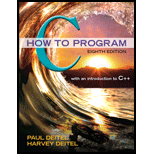
(Bucket Sort) A bucket sort begins with a one-dimensional array of positive integers to be sorted, and a two-dimensional array of integers with rows subscripted from 0 to 9 and columns subscripted from 0 to
The
- Loop through the one-dimensional array and place each of its values in a row of the bucket array based on its ones digit. For example, 97 is placed in row 7, 3 is placed in row 3 and 100 is placed in row 0.
- Loop through the bucket array and copy the values back to the original array. The new order of the above values in the one-dimensional array is 100, 3 and 97.
- Repeat this process for each subsequent digit position (tens, hundreds, thousands, and so on) and stop when the leftmost digit of the largest number has been processed.
On the second pass of the array, 100 is placed in row 0, 3 is placed in row 0 (it had only one digit so we treat it as 03) and 97 is placed in row 9. The order of the values in the one-dimensional array is 100, 3 and 97. On the third pass, 100 is placed in row 1, 3 (003) is placed in row zero and 97 (097) is placed in row zero (after 3). The bucket sort is guaranteed to have all the values property sorted after processing the leftmost digit of the largest number. The bucket sort knows it’s done when all the values are copied into row zero of the two-dimensional array. The two-dimensional array of buckets is ten times the size of the integer array being sorted. This sorting technique provides far better performance than a bubble sort but requires much larger storage capacity. Bubble sort requires only one additional memory location for the type of data being sorted. Bucket sort is an example of a space—time trade-off. It uses more memory but performs better. This version of the bucket sort requires copying all the data back to the original array on each pass. Another possibility is to create a second two-dimensional bucket array and repeatedly move the data between the two bucket arrays until all the data is copied into row zero of one of the arrays. Row zero then contains the sorted array.

Want to see the full answer?
Check out a sample textbook solution
Chapter D Solutions
C How to Program (8th Edition)
Additional Engineering Textbook Solutions
Starting out with Visual C# (4th Edition)
C Programming Language
Database Concepts (8th Edition)
Differential Equations: Computing and Modeling (5th Edition), Edwards, Penney & Calvis
Java: An Introduction to Problem Solving and Programming (8th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
- In statistics, the mode of a set of values is the value that occurs most often or with the greatest frequency. Write a function that accepts as arguments the following: A) an array of integers B) An integer that indicates the number of elements in the array and returns a vector of integers C) Do not sort the array The function should determine the mode of the array or modes. That is, it should determine which value or values in the array occurs most often. The mode is the value or values the function should return. If the array has no mode (none of the values occur more than once), the function should return an empty vector. (assume the array will always contain nonnegative values). Write functions that fill the array with random numbers Test your function thoroughly. Criteria 1) program compiles2) program solves problem according to specification3) program declares, creates, or initializes static or dynamic array correctly4) if required program defines function or functions with…arrow_forwardC++ Programming. OVERVIEW OF DATA STRUCTURES. Task: Write a program to process a data array. Execute a custom task as a separate function or method of a custom class. When performing a task, use functional methods for manually entering array elements, generating random numbers, and printing array elements. Given an array of size N. Create a function to determine the element that is furthest from the arithmetic mean of the array elements (that is, the maximum absolute difference).arrow_forward1arrow_forward
- Hello, I am having trouble with this homework. Random modification of all elements in an integer array.a) Initialize an integer array of capacity 5.b) Implement an array print function.c) Print the array to the console.d) Implement an offset function that accepts an integer array as inputand randomly modifies each element by up to +/- 5.e) Use the offset function to update the array.f) Print the modified array. Example Output (input in bold italics) Original: 5 1 4 9 2Offset: 7 -2 6 11 4arrow_forwardc) Write a version of enqueue that checks for a full array. If the array is full, the function simply returns false. If the array is not full, the behaviour is the same as the original enqueue, except that the function also returns true.arrow_forward"""3. Write a function validSolution/ValidateSolution/valid_solution()that accepts a 2D array representing a Sudoku board, and returns trueif it is a valid solution, or false otherwise. The cells of the sudokuboard may also contain 0's, which will represent empty cells.Boards containing one or more zeroes are considered to be invalid solutions.The board is always 9 cells by 9 cells, and every cell only contains integersfrom 0 to 9. (More info at: http://en.wikipedia.org/wiki/Sudoku)""" # Using dict/hash-tablefrom collections import defaultdict def valid_solution_hashtable(board): for i in range(len(board)): dict_row = defaultdict(int) dict_col = defaultdict(int) for j in range(len(board[0])): value_row = board[i][j] value_col = board[j][i] if not value_row or value_col == 0: return False if value_row in dict_row: return False else: dict_row[value_row] += 1.arrow_forward
- 9. Implement an array with values 1, 5, 14, 23, 45, 52, 58, 81, 82 91. a) Create a getindex( function which does a linear search upon the array for a specific value n. Return the index of n, or -1 ifn does not exist, b) Print the array. c) Search the array for the values 23, 58, 11, rint the reaults. Qutpur Examnle 15 14 23 45 52 58 71 82 91 Number 23 is located at index Number 58 As located at index C Number 11 i looated at index -1arrow_forwardNB: Square bracket notation for array access is NOT allowed for Question 3, i.e. array[i]. Array access MUST be facilitated by the use of pointers. Write a function, ReadFile(), that reads the content of the provided text file “Message.txt” and stores it in an array. The function receives as parameter a pointer to a character array where the content of the text file should be stored.. The number of lines that was read from the text file must be returned to the calling statement. Write a function, ReverseMyString(), that converts the character array (which now contains the contents of the text file) by replacing the first character with the last character, the second character replaced with the second last character, etc, until the message is reversed as shown in the Figure 3. The function receives as parameters, a pointer to the character array and an integer value indicating the length of the array. The function returns a 1 or 0 to indicate success and failure respectively. NB: You…arrow_forward1)Please do it in C++ languagearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
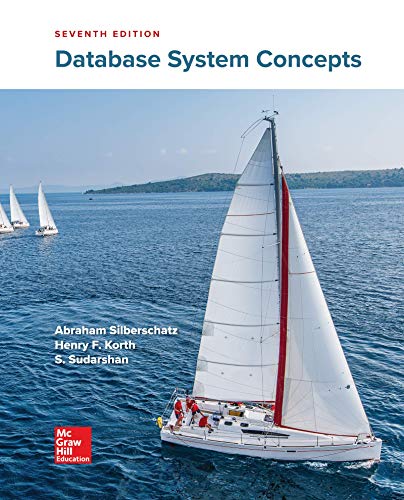
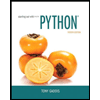
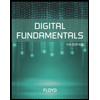
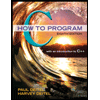
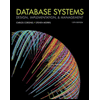
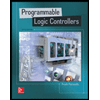