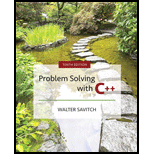
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 18, Problem 7PP
Program Plan Intro
Permutations using “set” class
Program Plan:
- Include required header file.
- Include required “std” namespace.
- Function declaration for display permutations, compute permutations, and display the content of list in set.
- Define main function.
- Call the function “displayPermutations” function.
- Define “displayPermutations” function.
- Declare variable “set1” in “set” template class.
- Declare variable “p” in “set” template class with “list” class of “int” type.
- Fill the set with the first “n” whole numbers.
- Display given statement.
- Initializes a variable “iter” to “0”.
- Display permutation set using for loop.
- Compute the possible set for given set by calling the function “computePermutations”.
- Display the set elements by calling the function “displayLists”.
- Define “computePermutations” function.
- Declare variable “result” in “set<list<int> >”.
- If the number size is equal to “1”, then push the iterator begin value to given list and then insert the list into set “result”.
- Otherwise, recursively call the function “computePermutations”
- Define “displayLists” function.
- Display the content of list using “for” loop.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Doubly Linked List is a data structure that holds a list of items with double links: previous and next. In this homework, you need to implement all functions in the defined ListLinked class (prototype is provided by the instructor). Please download the header file (ListLinked.h) from ecourses, read the comments and complete them using C++. You need to implement all of the ListLinked ADT functions in ListLinked.cpp file, and test them in main.cpp.
//--------------------------------------------------------------------//// Homework 3 ListLinked.h//// Class declaration for the Doubly linked implementation of the List ADT////--------------------------------------------------------------------
#ifndef LISTLINKED_H#define LISTLINKED_H
#include <iostream>
using namespace std;
template <typename DataType>class ListNode { // doubly linked list nodepublic: ListNode(const DataType& nodeData, ListNode* nextPtr, ListNode* prevPtr);…
Program needs to be in C++
The program will have a person, student, and faculty class. Use an STL for a list rather than using the "list" class. Populate an STL structure with a series of objects (at least 5 combined). Create a list of POINTERS to objects, so you can use polymorphism. The beginning of the list should be all faculty (add to the head), and the end of the list (add at the tail) should be students/interns. It is not necessary to add the base class objects. Utilize the STL functions to apply: add student/intern/faculty, remove a specific entry from a user input of a name, search for name, print all, and delete all. The menu items will be in main and call the STL functions.
Please use the code below and make the appropriate edits
#include <iostream>#include <bits/stdc++.h>using namespace std;
struct Date { int month, year;};
class Person {protected: string name, department;public: Person() { name = ""; department = ""; } Person(string…
Read this: Complete the code in Visual Studio using C++ Programming Language
- Give me the answer in Visual Studio so I can copy***
- Separate the files such as .cpp, .h, or .main if any!
Develop a C++ "doubly" linked list class of your own that can hold a series of signed shorts
Develop the following functionality:
Develop a linked list node struct/class
You can use it as a subclass like in the book (Class contained inside a class)
You can use it as its own separate class
Your choice
Maintain a private pointer to a node class pointer (head)
Constructor
Initialize head pointer to null
Destructor
Make sure to properly delete every node in your linked list
push_front(value)
Insert the value at the front of the linked list
pop_front()
Remove the node at the front of the linked list
If empty, this is a no operation
operator <<
Display the contents of the linked list just like you would print a character string
operator []
Treat like…
Chapter 18 Solutions
Problem Solving with C++ (10th Edition)
Ch. 18.1 - If v is a vector, what does v.begin() return? What...Ch. 18.1 - If p is an iterator for a vector object v, what is...Ch. 18.1 - Suppose v is a vector of ints. Write a for loop...Ch. 18.1 - Suppose the vector v contains the letters 'A',...Ch. 18.1 - Suppose the vector v contains the letters 'A',...Ch. 18.1 - Suppose you want to run the following code, where...Ch. 18.2 - Prob. 7STECh. 18.2 - Prob. 8STECh. 18.2 - Prob. 9STECh. 18.2 - Prob. 10STE
Ch. 18.2 - Prob. 11STECh. 18.2 - Prob. 12STECh. 18.2 - Prob. 13STECh. 18.2 - Prob. 14STECh. 18.2 - Prob. 15STECh. 18.2 - Prob. 16STECh. 18.3 - Prob. 17STECh. 18.3 - Prob. 18STECh. 18.3 - Prob. 19STECh. 18.3 - Suppose v is an object of the class vectorint. Use...Ch. 18.3 - Prob. 21STECh. 18.3 - Can you use the copy template function with vector...Ch. 18.3 - Prob. 23STECh. 18 - Prob. 1PCh. 18 - Prob. 2PCh. 18 - Prob. 3PCh. 18 - Prob. 4PCh. 18 - Write a program that allows the user to enter any...Ch. 18 - Prob. 3PPCh. 18 - Prob. 5PPCh. 18 - Solution to Programming Project 18.6 In this...Ch. 18 - Prob. 7PPCh. 18 - You have collected a file of movie ratings where...Ch. 18 - Prob. 9PPCh. 18 - Prob. 11PPCh. 18 - Write a program that uses regular expressions to...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Develop a *Typing* *Tutor* project game in C/C++ language using Linked list. In this game user should enter the word which is required before the required time and this also displays the correct word and mistaken words separately.arrow_forwardIn C++ data structure Please write program per instructions. Thank you A set is a collection of distinct elements of the same type. Design the class unorderedSetType, derived from the unorderedArrayListType, to manipulate sets. Note that you need to redefine only the f unctions insertAt, insertEnd, and replaceAt. If the item to be inserted is already in the list, the functions insertAt and insertEnd output an appropriate message. Similarly, if the item to be replaced is already in the list, the function replaceAt outputs and appropriate message. Also, write a program to test your class: then Redo the program using templatesarrow_forwardfor c++ please thank you 4, List search Modify the linked listv class you created in the previous programming challange to include a member function named search that returns the position of a specific value, x, in the lined list. the first node in the list is at position 0, the second node is at position 1, and so on. if x is not found on the list, the search should return -1 test the new member function using an approprate driver program. here is my previous programming challange. #include <iostream> using namespace std; struct node { int data; node *next; }; class list { private: node *head,*tail; public: list() { head = NULL; tail = NULL; } ~list() { } void append() { int value; cout<<"Enter the value to append: "; cin>>value; node *temp=new node; node *s; temp->data = value; temp->next = NULL; s = head; if(head==NULL) head=temp; else { while (s->next != NULL) s = s->next; s->next = temp; } } void insert() { int value,pos,i,count=0;…arrow_forward
- emacs/lisp function Write a function in Lisp that takes one parameter which we can assume to be a list. The function should print all the elements of the list using the function princ and a space between them. Use the function mapc for this purpose.Show a few examples of testing this function. Show one example using the function apply and one using the function funcall.arrow_forwardC++ PROGRAMMINGTopic: HashTable - PolyHash Quadratic Explain the c++ code below.: SEE ATTACHED PHOTO FOR THE PROBLEM INSTRUCTIONS It doesn't have to be long, as long as you explain what the important parts of the code do. (The code is already implemented and correct, only the explanation needed). You can also explain line by line for an upvote, thanks. EXPLAIN THE CODE BELOW: #include <cstdlib> #include <math.h> #include <cstring> #include <iostream> using namespace std; class HashTable { string* table; int N; int count; // TODO: Polynomial Hash Code using a=7 int hash_code(string key) { int code; int hash = 0; for (int i = 0; i < key.size(); i++) { char ch = key[i]; code += ((ch - 96) * pow(7, key.size() - (i + 1))); } return code; } // TODO: This hash table uses a MAD compression function // where a = 11, b = 461, p = 919 int compress(int code) { return (((11*code)+461) % 919) % N; }…arrow_forwardThis is a free form programming assignment where you are: Required to create a simple phone book application Asked to make a choice of appropriate data structure and implementation. Defend your selection and explain the efficiency of your program using Big(O) notation.You do not need to create data structures from scratch. You are free to use datastructures available in the standard template C++ library. For example std::stack<int> orstd::set<int> , std::maps<int,int>, etc. You have familiarity with these from your CodeStep by Step labs. You are not permitted to use simple arrays. SpecificationsCreate a phone book program that stores and manages contact information.Contact information is name and corresponding phone number. The program reads thisinformation for multiple contacts from a data file “contacts.txt” and saves them in asuitable data structure. Once the data is stored, it allows the user to display all contacts,add a single contact, remove a contact,…arrow_forward
- Read this: Complete the code in Visual Studio using C++ Programming Language with 1 file or clear answer!! - Give me the answer in Visual Studio so I can copy*** - Separate the files such as .cpp, .h, or .main if any! Develop a C++ "doubly" linked list class of your own that can hold a series of signed shorts Develop the following functionality: Develop a linked list node struct/class You can use it as a subclass like in the book (Class contained inside a class) You can use it as its own separate class Your choice Maintain a private pointer to a node class pointer (head) Constructor Initialize head pointer to null Destructor Make sure to properly delete every node in your linked list push_front(value) Insert the value at the front of the linked list pop_front() Remove the node at the front of the linked list If empty, this is a no operation operator << Display the contents of the linked list just like you would print a character…arrow_forwardPlease just use main.cpp,sequence.cpp and sequence.h. It has provided the sample insert()function. No documentation (commenting) is required for this assignment. This assignment is based on an assignment from "Data Structures and Other Objects Using C++" by Michael Main and Walter Savitch. Use linked lists to implement a Sequence class that stores int values. Specification The specification of the class is below. There are 9 member functions (not counting the big 3) and 2 types to define. The idea behind this class is that there will be an internal iterator, i.e., an iterator that the client cannot access, but that the class itself manages. For example, if we have a Sequence object named s, we would use s.start() to set the iterator to the beginning of the list, and s.advance() to move the iterator to the next node in the list. (I'm making the analogy to iterators as a way to help you understand the point of what we are doing. If trying to think in terms of iterators is confusing,…arrow_forwardin Haskell: Write a function diffList with two lists as parameters that deletes every occurrence of every element of the second list from the first list. This is analogous to a set difference.arrow_forward
- Programming in C: Challenge 1: Create and Print a Doubly Linked List In class, I briefly mentioned how to create a doubly linked list (a linked list with two directions: forward and backwards). This task challenges you to create a doubly linked list of 5 nodes. If your nodes were called n1, n2, n3, n4 and n5 (which they shouldn’t, please give them original names), your list should be linked as follows: We discussed what "start" and "end" should be. Then, also in main, you will have to print the list forwards by taking advantage of the arrows pointing to the right. You will also have to print it backwards taking advantage of the arrows pointing to the left. Again, everything must be done in main. Hint: look at the way in which I printed the singly linked list in class. In other words, if your nodes had the values: n1=1, n2=2, n3=3, n4=4 and n5=5; your program should print:1 3 5 2 4 (forwards) and 4 2 5 3 1 (backwards). Please give different values to your nodes, they should not have…arrow_forwardWrite a COMPLETE C++ code by using only #include library, Includes an explanation of the code and its working details completely. It must contain the pictures of each and every functions results. Write a menu driven program to implement the linked list that has the following operations: Insert(data) Search(key) Delete(key) Your Linked List must accommodate Trader type of data. A bloom filter that acts as a membership data structure giving a very quick heads up on search of an item. Data is key/value fields for a Trader type of data tradernumber is the key or identifying field and other data fields name and department are value fields. All these fields combine to form the data and for Search and Delete all we need from the user is the key field.arrow_forwardExercise, maxCylinderVolume F# system function such as min or methods in the list module such as List.map are not allowed Write a function maxCylinderVolume that takes a list of floating-point tuples that represent dimensions of a cylinder and returns the volume of the cylinder that has the largest volume. Each tuple has two floating point values that are both greater than zero. The first value is the radius r and the second value is the height h. The volume of the cylinder is computed using ??2h. The value π is represented in F# with System.Math.PI. If the list is empty, return 0.0. Examples: > maxCylinderVolume [(2.1, 3.4); (4.7, 2.8); (0.9, 6.1); (3.2, 5.4)];;val it : float = 194.3137888> maxCylinderVolume [(0.33, 0.66)];;val it : float = 0.2257988304arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
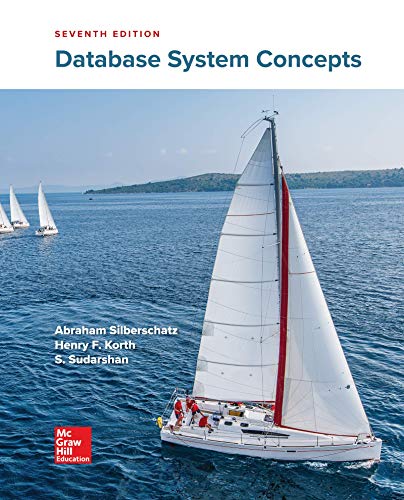
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
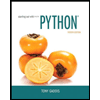
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
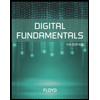
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
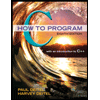
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
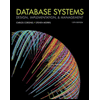
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
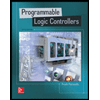
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education