate;
public void setTitle(String userTitle) { title = userTitle; }
public String getTitle() { return title; }
public void setAuthor(String userAuthor) { author = userAuthor; }
public String getAuthor(){ return author; }
public void setPublisher(String userPublisher) { publisher = userPublisher; }
public String getPublisher() { return publisher; }
public void setPublicationDate(String userPublicationDate) { publicationDate = userPublicationDate; }
public String getPublicationDate() { return publicationDate; }
public void printInfo() { System.out.println("Book Information: "); System.out.println(" Book Title: " + title); System.out.println(" Author: " + author); System.out.println(" Publisher: " + publisher); System.out.println(" Publication…
arrow_forward
class IndexItem { public: virtual int count() = 0; virtual void display()= 0; };class Book : public IndexItem { private: string title; string author; public: Book(string title, string author): title(title), author(author){} virtual int count(){ return 1; } virtual void display(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };class Category: public IndexItem { private: /* fill in the private member variables for the Category class below */
?
int count;
public: Category(string name, string code): name(name), code(code){} /* Implement the count function below. Consider the use of the function as depicted in main() */
?
/* Implement the add function which fills the category with contents below. Consider the use of the function as depicted in main() */
?
virtualvoiddisplay(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };
arrow_forward
class Course { private String courseNumber; private String courseName; private int creditHrs;
public Course (String number, String name, int creditHrs){ this.courseNumber = number; this.courseName = name; this.creditHrs = creditHrs; }
public String getNumber() { return courseNumber; }
public String getName() { return courseName; }
public int getCreditHrs() { return creditHrs; }
public void setCourseNumber(String courseNumber) { this.courseNumber = courseNumber; }
public void setCourseName(String courseName) { this.courseName = courseName; }
public void setCreditHrs(int creditHrs) { this.creditHrs = creditHrs; }}
class Student { private String firstName; private String lastName; private String gender; private String phoneNumber; private String email; private String jNumber; protected ArrayList<MyCourse> courseList;
public String…
arrow_forward
class Course { private String courseNumber; private String courseName; private int creditHrs;
public Course (String number, String name, int creditHrs){ this.courseNumber = number; this.courseName = name; this.creditHrs = creditHrs; }
public String getNumber() { return courseNumber; }
public String getName() { return courseName; }
public int getCreditHrs() { return creditHrs; }
public void setCourseNumber(String courseNumber) { this.courseNumber = courseNumber; }
public void setCourseName(String courseName) { this.courseName = courseName; }
public void setCreditHrs(int creditHrs) { this.creditHrs = creditHrs; }}class Student { private String firstName; private String lastName; private String gender; private String phoneNumber; private String email; private String jNumber; protected ArrayList courseList;
public String getFullName() {…
arrow_forward
C# problem
when printing Weigh no value shows up
code
public class HealthProfile{private String _FirstName;private String _LastName;private int _BirthYear;private double _Height;private double _Weigth;private int _CurrentYear;public HealthProfile(string firstName, string lastName, int birthYear, double height, double weigth, int currentYear){_FirstName = firstName;_LastName = lastName;_BirthYear = birthYear;_Height = height;_Weigth = weight;_CurrentYear = currentYear;}public string firstName { get; set; }public string lastName { get; set; }public int birthYear { get; set; }public int height { get; set; }public double weight { get; set; }public int currentYear { get; set; }public int Person_Age{get { return _CurrentYear - _BirthYear; }}public double Height{get { return _Height; }}public double Weight{get { return Weight; }}public int Max_Heart_Rate{get { return 220 - Person_Age; }}public double Min_Target_Heart_Rate{get { return Max_Heart_Rate * 0.65; }}public double…
arrow_forward
public class ItemToPurchase { private String itemName; private int itemPrice; private int itemQuantity;
// Default constructor public ItemToPurchase() { itemName = "none"; itemPrice = 0; itemQuantity = 0; }
// Mutator for itemName public void setName(String itemName) { this.itemName = itemName; }
// Accessor for itemName public String getName() { return itemName; }
// Mutator for itemPrice public void setPrice(int itemPrice) { this.itemPrice = itemPrice; }
// Accessor for itemPrice public int getPrice() { return itemPrice; }
// Mutator for itemQuantity public void setQuantity(int itemQuantity) { this.itemQuantity = itemQuantity; }
// Accessor for itemQuantity public int getQuantity() { return itemQuantity; }}
import java.util.Scanner;
public class ShoppingCartPrinter { public static void main(String[] args) { Scanner scnr = new…
arrow_forward
//PLEASE CORRECT MY MISTAKES, THANK YOUpublic class Person {
private String personID; private String firstName; private String lastName; private String birthDate; private String address;
public Person(){
personID = ""; firstName = ""; lastName = ""; birthDate = ""; address = "";
}
public Person(String id, String first, String last, String birth, String add){ setPerson(id,first,last,birth,add);
}
public void setPerson(String id, String first, String last, String birth, String add){
personID = id; firstName = first; lastName = last; birthDate = birth; address = add;
}
public String getFirstName(){
return firstName;
}
public String getLastName(){
return lastName;
}
public String getBirthdate(){
return birthDate;
}
public String getAddress(){ return address;
}
public void print(){
System.out.print("\nPerson ID = " + personID);
System.out.print("\nFirst Name = " +firstName);
System.out.print("\nLast Name = " +lastName);
System.out.print("\nBirth Date = " +birthDate);…
arrow_forward
Public classTestMain
{
public static void main(String [ ] args)
{
Car myCar1, myCar2;
Electric Car myElec1, myElec2;
myCar1 = new Car( );
myCar2 = new Car("Ford", 1200, "Green");
myElec1 = new ElectricCar( );
myElec2 = new ElectricCar(15);
}
}
arrow_forward
Class Quiz
public Quiz (int quesList, int quesMissed) {quesList = this.quesList;quesMissed = this.quesMissed;}
private void calculate(){// get the point worth of each question and calculate final scorepointsPerQues = (100/quesList);scoreQuiz = quesList - quesMissed;}
Class PassFailQuiz
public PassFailQuiz (int quesList, int quesMissed, int scoreQuiz) {
super(quesList, quesMissed);}
How do I move scoreQuiz from private void calculate() to equal the third constructor value in PassFailQuiz?
arrow_forward
sensors.io code:
lass Sensor {
public:
virtual float read() = 0;
virtual const char* sensorName();
protected:
Sensor(int p) : pin(p) {}
protected:
int pin;
};
class Thermistor : public Sensor {
public:
Thermistor(int);
const char* sensorName();
float read();
};
class LightSensor : public Sensor {
public:
LightSensor(int);
const char* sensorName();
float read();
};
// TODO write the Thermistor and LightSensor constructor, sensorName,
// and read methods
Sensor* sensors[2];
void setup() {
Serial.begin(9600);
sensors[0] = new Thermistor(A3);
sensors[1] = new LightSensor(A0);
}
int sensorno = 0;
void loop() {
// TODO write code to check if data is available from the serial port.
// If the user types in a '0', change the sensorno to 0.
// If the user types in a '1', change the sensorno to 1.
Sensor* mysensor = sensors[sensorno];
Serial.print(mysensor->sensorName());
Serial.print(": ");…
arrow_forward
public class Author2 {3 private String name;4 private int numberOfAwards;5 private boolean guildMember;6 private String[] bestsellers;78 public String Author()9 {10 name = "Grace Random";11 numberOfAwards = 0;12 guildMember = false;13 bestsellers = {"Minority Report", "Ubik", "The Man in the HighCastle"};14 }1516 public void setName(String n)17 {18 name = n;19 }20 public String getName()21 {22 return name;23 }2425 public void winsAPulitzer()26 {27 System.out.println(name + " gave a wonderful speech!");28 }29 }
1. This class includes a constructor that begins on line 8; however, it contains an error. Describe the error.
2. Rewrite the constructor header on line 8 with the error identied in part c of this question corrected.
3. Demonstrate how you might overload the constructor for this class. Write only the header.
4. Write a line of Java code to create an instance (object) of this class. Name it someAuthor.
5. Write a line of code to demonstrate how you would use the someAuthor object…
arrow_forward
class Clock {
public: Clock() : hourValue_(0), minuteValue_(0), secondValue_(0) {}; Clock(const int hourValue, const int minuteValue, const int secondValue) : hourValue_(hourValue), minuteValue_(minuteValue), secondValue_(secondValue) {};
virtual std::string ShowTime(void) = 0; virtual void SetTime(int hourValue, int minuteValue, int secondValue) = 0;
int hourValue_; int minuteValue_; int secondValue_;
int GetHour() { return hourValue_; }; int GetMinute() { return minuteValue_; }; int GetSecond() { return secondValue_; };
void SetHour(const int hourValue) { hourValue_ = hourValue; }; void SetMinute(const int minuteValue) { minuteValue_ = minuteValue; }; void SetSecond(const int secondValue) { secondValue_ = secondValue; };};
class DigitalClock final : public Clock {
public: DigitalClock(const int hourValue, const int minuteValue, const int secondValue);
virtual std::string ShowTime(void) override; virtual void SetTime(const int…
arrow_forward
add override display methodusing System;
//base classclass Book{//data membersprivate string title;private string author;protected double price;//constructorpublic Book(string t, string a){title = t;author = a;price = 500;}//method to display detailspublic void display(){Console.WriteLine("Title:" + title + " author:" + author + " price:" + price);}}
//child classclass PopularBooks : Book {//constructorpublic PopularBooks(string t, string a) :base(t,a) {price = 50000;}}
class Program {static void Main() {//an array of 5 objectsBook[] B = new Book[5];//input title and authorfor(int i=0; i<5;i++){Console.Write("Input title: ");string name = Console.ReadLine();Console.Write("Input author name: ");string author = Console.ReadLine();//if author is popular,//call constructor of sub classif (author.Equals("Khaled Hosseini") || author.Equals("Oscar Wilde") || author.Equals("Rembrandt")){B[i] = new PopularBooks(name, author);}//call base class constructorelse B[i] = new Book(name,…
arrow_forward
Java program
arrow_forward
public class Employee
{
}
private String Name;
private String Surname;
private int Age;
private String EmployeeNum;
public Employee (String Name, String Surname, int Age)
{
this.Name = Name;
this. Surname = Surname;
this. Age Age;
}
public Employee Clone()
{
Employee copy = new Employee (this. Name, this. Surname, this.Age);
return copy;
}
arrow_forward
interface StudentsADT{void admissions();void discharge();void transfers(); }public class Course{String cname;int cno;int credits;public Course(){System.out.println("\nDEFAULT constructor called");}public Course(String c){System.out.println("\noverloaded constructor called");cname=c;}public Course(Course ch){System.out.println("\nCopy constructor called");cname=ch;}void setCourseName(String ch){cname=ch;System.out.println("\n"+cname);}void setSelectionNumber(int cno1){cno=cno1;System.out.println("\n"+cno);}void setNumberOfCredits(int cdit){credits=cdit;System.out.println("\n"+credits);}void setLink(){System.out.println("\nset link");}String getCourseName(){System.out.println("\n"+cname);}int getSelectionNumber(){System.out.println("\n"+cno);}int getNumberOfCredits(){System.out.println("\n"+credits); }void getLink(){System.out.println("\ninside get link");}}
public class Students{String sname;int cno;int credits;int maxno;public Students(){System.out.println("\nDEFAULT constructor…
arrow_forward
Class Phone{
bool wired()
string ageOfInnovation()
}
Class CellPhone extends Phone{
bool wired(){
return false;
}
string ageOfInnovation(){
return "1970's"
}
int thickness(){
return 0;
}
}
Class SmartPhone extends CellPhone{
bool wired(){
return false;
}
string ageOfInnovation(){
return "1990's"
}
string popularlyCalled(){
return "mobile"
}
string mainFeature(){
return "Internet Capability";
}
}
arrow_forward
Java Programming
Please do not change anything in Student class or Course class
class John_Smith extends Student{ public John_Smith() { setFirstName("John"); setLastName("Smith"); setEmail("jsmith@jaguar.tamu.edu"); setGender("Male"); setPhoneNumber("(200)00-0000"); setJNumber("J0101459"); }
class MyCourse extends Course { public MyCourse {
class Course { private String courseNumber; private String courseName; private int creditHrs;
public Course (String number, String name, int creditHrs){ this.courseNumber = number; this.courseName = name; this.creditHrs = creditHrs; }
public String getNumber() { return courseNumber; }
public String getName() { return courseName; }
public int getCreditHrs() { return creditHrs; }
public void setCourseNumber(String courseNumber) { this.courseNumber = courseNumber; }
public void setCourseName(String…
arrow_forward
C#
List the differences between CommissionEmployee class and BasePlusCommissionEmployee class
public class BasePlusCommissionEmployee
{
public string FirstName { get; }
public string LastName { get; }
public string SocialSecurityNumber { get; }
private decimal grossSales;
private decimal commissionRate;
private decimal baseSalary;
public BasePlusCommissionEmployee(string firstName, string lastName,
string socialSecurityNumber, decimal grossSales,
decimal commissionRate, decimal baseSalary)
{
FirstName = firstName;
LastName = lastName;
SocialSecurityNumber = socialSecurityNumber;
GrossSales = grossSales;
CommissionRate = commissionRate;
BaseSalary = baseSalary;
}
public decimal GrossSales
{
get
{
return grossSales;
}
set
{
if (value < 0) // validation
{
throw new ArgumentOutOfRangeException(nameof(value),…
arrow_forward
public class Student
{
private String name;
private String major;
privatedoublegpa;
privateinthoursCompleted;
/**Constructor
* @param name
* The student's name
* @param major
* The student's major
*/
public Student(String name, String major)
{
this.name = name;
this.major = major;
this.gpa = 0.0;
hoursCompleted = 0;
}
/**Constructor
* @param name
* The student's name
* @param major
* The student's major
* @param gpa
* The student's cumulative gpa
* @param hoursCompleted
* Number of credit hours the student has completed
*/
public Student(String name, String major, doublegpa, inthoursCompleted)
{
this.name = name;
this.major = major;
this.gpa = gpa;
this.hoursCompleted = hoursCompleted;
}
/**
* @return The student's major.
*/
public String getMajor()
{
returnmajor;
}
/**
* @param major The major to set.
*/
publicvoid setMajor(String major)
{
this.major = major;
}
/**
* @return The student's name.
*/
public String getName()
{
returnname;
}
/**
* @param name The name to set.
*/…
arrow_forward
object oriented programming using c++
class decleration:
class MyPhoneBook{ string* names; string* phones; int phoneBookSize;
public: MyPhoneBook(int); //Takes size MyPhoneBook(const MyPhoneBook&); //Copy Constructor bool addEntry(string ,string); bool displayEntryAtIndex(int); void displayEntryAtIndices(int*); void displayAll(); int* findByName(string); int* findByPhone(string); bool updateNameAt(string, int); bool updatePhoneAt(string, int); ~MyPhoneBook();};
arrow_forward
public class Pet {
protected String name; protected int age;
public void setName(String userName) { name = userName; }
public String getName() { return name; }
public void setAge(int userAge) { age = userAge; }
public int getAge() { return age; }
public void printInfo() { System.out.println("Pet Information: "); System.out.println(" Name: " + name); System.out.println(" Age: " + age); }
}
import java.util.Scanner;public class PetInformation { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Pet myPet = new Pet(); Cat myCat = new Cat(); String petName, catName, catBreed; int petAge, catAge; petName = scnr.nextLine(); petAge = scnr.nextInt(); scnr.nextLine(); catName = scnr.nextLine(); catAge = scnr.nextInt(); scnr.nextLine(); catBreed = scnr.nextLine(); // TODO: Create generic pet (using petName, petAge) and then…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
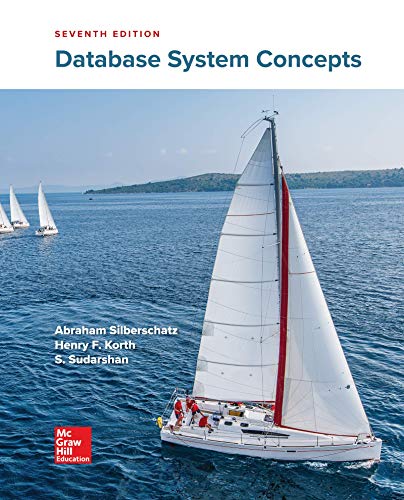
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
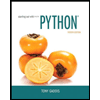
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
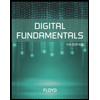
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
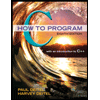
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
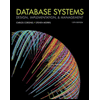
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
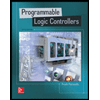
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- public class Aircraft { // instance vairables private String aircraftName; private String regNumber; private String manufacturer; privateintmaxRange; privateintcrewSize; private LocalDate yearPutInService; privateintmaxServiceWeight; privateintnumberOfPassengers; privateintcurrentAirMiles; private LocalDate lastMaintenanceDate; privateintlastMaintenanceMiles; /** * Default Constructor * * @param lastMaintenanceMiles * @param lastMaintenanceDate * @param totalMiles * @param model * @param manufacturer * @param regNumber * @param vehicleType */ public Aircraft(String aircraftName, String regNumber, String manufacturer, intmaxRange, intcrewSize, LocalDate yearPutInService, int maxServiceWeight, int numberOfPassengers, int currentAirMiles, LocalDate lastMaintenanceDate, int lastMaintenanceMiles) { { this.aircraftName = aircraftName; this.regNumber = regNumber; this.manufacturer = manufacturer; this.maxRange = maxRange; this.crewSize = crewSize; this.yearPutInService = yearPutInService;…arrow_forwardclass Player { protected: string name; double weight; double height; public: Player(string n, double w, double h) { name = n; weight = w; height = h; } string getName() const { return name; } virtual void printStats() const = 0; }; class BasketballPlayer : public Player { private: int fieldgoals; int attempts; public: BasketballPlayer(string n, double w, double h, int fg, int a) : Player(n, w, h) { fieldgoals = fg; attempts = a; } void printStats() const { cout << name << endl; cout << "Weight: " << weight; cout << " Height: " << height << endl; cout << "FG: " << fieldgoals; cout << " attempts: " << attempts; cout << " Pct: " << (double) fieldgoals / attempts << endl; } }; a. What does = 0 after function printStats() do? b. Would the following line in main() compile: Player p; -- why or why not? c. Could…arrow_forwardpublic class Plant { protected String plantName; protected String plantCost; public void setPlantName(String userPlantName) { plantName = userPlantName; } public String getPlantName() { return plantName; } public void setPlantCost(String userPlantCost) { plantCost = userPlantCost; } public String getPlantCost() { return plantCost; } public void printInfo() { System.out.println(" Plant name: " + plantName); System.out.println(" Cost: " + plantCost); }} public class Flower extends Plant { private boolean isAnnual; private String colorOfFlowers; public void setPlantType(boolean userIsAnnual) { isAnnual = userIsAnnual; } public boolean getPlantType(){ return isAnnual; } public void setColorOfFlowers(String userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } public String getColorOfFlowers(){ return colorOfFlowers; } @Override public void printInfo(){…arrow_forward
- public class Person { private String personID; private String firstName; private String lastName; private String birthDate; private String address; public Person(){ personID = ""; firstName = ""; lastName = ""; birthDate = ""; address = ""; } public Person(String id, String first, String last, String birth, String add){ setPerson(id,first,last,birth,add); } public void setPerson(String id, String first, String last, String birth, String add){ personID = id; firstName = first; lastName = last; birthDate = birth; address = add; } public String getFirstName(){ return firstName; } public String getLastName(){ return lastName; } public String getBirthdate(){ return birthDate; } public String getAddress(){ return address; } public void print(){ System.out.print("\nPerson ID = " + personID); System.out.print("\nFirst Name = " +firstName); System.out.print("\nLast Name = " +lastName); System.out.print("\nBirth Date = " +birthDate); System.out.print("\nAddress = " +address); } public String…arrow_forwardpublic class Person { private String personID; private String firstName; private String lastName; private String birthDate; private String address; public Person(){ personID = ""; firstName = ""; lastName = ""; birthDate = ""; address = ""; } public Person(String id, String first, String last, String birth, String add){ setPerson(id,first,last,birth,add); } public void setPerson(String id, String first, String last, String birth, String add){ personID = id; firstName = first; lastName = last; birthDate = birth; address = add; } public String getFirstName(){ return firstName; } public String getLastName(){ return lastName; } public String getBirthdate(){ return birthDate; } public String getAddress(){ return address; } public void print(){ System.out.print("\nPerson ID = " + personID); System.out.print("\nFirst Name = " +firstName); System.out.print("\nLast Name = " +lastName); System.out.print("\nBirth Date = " +birthDate); System.out.print("\nAddress = " +address); } public String…arrow_forwardInstrument.java public class Instrument { protectedStringinstrumentName; protectedStringinstrumentManufacturer; protectedintyearBuilt, cost; publicvoidsetName(StringuserName) { instrumentName = userName; } publicStringgetName() { return instrumentName; } publicvoidsetManufacturer(StringuserManufacturer) { instrumentManufacturer = userManufacturer; } publicStringgetManufacturer(){ return instrumentManufacturer; } publicvoidsetYearBuilt(intuserYearBuilt) { yearBuilt = userYearBuilt; } publicintgetYearBuilt() { return yearBuilt; } publicvoidsetCost(intuserCost) { cost = userCost; } publicintgetCost() { return cost; } publicvoidprintInfo() { System.out.println("Instrument Information: "); System.out.println(" Name: " + instrumentName); System.out.println(" Manufacturer: " + instrumentManufacturer); System.out.println(" Year built: " + yearBuilt); System.out.println(" Cost: " + cost); } } StringInstrument.java // TODO: Define a class: StringInstrument that is derived from…arrow_forward
- 2arrow_forwardpublic class Book { protected String title; protected String author; protected String publisher; protected String publicationDate; public void setTitle(String userTitle) { title = userTitle; } public String getTitle() { return title; } public void setAuthor(String userAuthor) { author = userAuthor; } public String getAuthor(){ return author; } public void setPublisher(String userPublisher) { publisher = userPublisher; } public String getPublisher() { return publisher; } public void setPublicationDate(String userPublicationDate) { publicationDate = userPublicationDate; } public String getPublicationDate() { return publicationDate; } public void printInfo() { System.out.println("Book Information: "); System.out.println(" Book Title: " + title); System.out.println(" Author: " + author); System.out.println(" Publisher: " + publisher); System.out.println(" Publication…arrow_forwardclass IndexItem { public: virtual int count() = 0; virtual void display()= 0; };class Book : public IndexItem { private: string title; string author; public: Book(string title, string author): title(title), author(author){} virtual int count(){ return 1; } virtual void display(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };class Category: public IndexItem { private: /* fill in the private member variables for the Category class below */ ? int count; public: Category(string name, string code): name(name), code(code){} /* Implement the count function below. Consider the use of the function as depicted in main() */ ? /* Implement the add function which fills the category with contents below. Consider the use of the function as depicted in main() */ ? virtualvoiddisplay(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };arrow_forward
- class Course { private String courseNumber; private String courseName; private int creditHrs; public Course (String number, String name, int creditHrs){ this.courseNumber = number; this.courseName = name; this.creditHrs = creditHrs; } public String getNumber() { return courseNumber; } public String getName() { return courseName; } public int getCreditHrs() { return creditHrs; } public void setCourseNumber(String courseNumber) { this.courseNumber = courseNumber; } public void setCourseName(String courseName) { this.courseName = courseName; } public void setCreditHrs(int creditHrs) { this.creditHrs = creditHrs; }} class Student { private String firstName; private String lastName; private String gender; private String phoneNumber; private String email; private String jNumber; protected ArrayList<MyCourse> courseList; public String…arrow_forwardclass Course { private String courseNumber; private String courseName; private int creditHrs; public Course (String number, String name, int creditHrs){ this.courseNumber = number; this.courseName = name; this.creditHrs = creditHrs; } public String getNumber() { return courseNumber; } public String getName() { return courseName; } public int getCreditHrs() { return creditHrs; } public void setCourseNumber(String courseNumber) { this.courseNumber = courseNumber; } public void setCourseName(String courseName) { this.courseName = courseName; } public void setCreditHrs(int creditHrs) { this.creditHrs = creditHrs; }}class Student { private String firstName; private String lastName; private String gender; private String phoneNumber; private String email; private String jNumber; protected ArrayList courseList; public String getFullName() {…arrow_forwardC# problem when printing Weigh no value shows up code public class HealthProfile{private String _FirstName;private String _LastName;private int _BirthYear;private double _Height;private double _Weigth;private int _CurrentYear;public HealthProfile(string firstName, string lastName, int birthYear, double height, double weigth, int currentYear){_FirstName = firstName;_LastName = lastName;_BirthYear = birthYear;_Height = height;_Weigth = weight;_CurrentYear = currentYear;}public string firstName { get; set; }public string lastName { get; set; }public int birthYear { get; set; }public int height { get; set; }public double weight { get; set; }public int currentYear { get; set; }public int Person_Age{get { return _CurrentYear - _BirthYear; }}public double Height{get { return _Height; }}public double Weight{get { return Weight; }}public int Max_Heart_Rate{get { return 220 - Person_Age; }}public double Min_Target_Heart_Rate{get { return Max_Heart_Rate * 0.65; }}public double…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
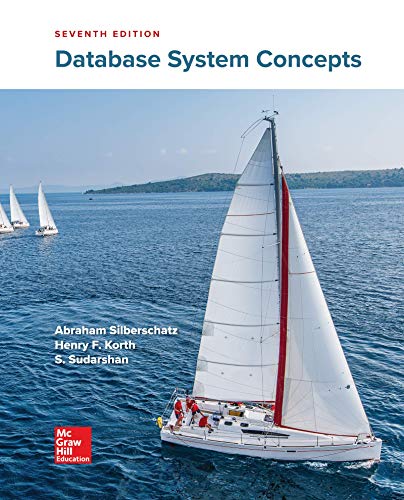
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
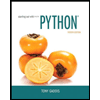
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
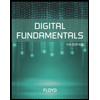
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
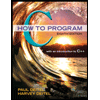
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
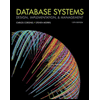
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
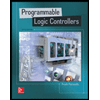
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education