Concept explainers
Fat Gram Calculator
Design a
Validate the input as follows:
- Make sure the number of fat grams and calories is not less than 0.
- According to nutritional formulas, the number of calories cannot exceed fat grams X 9. Make sure that the number of calories entered is not greater than fat grams X 9.
Once correct data has been entered, the program should calculate and display the percentage of calories that come from fat. Use the following formula:
Some nutritionists classify a food as “low fat” if less than 30 percent of its calories come from fat. If the results of this formula are less than 0.3, the program should display a message indicating the food is low in fat.

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
EBK STARTING OUT WITH PROGRAMMING LOGIC
Additional Engineering Textbook Solutions
Management Information Systems: Managing The Digital Firm (16th Edition)
SURVEY OF OPERATING SYSTEMS
Starting Out with Python (4th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Degarmo's Materials And Processes In Manufacturing
- (Mathematical functions) Write a program that calculates and displays values for y when y=xz/(xz) Your program should calculate y for values of x ranging between 1 and 5 and values of z ranging between 2 and 6. The x variable should control the outer loop and be incremented in steps of 1, and z should be incremented in steps of 1. Your program should also display the message Function Undefined when the x and z values are equal.arrow_forwardOdd Even Number Problem Description Even Numbers are integers that are exactly divisible by 2, whereas an odd number cannot be exactly divided by 2. Example of even numbers are 2,4,6,8 and odd numbers are 1, 3, 5, 7, 9. Input The first line input contains an integer, which determines the number of test cases. Each of following lines represent sequence of integers that ends with 0. 2 34 1 8 5 22 0 10 7 16 -2 0 Output For each test case, the output will present the size of oddQueue and evenQueue following with the integers of odd and even numbers. oddQueue 2: 1 5 evenQueue 3: 34 8 22 oddQueue 1: 7 evenQueue 3: 10 16 -2 ** Your task is to write a program that will read the input and identify whether it is an odd number or even number. /******************************************************************************* Compilation: javac Queue.java** The Queue class represents a first-in-first-out (FIFO) queue of generic items.* It supports the usual enqueue and dequeue…arrow_forwardA car’s miles-per-gallon (MPG) can be calculated with the following formula:MPG 5 Miles driven Gallons of gas usedWrite a program that asks the user for the number of miles driven and the gallons of gas used. It should calculate the car’s MPG and display the result.arrow_forward
- An online book club awards points to its customers based on the number of books purchased each month. Points are awarded as follows: Books Purchased Points Earned 1 5 2 15 3 30 4 or more 60 Write a program that asks the user to enter the number of books purchased this month and then displays the number of points awarded.arrow_forwardPraticing Write a flowgorithm program that will calculate a person’s mobile phone bill based on plan chosen and the data used. The program should perform the following: Ask for customer’s mobile plan Ask for the number of gigabytes of data used Display an error message if the plan choice is invalid or the gigabytes used is less than zero (0) Mobile data plans are: Plan A 19.99/month, with 4 gigabytes data, additional data $10/gigabyte Plan B 29.99/month, with 8 gigabytes data, additional data $5/gigabyte Plan C 39.99/month, unlimited data Remember the following: use clear prompts for your input label each output number or name (Comment: I need help practicing with Flowgorithm, I don't understand the concept of flowgorithm, and I need the practice to help me understand computer science better. Please help me, thank you).arrow_forwardHide Assignment Information Instructions The civil engineering department has asked you to write a program to compare three different designs of water towers shown in the diagrams below. The water towers are a cylinder topped with a half sphere. The engineers want to find the design that has the highest volume. The radius of the sphere and the radius and height of the cylinder for each of the three projects will need to be input by the user. The formula for the volume of a Sphere is:V = 4/3 π r3The formula for the volume of a Cylinder is:V = π r2 h Write two functions that will calculate the volume of a sphere and a cylinder respectively. Then, use these functions to write a program that will find the design with the largest volume. Hint: Remember that the tower only consists of a half sphere.arrow_forward
- - Calculate Income Tax Problem Statement A program is required to determine to calculate the amount of Virginia State Income tax a person will pay. The user will enter their annual income. The Virginia income tax rate is 5.75%. Display the amount of tax they need to pay.arrow_forwardPython code easy way pleasearrow_forwardSales Prediction The East Coast sales division of a company generates 58 percent of total sales. Based on that percentage, write a program that will predict how much the East Coast division will generate if the company has $8.6 million in sales this year. Display your prediction on the screen. Miles per Gallon A car holds 15 gallons of gasoline and can travel 375 miles before refueling. Write a program that calculates the number of miles per gallon (MPG) the car gets. Display the result on the screen. Stock Commission Kathryn brought 750 shares of stock at a price of $35.00 per share. She must pay her stockbroker a 2 percent commission for the transaction. Write a program that calculates and displays the following: The amount paid for the stock alone (without the commission). The amount of the commission. The total amount paid (for the stock plus the commission). Annual Pay Suppose an employee gets paid every two weeks and earns $2,200 each pay period. In a year, the employee gets…arrow_forward
- Instructions Instructions Write a program that prompts the user to enter two integers. The program outputs how many numbers are multiples of 3 and how many numbers are multiples of 5 between the two integers (inclusive).arrow_forwardInterest Rates Savings accounts state an interest rate and a compounding period. If theamount deposited is P, the stated interest rate is r, and interest is compounded m timesper year, then the balance in the account after one year is P⋅(1+rm)m. For instance, if$1000 is deposited at 3% interest compounded quarterly (that is, 4 times per year), thenthe balance after one year is1000⋅(1+.034)4=1000⋅1.00754=$1,030.34.Interest rates with different compounding periods cannot be compared directly.The concept of APY (annual percentage yield) must be used to make the comparison. TheAPY for a stated interest rate r compounded m times per year is defined byAPY=(1+rm)m−1.(The APY is the simple interest rate that yields the same amount of interest after oneyear as the compounded annual rate of interest.) Write a program to compare interestrates offered by two different banks and determine the most favorable interest rate. SeeFig. 4.24.arrow_forwardSummary During each summer, John and Jessica grow vegetables in their backyard and buy seeds and fertilizer from a local nursery. The nursery carries different types of vegetable fertilizers in various bag sizes. When buying a particular fertilizer, they want to know the price of the fertilizer per pound and the cost of fertilizing per square foot. Instructions The following program prompts the user to enter the size of the fertilizer bag, in pounds, the cost of the bag, and the area, in square feet, that can be covered by the bag. The program should output the desired result. However, the program contains logic errors. Find and correct the logic errors so that the program works properly. //Logic errors.#include <iostream>#include <iomanip> using namespace std; int main(){ double cost; double area; double bagSize; cout << fixed << showpoint << setprecision(2); cout << "Enter the amount of fertilizer, in pounds, " <<…arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
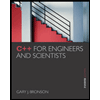
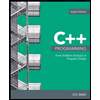