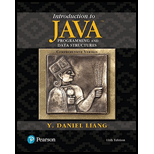
(The Account class) Design a class named Account that contains:
- A private int data field named id for the account (default 0).
- A private double data field named balance for the account (default 0).
- A private double data field named annual InterestRate that stores the current interest rate (default 0). Assume that all accounts have the same interest rate.
- A private Date data field named dateCreated that stores the date when the account was created.
- A no-arg constructor that creates a default account.
- A constructor that creates an account with the specified id and initial balance.
- The accessor and mutator methods for id, balance, and annual InterestRate.
- The accessor method for dateCreated.
- A method named getMonthlyInterestRate () that returns the monthly interest rate.
- A method named getMonthlyInterest () that returns the monthly interest.
- A method named withdraw that withdraws a specified amount from the account.
- A method named deposit that deposits a specified amount to the account.
Draw the UML diagram for the class then implement the class. (Hint: The method getMonthlyInterest () is to return monthly interest, not the i nterest rate.
Monthly interest is balance * monthlyInterestRate. monthlyInterestRate is annual InterestRate /12. Note annual InterestRate is a percentage, for example 4.5%. You need to divide it by 100.)
Write a test

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Starting Out With Visual Basic (7th Edition)
Starting Out with C++: Early Objects
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Starting Out with Programming Logic and Design (4th Edition)
C How to Program (8th Edition)
- 1. (The Fan class) Design a class named Fan to represent a fan. The class contains: The Fan class Three constants named SLOW, MEDIUM, and FAST with the values 1, 2, and 3 to denote the fan speed. A private int data field named speed that specifies the speed of the fan (the default is SLOW). A private boolean data field named on that specifies whether the fan is on (the default is false). A private double data field named radius that specifies the radius of the fan (the default is 5). A string data field named color that specifies the color of the fan (the default is blue). Accessor and mutator methods for all four data fields. A no-arg Constructor that creates a default Fan. A method named toString() that returns a string description for the fan.arrow_forwardReadme.md: Stars(C++) This lab exercise will practice creating objects with constructors and destructors and demonstrate when constructors and destructors are called. Star Class Create a class, Star. A Star object has two member variables: its name, and a solar radius. This class should have a constructor which takes a std::string, the name of the star, and a double, the solar radius of the star. In the constructor, the Star class should print to the terminal that the star was born. For example, if you create a Star as follows: Star my_star("Saiph", 22.2); Then the constructor should print: The star Saiph was born. In the destructor, the Star class should print to the terminal that the star was destroyed, along with the number of times the volume of the sun that that star was, formatted to two decimal places. Hint: use the following line to set the precision to 2 decimal places: std::cout << std::fixed << std::setprecision(2); For example, when my_star above has its…arrow_forward5 (The Time class) Design a class named Time. The class contains: ■ Data fields hour, minute, and second that represent a time. ■ A no-arg constructor that creates a Time object for the current time. ■ A constructor that constructs a Time object with a specified elapse time since the middle of night, Jan 1, 1970, in seconds. ■ A constructor that constructs a Time object with the specified hour, minute, and second. ■ Three get functions for the data fields hour, minute, and second. ■ A function named setTime(int elapseTime) that sets a new time for the object using the elapsed time. Draw the UML diagram for the class. Implement the class. Write a test program that creates two Time objects, one using a no-arg constructor and the other using Time (555550), and display their hour, minute, and second. (Hint: The first two constructors will extract hour, minute, and second from the elapse time. For example, if the elapse time is 555550 seconds, the hour is 10, the minute is 19, and the…arrow_forward
- (Java) The Abstract Art Class Write an abstract class as follows: The class is named Art It inherits from the Comparable interface It has a private String member variable named name It has a private String member variable named artist It has a private int member variable called year It has a default constructor that assigns the values "No name" to name, "No artist" to artist and -1 to the year. This default constructor calls the three argument constructor. It has a three-argument constructor to assign values to the name, artist and year variables. It has a copy constructor that makes a copy of another non-null Art object It has getters and setters for the name, artist and year variables It has a toString() method that creates a string of artist, with name and year tabbed once on subsequent lines (see sample output) It has an equals method that compares this Art to another Object It has a compareTo method that compares in this order: 1) artist, 2) name, 3) year This class contains no…arrow_forward(Person Class) Design a class named Person that contains: o name, gender, and personCase as a private attribute o Non-default constructor that specifies name and gender o toString method that returns person data o personCase () method with no implementation (Student Class) Design a class named Student which is a child of Person that contains: o studentID as a private attribute o Nondefault constructor that specifies the name, gender, and studentID o toString method that returns student data o Implement personCase () that assigns "Not Studying" to personCase if studentID equals 0, “Studying" in case studentID greater than 0, and "Not a student" in case studentID less than 0. (Employee Class) Design a class named Employee which is a child of Person that contains: o employeelD as a private attribute o Non-default constructor that specifies the name, gender, and employeelD o toString method that returns employee data o Implement personCase () that assign “Technical" to personCase if…arrow_forwardMark the following statements as true or false. The member variables of a class must be of the same type. (1) The member functions of a class must be public. (2) A class can have more than one constructor. (5) A class can have more than one destructor. (5) Both constructors and destructors can have parameters. (5)arrow_forward
- (java) The Painting Subclass Write class as follows: The class is named Painting, and it inherits from the Art class. It has a private String member variable named medium It has a default constructor that assigns the values "No name" to name, "No artist" to artist, -1 to year, and "No medium" to the medium variable. This default constructor calls the four-argument constructor. It has a four-argument constructor to assign values to the name, artist, year, and medium variables. It has a getter and settersfor the medium variable. It has a toString() method This class contains no other methods Make sure to include your name, the name of this class, our course number, and the Activity number in a Javadoc comment at the top. Make sure to write a Javadoc comment for each of these methods.arrow_forwardPlease help with the following: C# .NET change the main class so that the user is the one that as to put the name Write a driver class (app) that prompts for the person’s data input, instantiates an object of class HeartRates and displays the patient’s information from that object by calling the DisplayPatientRecord, method. MAIN CLASS---------------------- static void Main(string[] args) { // instance of patient record with each of the 4 parameters taking in a value HeartRates heartRate = new HeartRates("James", "Kill", 1988, 2021); heartRate.DisplayPatientRecord(); // call the method to display The Patient Record } CLASS HeartRATES------------------- class HeartRates { //class attributes private private string _First_Name; private string _Last_Name; private int _Birth_Year; private int _Current_Year; // Constructor which receives private parameters to initialize variables public HeartRates(string First_Name, string Last_Name, int Birth_Year, int Current_Year) { _First_Name =…arrow_forwardEmployee Class (Medium) Write a class named Employee that has the following member variables: name. A string that holds the employee’s name. idNumber. An int variable that holds the employee’s ID number. department. A string that holds the name of the department where the employee works. position. A string that holds the employee’s job title. The class should have the following constructors: A constructor that accepts the following values as arguments and assigns them to the appropriate member variables: employee’s name, employee’s ID number, department, and position. A constructor that accepts the following values as arguments and assigns them to the appropriate member variables: employee’s name and ID number. The department and position fields should be assigned an empty string (""). A default constructor that assigns empty strings ("") to the name, department, and position member variables, and 0 to the idNumber member variable. Write appropriate mutator functions that…arrow_forward
- Design a new class that contains a print and read function by creating a new object and calling the print and reading functions inside the case ( data structure in java)arrow_forward(Java) The Sculpture Subclass Write class as follows: The class is named Sculpture, and it inherits from the Painting class. It has a private boolean member variable named humanForm It has a default constructor that assigns the values "No name" to name, "No artist" to artist, -1 to the year, "No medium" to the medium, and false to the humanForm variable. This default constructor calls the five argument constructor. It has a five-argument constructor to assign values to the name, artist, year, medium, and humanForm variables. It has a getter and setter for the humanForm variable. It has a toString() method. This class contains no other methods Make sure to include your name, the name of this class, our course number, and the Activity number in a Javadoc comment at the top. Make sure to write a Javadoc comment for each of these methods.arrow_forwardUse Python Programming Language (The Stock class) Design a class named Stock to represent a company’s stock that contains: A private string data field named symbol for the stock’s symbol. A private string data field named name for the stock’s name. A private float data field named previousClosingPrice that stores the stock price for the previous day. A private float data field named currentPrice that stores the stock price for the current time. A constructor that creates a stock with the specified symbol, name, previous price, and current price. A get method for returning the stock name. A get method for returning the stock symbol. Get and set methods for getting/setting the stock’s previous price. Get and set methods for getting/setting the stock’s current price. A method named getChangePercent() that returns the percentage changed from previousClosingPrice to currentPrice. Write a test program that creates a Stock object with the stock symbol INTC, the name Inte lCorporation, the…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
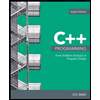