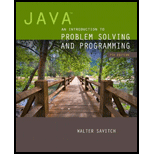
Concept explainers
Explanation of Solution
Modified code:
“Account.java”:
//Class Account
public class Account
{
//Private variable
private double balance;
//Define a constructor
public Account()
{
//Initialize the amount value
balance = 0;
}
//Parameterized constructor
public Account(double initialDeposit)
{
//Assign the value
balance = initialDeposit;
}
//Function to get balance
public double getBalance()
{
//Return the amount
return balance;
}
//Function to deposit the amount
public double deposit(double amount) throws NegativeAmountException
{
//Check if amount is greater than 0
if (amount > 0)
//Add the value to the balance
balance += amount;
//Else
Else
//return -1;
//Throw an exception
throw new NegativeAmountException();
//Return the balance
return balance;
}
//Function definition to withdraw the amount
public double withdraw(double amount) throws InsufficentBalanceException, NegativeAmountException
{
//Check if amount is greater than balance
if (amount > balance)
//throw an exception
throw new InsufficentBalanceException();
//Check if amount is less than 0
else if (amount < 0)
//Throw an exception
throw new NegativeAmountException();
//Else
else
//Calculate the balance
balance -= amount;
//Return the balance amount
return balance;
}
//Main method
public static void main(String[] args)
{
//Create an object for the class
Account a = new Account(10);
//Try block
try
{
//Deposit the value
a...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
- 1.class Nothing {public static void main(String args[]){int x = 0;int y = 20;int fraction = y/x;System.out.println("End Of Main");}}1. If there will occur exception, write the code that will handle the exception.arrow_forwardThe following class maintains an account balance and returns aspecial error code.public class Account{private double balance;// returns new balance or -1 if errorpublic double deposit(double amount){if (amount > 0)balance += amount;elsereturn -1; // Code indicating errorreturn balance;}}Rewrite the class so that it throws appropriate exception instead ofreturning -1 as an error code. Write test code that attempts to depositinvalid amounts and catches the exceptions that are thrownarrow_forwardC# Languagearrow_forward
- Note: It`s python codingarrow_forwardC# Programming What is the wrong of this code? Can you fix the error on this part? See attached photo for referencearrow_forwardQuestion 3 } public class WeekDay { static void daysFromSunday(int day) { String dayName = ""; try { } } if (day > 7) { throw new Exception("Invalid Day! Too high!"); } else if(day < 1){ throw new Exception("Invalid Day! Too low!"); } switch(day){ case 1: dayName="Sun"; break; case 2: dayName="Mon"; break; case 3: dayName="Tue"; break; case 4: dayName="Wed"; break; } ▬▬ } case 5: dayName="Thu"; Dreak; case 5: dayName="Thu"; break; case 6: dayName="Fri"; break; default: dayName="Sat"; System.out.println(dayName); } catch (Exception excpt) { System.out.println("Oops"); System.out.println(excpt.getMessage()); }finally{ System.out.println("Done!"); public static void main(String[] args) { daysFromSunday(10); daysFromSunday(3); daysFromSunday(0); OOops Invalid Day! Too high! Tue Oops Invalid Day! Too low! OOops Invalid Day! Too high! Done! Tue Done! Oops Invalid Day! Too low! Done! O Oops Invalid Day! Too high! Done! Oops Invalid Day! Too high! Done! Oops Invalid Day! Too low! Done! O Oops…arrow_forward
- PLZ help with the following: True/False In java it is possible to throw an exception, catch it, then re-throw that same exception if it is desired GUIs are windowing interfaces that handle user input and output. An interface can contain defined constants as well as method headings or instead of method headings. When a recursive call is encountered, computation is temporarily suspended; all of the information needed to continue the computation is saved and the recursive call is evaluated. Can you have a static method in a nonstatic inner class?arrow_forwardbasic java please Write a void method named emptyBox()that accepts two parameters: the first parameter should be the height of a box to be drawn, and the second parameter will be the width. The method should then draw an empty box of the correct size. For example, the call emptyBox(8, 5) should produce the following output: ***** * * * * * * * * * * * * ***** The width of the box is 5. The middle lines have 3 spaces in the middle. The height of the box is 8. The middle columns have 6 spacesarrow_forwardNote: It`s python codingarrow_forward
- Instructions The files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly. An example of the program is shown below: Enter name Juan Hello, Juan! Task 1: The DebugThree3 class compiles without error. Task 2: The getName() method accepts user input for a name and returns the name value as a String. Task 3: The displayGreeting() method displays a personalized greeting. Task 4: The DebugThree3 program accepts user input and displays the correct output.arrow_forwardComplete the code below according to the instructions below and the example test: method withdraw throws an exception if amount is greater than balance. For example: Test Result Account account = new Account("Acct-001","Juan dela Cruz", 5000.0); account.withdraw(5500.0); System.out.println("Balance: "+account.getBalance()); Insufficient: Insufficient funds. Balance: 5000.0 Account account = new Account("Acct-001","Juan dela Cruz", 5000.0); account.withdraw(500.0); System.out.println("Balance: "+account.getBalance()); Balance: 4500.0 Incomplete java code: public class Account{ private String accntNumber; private String accntName; private double balance; public Account(){} public Account(String num, String name, double bal){ accntNumber = num; accntName = name; balance = bal; } public double getBalance(){ return balance;}}//your class herearrow_forwardCode in C# (OOP Concept) Do a program that does the following: Ask for a number from the user in the main method o Pass thenumber to a method call it "Multiplication”. In the Method Multiplication, multiply the number by a number enteredby the user (mention that the number should be positive). Send the result back to the main and print it from the mainModify the previous program if the user enters a negative second number in the method Multiplication, do not acceptthe number and ask the user to reenter it again. . Add another method call it also Multiplication Ask for a float numberfrom the main and send it to the new Multiplication. This method should also ask for another positive number fromthe user and multiply it by the float number. Design and implement a function called "multiply" in C++ that accepttwo numbers and returns either the arithmetic multiplication or repetition of a given string a specific number of timesdepending on the data types of the argument values. The…arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
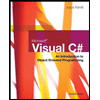
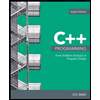
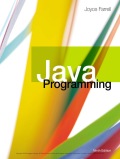
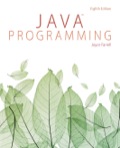
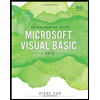