What is a predicate?
A predicate is a function defined with set of variables, which returns the result True or False. The output of a predicate is a Boolean value as a response when given a set of parameters:
boolean predicate(set of parameters)
True or false is the value of a Boolean (yes or no). Any set of arguments can be used as the function's input. For example, a numerical inquiry, such as "Is the temperature over 15 degrees?" can be written as follows:
temperature > 15
If the temperature is 30, for example, the response is yes. If the temperature is 10, the answer is false.
If the predicate must be constructed as a Java function, then it would look like this:
boolean checkTemp(int tem) { return tem > 15;}
Boolean Values and Boolean Expressions
A Boolean data type exists in every programming language, as well as a mechanism to describe Boolean operations such as true and false.
Programming Language | Type of Data | True | False | Example Expression |
Python | <class 'bool'> | True | False | (x>3) and (3==9) |
C | bool | true | false | x>3 && 3==9 |
C++ | bool | true | false | x>3 && 3==9 |
Java | boolean | true | false | x>3 && 3==9 |
Fortran | logical | .true. | .false. | (x.GT.3).AND.(3.EQ.9) |
Algol | BOOLEAN | TRUE | FALSE | X GTR 3 AND 3 EQL 9 |
An expression that gives to a Boolean value is known as a Boolean expression. The equality operator == compares two values and gives result either true or false. If LHS is equal to RHS, then it gives true. Otherwise, it gives false. Thus, the output contains only two values namely true and false.
For Example-
print(2 == 2) # 2 is equal to 2 print(3 == 8) # 3 is not equal to 8j = "Su"print(j + "prabhat" == "Suprabhat") # j+prabhat = Suprabhat which is equal to Suprabhat#OUTPUT--->truefalsetrue
Because the two operands in the first statement are equal, the expression evaluates to true. Since 3 is not equal 8, the second assertion returns false.
No Explicit Boolean Value
There is no explicit Boolean data type in some languages, such as Structure Query Language (SQL), Perl, LISP, etc. Though not specifically mentioned, these languages employ a bit, byte, digit according to the Boolean data type. In summary, zero is false, whereas the rest of the equation is correct.
The bool data type, for example, is derived from the _BOOL data type in the C programming language. The usage of bool was thought to be more user-friendly.
The unsigned int is the foundation of the _BOOL data type. The variable Y is declared as Boolean type and different values are assigned in the following code:
_BOOL Y;Y = 7 /* Y gets assigned 7 */Y = 67 /* Y gets assigned 67 */Y = 122 /* Y gets assigned 122 */Y = "Hello" /* Y gets assigned Hello */
When a number other than zero is assigned to Y, then it takes on the value of one.
Predicates in Programming
A function from arbitrary variables to a single Boolean value, as in normal predicate logic; or, equivalently:
- A defining function for a set of tuples with a unified schema.
- A relation, in the sense of relational algebra, is a collection of such tuples.
A predicate in logic programming differs from a Boolean-valued function in any other programming language. In predicate that it takes those equivalences seriously, to the point where the language runtime can treat any argument as either input or output. It is searching through the actual concrete set of tuples or dynamically computing tuples that satisfy the characteristic function to find those that match whatever values are chosen to treat as inputs.
Controlling Flow in Imperative Programs
The IF statement is a frequent decision statement in imperative programming languages such as to name a few, there are Python, C, and Java programming languages. It states that "If a set of operations is true, then it gives result as yes; Otherwise, it gives the result as no (false)".
For example, in a C++ coding:
if (z > 16) { /* The condition is true, so run if block*/} else { /* The condition is false, so run else block */}
The predicate, in this case, is the condition z>16. In this code, z is a variable, and the 'if' statement checks 'the assigned value of z is greater than 16 or not.' If the value of z is greater than 16, then it gives result "true". Otherwise, it gives a result "false". The overall statements execute based on a Boolean value.
In C++ code:
boolean checkInteger(int a) { return a > 15;}
Implementation of predicate in Object-Oriented Programming(Sorting and Filtering)
A predicate is also used in object-oriented programming. This logic is mentioned in the definition of the class and required to create the methods also, such as "isLessThan." Not only would the sorting or filtering algorithm have to deal with a set of objects, but it would also have to deal with the class that is used to implement the predicate.
class Predicateclass { abstract boolean isLessThan(Object o1, Object o2);}
The sorting algorithm would then perform its function using this abstract class defined in their declaration. In the above code, the class Predicateclass is defined and the class has a function isLessThan() with two parameters.
Take another example, let's create a FilterSetObject class and the class has a method addFilteredSet. This function adds the filtered object to the filteredSet:
class FilterSetObject { void addFilteredSet (Set setOfObjects, Set filteredSet, Predicateclass predicate) { for (Object obj: setOfObjects) { if(predicate.isLessThan(obj)) { filteredSet.add(obj); } } }}
Sorting Algorithms using Predicates
The main task of the sorting algorithm is to find out how a sorting algorithm is determining the relationship between two objects. It can seem that no matter what sorting method is used, all algorithms must discover the link between the objects. The predicate's job is to establish this connection.
It frequently has to decide if one object is "less-than" another:
isLessThan(obj1,obj2) := true if obj1 is less than obj2
For example, in the bird example, it may say:
isLessThan(bird1,bird2) := true if bird1 weights less than bird2
This could lead to a list of birds ranging in size from the higher weight to lower weight. To arrange the birds in order of size from largest to smallest, it can be done easily by:
isLessThan(bird1,bird2) := true if bird1 height more than bird2
The whole computation is done using the “isLessThan” predicate. The predicate in the prior example representation would be:
isLessThan(bird1,bird2) := birdDescription(bird1, 1) > birdDescription(bird2, 2)
Predicates in Fuzzy Logic
When a programmers are essentially asking a yes/no question, they should be aware that the response isn't always true or false. Perhaps they are confused, or perhaps they are in the midst. For example, if to make a predicate about temperature.
Question: Is the water warm?
Predicate: IsItWarm(T)
The answer is so confusing because if the water is boiling 100 degrees Celsius it gives the output “yes”. But if the water is freezing at 4 degrees Celsius, then it gives a negative response.
To understand it in another scenario if the water is boiled and after the boiling of water, put it in the snow. Then what happens? If the water is warm, then the predicate answers “yes”. And after some hour, if water is not warm or cool, then the predicate answers “no”.
But the question has arisen that at which temperature the predicate is switched its answer? Was it forty-five, thirty-three, or twenty? Do believe, for example, that water is hot at 31 degrees and then becomes cold at 30 degrees? It is known that the water cools, then turns lukewarm before becoming cold. It creates a "not sure which" zone.
To make matters even more confusing, Steven from Norway might define "hot" differently than Giovanni from Italy.
So, it is the concept of fuzzy logic. It creates a smooth movement from true to false.
Example of fuzzy logic predicate
Consider defining a predicate IsItHot(T). Let’s see the diagram. The diagram answers the question "Is it hot?" If IsItHot(T)=1.0, the output is "yes," while if IsItHot(T)=0.0, it gives a result “no.”
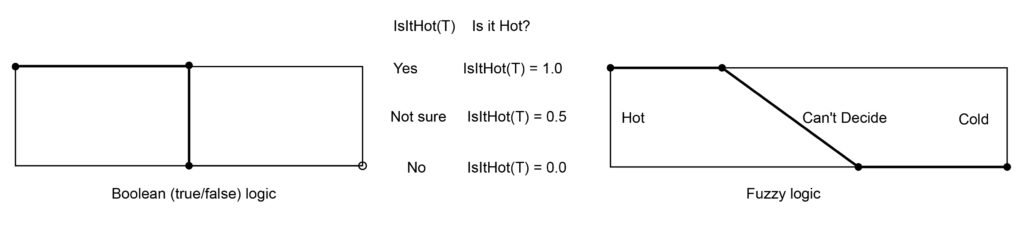
It can detect a dramatic transition between hot and cold in standard Boolean (true/false) reasoning. Assume that, this isn't the case. The fuzzy logic predicate, on the other hand, has a delayed transition from hot to cold. The water is hotter when the answer is near 1.0, and colder when the answer is close to 0.0. If the value is 0.5, it has no way of knowing.
Common Mistakes
The predicate notion was used in program flow, filtering, and sorting. In other words, the concept of a predicate is utilized whenever a question or a judgment needs to be made. Applying fuzzy logic to the boolean idea is also a concept of the predicate. This is where we acknowledge that we can't always make black-and-white decisions. There is a grey region from time to time.
Context and Applications
This is an important topic in the professional test and for a variety of courses, particularly for-
- A Prolog Programming Courses
- AI & ML series
Related Concepts
- Predicate Function
- Naming convention of predicate
- Unary predicate
- Binary predicate
Practice Problems
Q1- Predicate gives which type of value?
- true
- false
- both
- none
Correct answer- both
Explanation- A predicate is a type of function. The output of a predicate is a Boolean value (true or false) as a response.
Q2- what is the value of this code?
#include<iostream>using namespace std;boolean checkInteger(int a){return (a == 4);}int main (){int b = checkInteger(5);cout<<b;return 0;}
- 1
- 6
- 3
- 0
Correct Answer- 0
Explanation- In the main function, the check function, the function passes the parameter 5 which is not equal to 3. so the function returns the 0 value.
Q3- What is the meaning of fuzzy?
- lacking in clarity
- Clear
- Ambiguous
- None
Correct Answer- lacking in clarity
Explanation- If something is not clear that means it is fuzzy.
Q4- Which of the following is the sample expression of Fortran?
- (x.GT.3).AND.(3.EQ.9)
- x >6 and 3==9
- x > 6 && 3==9
- None
Correct answer- (x.GT.3).AND.(3.EQ.9)
Explanation- (x.GT.3).AND.(3.EQ.9) is correct syntax in Fortran.
Q5- Which of the following is the sample expression of Algol?
- (x.GT.3).AND.(3.EQ.9)
- x >6 and 3==9
- x > 6 && 3==9
- X GTR 3 AND 3 EQL 9
Correct answer- X GTR 3 AND 3 EQL 9
Explanation- X GTR 3 AND 3 EQL 9 is the correct syntax in Algol.
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Predicates Homework Questions from Fellow Students
Browse our recently answered Predicates homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.