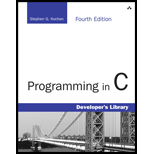
Programming in C
4th Edition
ISBN: 9780321776419
Author: Stephen G. Kochan
Publisher: Addison-Wesley
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 9, Problem 12E
Write a function called s that converts a character string into a floating point value. Have the function accept an optional leading minus sign. So, the call
s
should return the value – 8.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
MGMT SS STATS, an umbrella body that facilitates and serves various Social SecurityOrganizations/Departments within the Caribbean territories, stoodpoised to meet the needs of its stakeholders by launching an onlinedatabase at www.SSDCI.gov. The database will provide membersand the public access to the complete set of services that can (also)be initiated face-to-face, and it will provide managed, private, secure access to a repository ofpublic and/or personal information. Ideally, the database will have basic details of pensionplans recorded in the registry, member plan statistics, and cash inflows and outflows frompension funds.For example, insured persons accumulate contributions. Records for these persons will includeinformation on the insured persons able to acquire various benefits once work is interrupteddue to sickness, death, retirement, and maternity or employment injury. They will also includeinformation on pensions such as invalidity, disability, and survivors that stem from…
(c) Consider the following set of processes:
Process ID
Arrival Time Priority
Burst Time
A
2
3
100
B
6
C
10
1 (highest)
2
40
80
D
16
4 (lowest)
20
(3c) In the following resource allocation graph, is the state a deadlocked one? If so
which ones are deadlocked? (3 points)
Resource allocation graph. R₁ = Resource, P = process.
R1
R3
R3
7
Chapter 9 Solutions
Programming in C
Ch. 9 - Type in and run the 11 programs presented in this...Ch. 9 - Prob. 2ECh. 9 - Write a function called s to extract a portion of...Ch. 9 - Write a function called f to determine if one...Ch. 9 - Write a function called r to remove a specified...Ch. 9 - Write a function called i to insert one character...Ch. 9 - Using the f , r , and i functions from preceding...Ch. 9 - Extend the s function from Program 9.11 so that if...Ch. 9 - Write a function called s that converts a...Ch. 9 - If c is a lowercase character, the expression C...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Find the magnitude of the projected component of the force along the pipe AO.
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Identify and correct the errors in each of the following code segments assume that all variables have been prop...
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Determine the resultant internal normal force, shear force, and bending moment at point C in the beam.
Mechanics of Materials (10th Edition)
Can you use the method add to insert an element at a position for which you cannot use set?
Java: An Introduction to Problem Solving and Programming (8th Edition)
Explain the term cursor.
Database Concepts (8th Edition)
True or False: The superclass constructor always executes before the subclass constructor.
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- What resources are used when a thread is created? How do these differ from those used when a process is created?arrow_forward(c) Consider the following set of processes: Process ID Arrival Time Priority Burst Time A 2 3 100 B 6 C 10 1 (highest) 2 40 80 D 16 4 (lowest) 20arrow_forward(3e) Test-and-Set. The Test-and-Set instruction is used in hardware to achieve synchronization. It can be defined in the following way: function Test-and-Set (var target: boolean): boolean; begin Test-and-Set:= target; target := true; end; Now show how the Test-and-Set instruction can be used to protect a critical region and hence achieve mutual exclusion (do not worry about satisfying the bounded waiting condition). (8 points) repeatarrow_forward
- Consider a system with three smoker processes and one agent process. Each smoker continuously rolls a cigarette and then smokes it. But to roll and smoke a cigarette, the smoker needs three ingredients: tobacco, paper and matches. One of the smoker processes has paper, another has tobacco and the third has the matches. The agent has an infinite supply of all three materials. The agent places two of the ingredients on the table. The smoker who has the remaining ingredient then makes and smokes a cigarette, signaling the agent on completion. The agent then puts out another two of the three ingredients, and the cycle repeats. Given below is a solution to the Cigarette-Smokers Problem. Give initial conditions for the semaphores as well as plausible values for the variables i & j and r & s, such that the agent and smokers are synchronized. Write a couple of sentences on why these initial conditions are necessary and sufficient. Solution: var a: array [0..2] of semaphore (initial condition =…arrow_forwardLevel-0 Diagram for this: A customer sends in an order form containing details of their order and their membership number. A check is made to verify that they are a member. When their order is verified, a check is made to validate that the items ordered are produced by the company. Next, the valid order is used to update the daily order file, and then used to create a shipping list and invoice, which are sent on to the Order Fulfilment System.arrow_forwardIn this assignment, you will use all of the graphics commands you have learned to create an animated scene. Your program should have a clear theme and tell a story. You may pick any school-appropriate theme that you like. The program must include a minimum of: 5 circles 5 polygons 5 line commands 2 for loops 1 global variable You may wish to use the standard code for simplegui graphics below: import simplegui def draw_handler(canvas): frame = simplegui.create_frame('Testing', 600, 600) frame.set_canvas_background("Black") frame.set_draw_handler(draw_handler) frame.start() Submit Your Code After you write your code here in the programming environment, you will check it and submit it as usual. However, the grader will only perform basic checks against some requirements. If your code passes, you should submit your work, and your teacher will manually grade your submitted work using a rubric.arrow_forward
- 1. What is the difference between a relative cell reference and an absolute cell reference and give an example of when you would use each.arrow_forwardWhat is the goal of using a chart in excel, and how is a chart useful and what is the goal of using sparklines in excel, and how are sparklines useful?arrow_forwardProve for each pair of expression f(n) and g(n) whether f(n) is big O, little o Ω,ω or Θ of g(n). Use limits to find these. For each case it is possible that more than one of these conditions is satisfied:1. f(n) =log(n2^n), g(n) = log(sqrt(n)2^(n^2))2. f(n) =nsqrt(n) +log(n^n), g(n) =n + sqrt(n)lognarrow_forward
- Need this expression solved for mu. This can be done using a symbolic toolbox, however it needs to end up being mu = function (theta, m, L, g). If using MATLAB or something similar, run the code to make sure it works.arrow_forwardA business case scenario and asked to formulate an appropriate software design solution. Theyshould complete the case and upload the solution. will be required to read the case,identify and document the key issues, problems, and opportunities presented, and then design,and develop an appropriate integrated design solution to the problem. mustdemonstrate good spreadsheet, database, analytical, and word-processing skills whendeveloping solutions. Additionally, must be creative and demonstrate synthesising andapplying Database Management and Data Analytics Principles learned in the course. They willalso need to research some aspects of the assessment. CASE BACKGROUNDMGMT SS STATS, an umbrella body that facilitates and serves various Social SecurityOrganizations/Departments within the Caribbean territories, stoodpoised to meet the needs of its stakeholders by launching an onlinedatabase at www.SSDCI.gov. The database will provide membersand the public access to the complete set of…arrow_forwardA business case scenario and asked to formulate an appropriate software design solution. Theyshould complete the case and upload the solution. will be required to read the case,identify and document the key issues, problems, and opportunities presented, and then design,and develop an appropriate integrated design solution to the problem. mustdemonstrate good spreadsheet, database, analytical, and word-processing skills whendeveloping solutions. Additionally, must be creative and demonstrate synthesising andapplying Database Management and Data Analytics Principles learned in the course. They willalso need to research some aspects of the assessment. CASE BACKGROUNDMGMT SS STATS, an umbrella body that facilitates and serves various Social SecurityOrganizations/Departments within the Caribbean territories, stoodpoised to meet the needs of its stakeholders by launching an onlinedatabase at www.SSDCI.gov. The database will provide membersand the public access to the complete set of…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,COMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE L
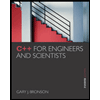
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
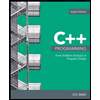
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
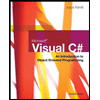
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
Computer Programming for Beginners | Functions, Parameters & Arguments | Ep24; Author: Programming With Avelx;https://www.youtube.com/watch?v=VXlh-qJpfw0;License: Standard YouTube License, CC-BY