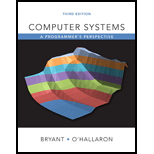
A.
Given code:
//Include necessary header files
#include <stdio.h>
#include "csapp.h"
//Define a main function
int main()
{
//Variable declaration
int status;
pid_t pid;
//Print the statement
printf("Hello\n");
//Call the method fork() and assign it to the variable pid
pid = fork();
//Print the statement
printf("%d\n",!pid);
//Check, pid is not equal to zero
if (pid != 0)
{
//True, check the condition
if (waitpid(-1, &status, 0) > 0)
{
//True, check the condition
if (WIFEXITED(status) != 0)
//True, print the statement
printf("%d\n" , WEXITSTATUS(status));
}
}
//Otherwise, print the statement
printf("Bye\n");
//Exit
exit(2);
}
Explanation:
The given code is used to print the number of output lines and possible outputs.
In the given code, the variable “status” and “pid_t pid” are declared.
- The main program first print the output line “Hello”.
- Then, call the function “fork()”, which is called once and return twice.
- Print the value that is not equal to the variable “pid”.
- The “if” condition check, the variable “pid” is not equal to “0”.
- True, “if” condition check, “(waitpid(-1, &status, 0) > 0)”.
- True, “if” condition check, “(WIFEXITED(status) != 0)”.
- True, print the value of “WEXITSTATUS(status)”.
- True, “if” condition check, “(WIFEXITED(status) != 0)”.
- True, “if” condition check, “(waitpid(-1, &status, 0) > 0)”.
- Print the statement “Bye”.
- Exit from the program.
B.
Explanation of Solution
Possible ordering of the output lines:
Possible output sequence corresponding to the process graph is as follows:
Hello

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
- Problem 1 jt"¡1, The following program is run on a machine where memory reference instructions take 8 cycles, arithmetic instructions take 6 cycles, and branches take 3 cycles. It is known that the “sqrt" procedure executes 7 instructions and requires 30 clock cycles. $s0, 20 ($s2) $t1, $s3, $s3 lw add j L1 $t1, $t1, $t1 $t1, $t1, $6 $a0, 0 ($t1) sqrt addi $s3, $s3, 1 beq $s0, $v0, Exit add L1: add lw jal (a). How long will it take to run this program if the machine has a 500 MHz clock? (b). What is the CPI for this program?arrow_forward2. Full code and plotting neweful. Task(5): Run the following code and note down the output. Also use real, imag and abs functions to plot real part, imaginary part and magnitude.>> n = [0:5]; x = exp ((2+3j)*n) Task(7): Write a program in Matlab to generate 1.5 s of a 50 Hz Saw tooth Waveform withsample rate fs = 10000 and plot 0.2 s of the generated waveform Task(1): Write a MATLAB code to sum unit step and unit ramp function . subject is signal and system please solve me all three.arrow_forward(a) Assume that five generation units with third order cost function (F, (R) = A; P+ B;P+C; P; + D;) are in the circuit. Write a computer program using any abitrary programming longuage (MATLAB, C++, C#, Python,.) to calculate economic load dipatch (ELD) using first order gradient method. Note that all parameters and variables should be defined inside the program (at tirst lines) such that units' characteristics and demand can be changed easily. Neglect grid losses. jusing dynamic programming (DP) methed.arrow_forward
- The programming language: C++ The union of two ordered lists (Sequential linear list)[the solution introduction: The first video of in 3.1, 35:00-43:00] [Problem description] Give the union of two ordered lists. The maximal number of elements in an inputted set is 30. [Basic requirements] 1) Use sequential linear list. 2) The result list should also be ordered. [Example] Problem: Give the union of the ordered lists (3,4,9,100,103) and (7,9,43,53,102,105). What you need to show in the terminal(the back part is outputted by you and the blue part is inputted by the user, i.e., teacher): Please input the first ordered list: (3,4,9,100,103)Please input the second ordered list: (7,9,43,53,102,105) The union is: (3,4,7,9,9,43,53,100,102,103,105)arrow_forward1. (Shortest Path) Please find the shortest path between the blue triangle and red tri- angle as Figure 1. Please explain your approach and develop the computationally efficient algorithm to get the results. Can you obtain (or estimate) the time com- plexity and memory complexity of your algorithm? 麗arrow_forwardPractice Problems (5) 1) Write a program to make a table of the physical properties for water in the range of temperatures from 273 to 323 K. The Density : The conductivity: K= 0.34 + 9.278 * 10* T The Specific heat: Cp= 0.015539 (T – 308.2)² + 4180.9 p= 1200.92 – 1.0056 T + 0.001084 Tarrow_forward
- Problem 7: Explain the outcome of each of the following code segment?(a)addi $t0, $zero, 0xFF2Bandi $t2, $t2, $t0 (b)ori $t2, $t2, 0x00E9 I NEED ANSWER ASAP. PLEASE DO FASTarrow_forwardPROBLEM 21 - 0517: Write a subroutine which computes the roots of the quadratic equation a,x2 + a,x + a, = 0 according to the quadratic formula: X12 = (-az/2a,) + V[(a,/2a,)2 – (a,/a,)) (= [{a, + v(a?, - 4a,a,)} / 2a,]) (START SUBROUTINE QUAD COMPUTE, DISCRIMINANT (DISC) DISCarrow_forwardQ1) Consider a simple traffic light system to regulate safe pedestrian crossing on a busy lane. Consider the following system requirement:(SysReq:)The traffic lights shall allow pedestrians to safely cross the lane by stopping cars together with the following software requirements: (SofReq1:)The light switch for pedestrians will be set to 'green' within x seconds after the pedestrian button has been pressed. (SofReq2:)The light switch for cars will be set to 'red' at least y seconds before the light switch for pedestrians is set to 'green'. Find missing environment assumptions and domain properties that are necessary to build the following satisfaction argument: {SofReq1, SofReq2, assumptions?, domain properties?} =SysReq Are the missing domain properties adequate? Are the missing assumptions realistic?arrow_forward1.Full code and plotting neweful. Task(5): Run the following code and note down the output. Also use real, imag and abs functions to plot real part, imaginary part and magnitude.>> n = [0:5]; x = exp ((2+3j)*n) Task(7): Write a program in Matlab to generate 1.5 s of a 50 Hz Saw tooth Waveform withsample rate fs = 10000 and plot 0.2 s of the generated waveform Task(1): Write a MATLAB code to sum unit step and unit ramp function . subject is signal and system please solve me all three.arrow_forwardI need help with problem 17arrow_forward3. The diagram below shows the main land routes for vehicular traffic between points A and G in a city. The figures in the arcs represent the cost of traveling between each pair of nodes. a) Manually apply Dijkstra's algorithm to find the cheapest route between A and G (visited nodes and total distance). b) Formulate a linear programming problem in extended form, to determine the shortest route to travel from A to G. Do not use subscripts, name 14 variables, for example XFE would be the variable that indicates that the arc from F to E is used. c) If there is a fixed cost for visiting each node, modify the formulation of the problem to include said fixed cost in the objective function, and the variables and restrictions that are required. NODE A B C D E F G FIXED COST 25 18 32 20 28 18 34arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
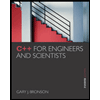