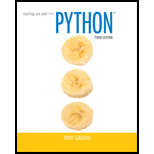
You can use the __________ operator to determine whether a key exists in a dictionary.
a. &
b. in
c. ^
d. ?

The “in” operator is used to check that key exists in the dictionary.
Hence, the correct answer is option “B”.
Explanation of Solution
Operator “in”:
- In Python dictionary, an “in” operator can be used to check if a key exists in the dictionary.
- The “in” operator returns true if the key exists in the dictionary.
- Otherwise, the “in” operator returns false.
Syntax:
In Python, the “in” operator can be expressed as follows:
key in dictionary_name
In the above syntax,
- The “dictionary_name” refers to the dictionary.
- The “key” represents the key to be searched in the dictionary.
Example program:
# Create a dictionary Student
Student={'Joanne':'CS-1234','Chris':'CS-1235','Jacob':'CS-1236'}
# Check if Joanne exists in the dictionary
if 'Joanne'in Student:
# Print the value corresponding to key 'Chris'
print("Value corresponding to key Joanne: " +Student['Joanne'])
Explanation:
In the above Python code, the first line creates a new dictionary “Student” with key-value pair elements “Joanne:CS-1234”, “Chris:1235”, and “Jacob:CS-1236”. The second line checks if the key “Joanne” exists in the dictionary “Student”. The third line prints the value corresponding to “Joanne” if the key “Joanne” exists in the dictionary “Student”.
Explanation for incorrect options:
Operator “&”:
In Python, the “&” operator is used for bitwise “And”.
Hence, option “A” is wrong.
Operator “^”:
In Python, the “^” operator is used for bitwise “Exclusive Or”.
Hence, option “C” is wrong.
Operator “?”:
The “?” is not a built- in operator in Python.
Hence, option “D” is wrong.
Output:
Value corresponding to key Joanne: CS-1234
Want to see more full solutions like this?
Chapter 9 Solutions
Starting Out with Python (3rd Edition)
Additional Engineering Textbook Solutions
Database Concepts (7th Edition)
Java How To Program (Early Objects)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Modern Database Management (12th Edition)
Software Engineering (10th Edition)
Starting Out with Java: Early Objects (6th Edition)
- Please write in python language.arrow_forwardExercise: empty_dictionary Description In this exercise, your function will receive no parameters. It will create an empty dictionary and return it. Function Name empty_dictionary Parameters None Return Value An empty dictionary. Examples empty_dictionary() > 0)arrow_forwardPython Programming Assignment: Create a dictionary that takes a basketball team's information (that includes team's name and points) as parameter, creates a teams_dict and returns it. Also, create a parameter for write_file parameter that opens current results text file in write mode and writes the dictionary information to file as per format and closes the file.arrow_forward
- Perform the following tasks: Create a dictionary named color_dict containing key-value pairs 'red': 222, 'green': 184, and 'blue': 135. Read strings key_read and value_read from input. Add key_read and value_read as a new key-value pair to color_dict with key_read as the key and value_read as the value.arrow_forwardPythonarrow_forwardPython code please help, indentation would be greatly appreciatedarrow_forward
- Assume the following list exists: names = ['Chris', 'Katie', 'Joanne', 'Kurt'] Write a statement that uses a dictionary comprehension to create a dictionary named X in which each element contains the length of a name from the list as the key, and the corresponding name's initial character as its value.arrow_forwardThis section has been set as optional by your instructor. OBJECTIVE: Write a program in Python that prints the middle value of a dictionary's values, using test_dict from the test_data.py module. NOTE: test_dict is created & imported from the module test_data.py into main.py Example 1: test dict = {'a':1,'b':9,'c':2,'d':5} prints 5 Example 2: test_dict = {'b':9,'d':5} prints 5 Hint: You may(or may not) find the following function useful: list.sort() which sorts the elements in a list. Note: the result is automatically stored in the original list, and return value from the function is None Туре list = [1, 2,3,2,1] After calling: list = [1,1,2,2,3] list.sort(), 339336.2266020 x3zgy7 LAB 13.11.1: Middlest Value (Structured Types) АCTIVITY 0/11 File is marked as read only Current file: test_data.py 1 #This module creates the test_dict to be used in main.py 2 #Reads input values from the user & places them into test_dict 3 dict_size = int(input()) #Stores the desired size of test_dict. 4…arrow_forwardCreate a static dictionary with a number of users and with the following values: First name Last name Email address Password Ask the user for: 5. Email address 6. Password Loop (for()) through the dictionary and if (if()) the user is found print the following: 7. Hello, first name last name you have successfully logged in 8. Notify the user if the password and email address are wrong 9. Additional challenge: if you want the program to keep asking for a username and password when the combination is wrong, you will need a while() loop. 10. Save the file as assignment03yourlastname.pyarrow_forward
- Overview This program will have you use a loop to prompt a user for three foods and their country of origin and then store them in a dictionary called food_dict). Then, it will prompt the user for a dish that they like and then will tell them the country of origin. Expected Output Example 1 What is good to eat? Tacos What country is that from? Mexico What is good to eat? Pho What country is that from? Vietnam What is good to eat? Burgers What country is that from? America What dish do you like? Burgers Burgers is from America Example 2 What is good to eat? Pizza What country is that from? Italy What is good to eat? Spaghetti What country is that from? Italy What is good to eat? Gelato What country is that from? Italy What dish do you like? Pizza Pizza is from Italy Specifications • You should submit a single file called M4A2.py • It should follow the submission standards outlined here: Submission Standards • Your program should start by defining an empty dictionary called food_dict •…arrow_forwardThis code is a part of a C dictionary. Please write the code for the below requirements. dict_get Next, you will implement: char* dict_get (const dict_t* dict, const char* key); This function goes through the list given by dict. If you use the above structure, this means starting at el = dict->head and checking each time whether the key at el is key; if it is not, we set el = el->next, until either key is found, or we reach el == NULL. To compare key with el->key, we need to compare one by one each character in key with those in el->key. Remember that strings in C are just pointers (memory addresses) to the first character in the string, so comparing el->key == key will not do what you want. So how do you even get the length of a string s? You would start at memory location s and advance as long as the byte at s (i.e. *s) is not the end-of-string marker (\0, the NULL character). This can get a bit messy, so luckily, you are allowed to use the string comparison…arrow_forwardCode the following directions in python: 1.Write a statement that creates a dictionary containing the following key-value pairs: 'a' : 1 'b' : 2 'c' : 3 2.Write a statement that creates an empty dictionary. 3.Assume the variable dct references a dictionary. Write an if statement that determines whether the key 'James' exists in the dictionary. If so, display the value that is associated with that key. If the key is not in the dictionary, display a message indicating so.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
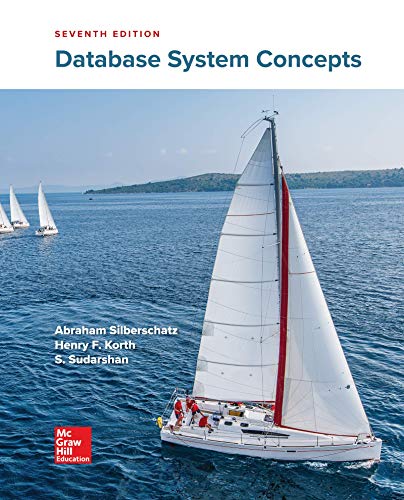
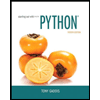
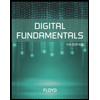
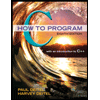
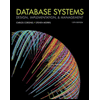
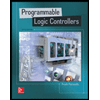