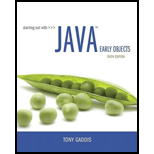
Array:
An array is defined as a group that consists of similar data types.
- The array size must be specified by an “int” value and not long or short.
- In Java, all arrays are allocated dynamically.
- An array is always indexed, starting from 0.
Declaring an array reference and creating an array:
- To insert elements to an array, first an array has to be created by using new operator and then the reference variable is assigned.
- The syntax is as follows,
arrayRefVar = new elementType[arraySize]
- From the above statement it is clear that, an array is created using new elementType[arraySize] and then the reference to the newly created array is assigned to the variable arrayRefVar.
- It is possible to declare an array variable, create an array and assign the reference to a variable in a single statement itself.
The syntax will be as follows,
elementType[]arrayRefVar = new elementType[arraySize];
elementType arrayRefVar[]= new elementType[arraySize);
Example:
double myFile = new double[20];
The statement given above declares an array variable myFile and then creates an array that consists of 20 elements which are of the datatype double and at last assign the reference to myFile.
Explanation of Solution
b. Loop to display each element in the array:
//Class definition
public class Sample {
// define main function
public static void main(String[] args) {
// Declaration of string array
String[] planets = {"Mercury", "Venus", "Earth", "Mars"};
// Loop to read each element in the planets array
for(int i = 0; i<planets.length; i++)
// Display the contents of the planet array
System...
Explanation of Solution
c. Loop to display first character of the elements present in the array:
//Class definition
public class Sample {
// define main function
public static void main(String[] args) {
// Declaration of string array
String[] planets = {"Mercury", "Venus", "Earth", "Mars"};
// Loop to read each element in the planets array
for(int i = 0; i<planets.length; i++)
// Display the contents of the planet array
System.out.println(planets[i]...

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Starting Out with Java: Early Objects (6th Edition)
- Please show the code for the Tikz figure of the complex plane and the curve C. Also, mark all singularities of the integrand.arrow_forward11. Go to the Webinars worksheet. DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows: a. Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell. b. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells. c. Determine and enter the constraints based on the information provided in Table 3. d. Use Simplex LP as the solving method to find a global optimal solution. e. Save the Solver model below the Maximum weekly profit model label. f. Solve the model, keeping the Solver solution. Table 3: Solver Constraints Constraint Cell or Range Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5 Each Tuesday and…arrow_forwardGo to the Webinars DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows: Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells. Determine and enter the constraints based on the information provided in Table 3. Use Simplex LP as the solving method to find a global optimal solution. Save the Solver model below the Maximum weekly profit model label. Solve the model, keeping the Solver solution. Table 3: Solver Constraints Constraint Cell or Range Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5 Each Tuesday and Thursday…arrow_forward
- I want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)? When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene. Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency. To elaborate,…arrow_forwardI want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)?When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene.Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency.To elaborate, what…arrow_forwardMale comedians were typically the main/dominant star of television sitcoms made during the FCC licensing freeze. Question 19 options: True False In the episode of The Honeymooners that you watched this week, why did Alice decide to get a job outside of the home? Question 1 options: to earn enough money to buy a mink coat to have something to do while the kids were at school to pay the bills after her husband got laid offarrow_forward
- After the FCC licensing freeze was lifted, sitcoms featuring urban settings and working class characters became far less common. Question 14 options: True Falsearrow_forwardsolve this questions for me .arrow_forwarda) first player is the minimizing player. What move should be chosen?b) What nodes would not need to be examined using the alpha-beta pruning procedure?arrow_forward
- Consider the problem of finding a path in the grid shown below from the position S to theposition G. The agent can move on the grid horizontally and vertically, one square at atime (each step has a cost of one). No step may be made into a forbidden crossed area. Inthe case of ties, break it using up, left, right, and down.(a) Draw the search tree in a greedy search. Manhattan distance should be used as theheuristic function. That is, h(n) for any node n is the Manhattan distance from nto G. The Manhattan distance between two points is the distance in the x-directionplus the distance in the y-direction. It corresponds to the distance traveled along citystreets arranged in a grid. For example, the Manhattan distance between G and S is4. What is the path that is found by the greedy search?(b) Draw the search tree in an A∗search. Manhattan distance should be used as thearrow_forwardwhats for dinner? pleasearrow_forwardConsider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand. ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page) main()arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
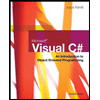
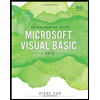
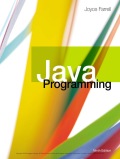
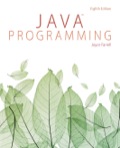
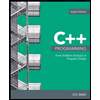