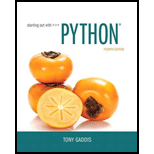
Starting Out with Python (4th Edition)
4th Edition
ISBN: 9780134444321
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 9, Problem 12AW
Write code that retrieves and unpickles the dictionary that you pickled in
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
Refer to page 80 for problems on white-box testing.
Instructions:
•
Perform control flow testing for the given program, drawing the control flow graph (CFG).
• Design test cases to achieve statement, branch, and path coverage.
• Justify the adequacy of your test cases using the CFG.
Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qo Hazb9tC440 AZF/view?usp=sharing]
Refer to page 10 for problems on parsing.
Instructions:
•
Design a top-down parser for the given grammar (e.g., recursive descent or LL(1)).
• Compute the FIRST and FOLLOW sets and construct the parsing table if applicable.
• Parse a sample input string and explain the derivation step-by-step.
Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]
Refer to page 20 for problems related to finite automata.
Instructions:
•
Design a deterministic finite automaton (DFA) or nondeterministic finite automaton (NFA) for the
given language.
• Minimize the DFA and show all steps, including state merging.
•
Verify that the automaton accepts the correct language by testing with sample strings.
Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]
Chapter 9 Solutions
Starting Out with Python (4th Edition)
Ch. 9.1 - An element in a dictionary has two parts. What are...Ch. 9.1 - Which part of a dictionary element must be...Ch. 9.1 - Suppose ' start' : 1472 is an element in a...Ch. 9.1 - Suppose a dictionary named employee has been...Ch. 9.1 - What will the following code display? stuff = {1 :...Ch. 9.1 - Prob. 6CPCh. 9.1 - Suppose a dictionary named inventory exists. What...Ch. 9.1 - What will the following code display? stuff = {1 :...Ch. 9.1 - What will the following code display? stuff = {1 :...Ch. 9.1 - What is the difference between the dictionary...
Ch. 9.1 - Prob. 11CPCh. 9.1 - Prob. 12CPCh. 9.1 - What does the values method return?Ch. 9.2 - Prob. 14CPCh. 9.2 - Prob. 15CPCh. 9.2 - Prob. 16CPCh. 9.2 - After the following statement executes, what...Ch. 9.2 - After the following statement executes, what...Ch. 9.2 - After the following statement executes, what...Ch. 9.2 - After the following statement executes, what...Ch. 9.2 - After the following statement executes, what...Ch. 9.2 - Prob. 22CPCh. 9.2 - After the following statement executes, what...Ch. 9.2 - After the following statement executes, what...Ch. 9.2 - Prob. 25CPCh. 9.2 - Prob. 26CPCh. 9.2 - After the following code executes, what elements...Ch. 9.2 - Prob. 28CPCh. 9.2 - After the following code executes, what elements...Ch. 9.2 - After the following code executes, what elements...Ch. 9.2 - After the following code executes, what elements...Ch. 9.2 - Prob. 32CPCh. 9.3 - Prob. 33CPCh. 9.3 - When you open a file for the purpose of saving a...Ch. 9.3 - When you open a file for the purpose of retrieving...Ch. 9.3 - Prob. 36CPCh. 9.3 - Prob. 37CPCh. 9.3 - Prob. 38CPCh. 9 - You can use the __________ operator to determine...Ch. 9 - You use ________ to delete an element from a...Ch. 9 - The ________ function returns the number of...Ch. 9 - You can use_________ to create an empty...Ch. 9 - The _______ method returns a randomly selected...Ch. 9 - The __________ method returns the value associated...Ch. 9 - The __________ dictionary method returns the value...Ch. 9 - The ________ method returns all of a dictionary's...Ch. 9 - The following function returns the number of...Ch. 9 - Prob. 10MCCh. 9 - Prob. 11MCCh. 9 - This set method removes an element, but does not...Ch. 9 - Prob. 13MCCh. 9 - Prob. 14MCCh. 9 - This operator can be used to find the difference...Ch. 9 - Prob. 16MCCh. 9 - Prob. 17MCCh. 9 - The keys in a dictionary must be mutable objects.Ch. 9 - Dictionaries are not sequences.Ch. 9 - Prob. 3TFCh. 9 - Prob. 4TFCh. 9 - The dictionary method popitem does not raise an...Ch. 9 - The following statement creates an empty...Ch. 9 - Prob. 7TFCh. 9 - Prob. 8TFCh. 9 - Prob. 9TFCh. 9 - Prob. 10TFCh. 9 - What will the following code display? dct =...Ch. 9 - What will the following code display? dct =...Ch. 9 - What will the following code display? dct =...Ch. 9 - What will the following code display? stuff =...Ch. 9 - How do you delete an element from a dictionary?Ch. 9 - How do you determine the number of elements that...Ch. 9 - Prob. 7SACh. 9 - What values will the following code display? (Dont...Ch. 9 - After the following statement executes, what...Ch. 9 - After the following statement executes, what...Ch. 9 - After the following statement executes, what...Ch. 9 - After the following statement executes, what...Ch. 9 - After the following statement executes, what...Ch. 9 - What will the following code display? myset =...Ch. 9 - After the following code executes, what elements...Ch. 9 - Prob. 16SACh. 9 - Prob. 17SACh. 9 - Prob. 18SACh. 9 - After the following code executes, what elements...Ch. 9 - Prob. 20SACh. 9 - Write a statement that creates a dictionary...Ch. 9 - Write a statement that creates an empty...Ch. 9 - Assume the variable dct references a dictionary....Ch. 9 - Assume the variable dct references a dictionary....Ch. 9 - Prob. 5AWCh. 9 - Prob. 6AWCh. 9 - Prob. 7AWCh. 9 - Prob. 8AWCh. 9 - Prob. 9AWCh. 9 - Prob. 10AWCh. 9 - Assume the variable dct references a dictionary....Ch. 9 - Write code that retrieves and unpickles the...Ch. 9 - Course information Write a program that creates a...Ch. 9 - Capital Quiz The Capital Quiz Problem Write a...Ch. 9 - File Encryption and Decryption Write a program...Ch. 9 - Unique Words Write a program that opens a...Ch. 9 - Prob. 5PECh. 9 - File Analysis Write a program that reads the...Ch. 9 - World Series Winners In this chapters source code...Ch. 9 - Name and Email Addresses Write a program that...Ch. 9 - Blackjack Simulation Previously in this chapter...Ch. 9 - Word Index Write a program that reads the contents...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Refer to page 60 for solving the Knapsack problem using dynamic programming. Instructions: • Implement the dynamic programming approach for the 0/1 Knapsack problem. Clearly define the recurrence relation and show the construction of the DP table. Verify your solution by tracing the selected items for a given weight limit. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440AZF/view?usp=sharing]arrow_forwardRefer to page 70 for problems related to process synchronization. Instructions: • • Solve a synchronization problem using semaphores or monitors (e.g., Producer-Consumer, Readers-Writers). Write pseudocode for the solution and explain the critical section management. • Ensure the solution avoids deadlock and starvation. Test with an example scenario. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward15 points Save ARS Consider the following scenario in which host 10.0.0.1 is communicating with an external SMTP mail server at IP address 128.119.40.186. NAT translation table WAN side addr LAN side addr (c), 5051 (d), 3031 S: (e),5051 SMTP B D (f.(g) 10.0.0.4 server 138.76.29.7 128.119.40.186 (a) is the source IP address at A, and its value. S: (a),3031 D: (b), 25 10.0.0.1 A 10.0.0.2. 1. 138.76.29.7 10.0.0.3arrow_forward
- 6.3A-3. Multiple Access protocols (3). Consider the figure below, which shows the arrival of 6 messages for transmission at different multiple access wireless nodes at times t=0.1, 1.4, 1.8, 3.2, 3.3, 4.1. Each transmission requires exactly one time unit. 1 t=0.0 2 3 45 t=1.0 t-2.0 t-3.0 6 t=4.0 t-5.0 For the CSMA protocol (without collision detection), indicate which packets are successfully transmitted. You should assume that it takes .2 time units for a signal to propagate from one node to each of the other nodes. You can assume that if a packet experiences a collision or senses the channel busy, then that node will not attempt a retransmission of that packet until sometime after t=5. Hint: consider propagation times carefully here. (Note: You can find more examples of problems similar to this here B.] ☐ U ப 5 - 3 1 4 6 2arrow_forwardJust wanted to know, if you had a scene graph, how do you get multiple components from a specific scene node within a scene graph? Like if I wanted to get a component from wheel from the scene graph, does that require traversing still? Like if a physics component requires a transform component and these two component are part of the same scene node. How does the physics component knows how to get the scene object's transform it is attached to, this being in a scene graph?arrow_forwardHow to develop a C program that receives the message sent by the provided program and displays the name and email included in the message on the screen?Here is the code of the program that sends the message for reference: typedef struct { long tipo; struct { char nome[50]; char email[40]; } dados;} MsgStruct; int main() { int msg_id, status; msg_id = msgget(1000, 0600 | IPC_CREAT); exit_on_error(msg_id, "Creation/Connection"); MsgStruct msg; msg.tipo = 5; strcpy(msg.dados.nome, "Pedro Silva"); strcpy(msg.dados.email, "pedro@sapo.pt"); status = msgsnd(msg_id, &msg, sizeof(msg.dados), 0); exit_on_error(status, "Send"); printf("Message sent!\n");}arrow_forward
- 9. Let L₁=L(ab*aa), L₂=L(a*bba*). Find a regular expression for (L₁ UL2)*L2. 10. Show that the language is not regular. L= {a":n≥1} 11. Show a derivation tree for the string aabbbb with the grammar S→ABλ, A→aB, B→Sb. Give a verbal description of the language generated by this grammar.arrow_forward14. Show that the language L= {wna (w) < Nь (w) < Nc (w)} is not context free.arrow_forward7. What language is accepted by the following generalized transition graph? a+b a+b* a a+b+c a+b 8. Construct a right-linear grammar for the language L ((aaab*ab)*).arrow_forward
- 5. Find an nfa with three states that accepts the language L = {a^ : n≥1} U {b³a* : m≥0, k≥0}. 6. Find a regular expression for L = {vwv: v, wЄ {a, b}*, |v|≤4}.arrow_forward15. The below figure (sequence of moves) shows several stages of the process for a simple initial configuration. 90 a a 90 b a 90 91 b b b b Represent the action of the Turing machine (a) move from one configuration to another, and also (b) represent in the form of arbitrary number of moves.arrow_forward12. Eliminate useless productions from Sa aA BC, AaBλ, B→ Aa, C CCD, D→ ddd Cd. Also, eliminate all unit-productions from the grammar. 13. Construct an npda that accepts the language L = {a"b":n≥0,n‡m}.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
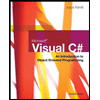
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Dictionaries - Introduction to Data Structures (Episode 8); Author: NullPointer Exception;https://www.youtube.com/watch?v=j0cPnbtp1_w;License: Standard YouTube License, CC-BY