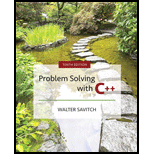
A common memory matching game played by young children is to start with a deck of cards that contains identical pairs. For example, given six cards in the deck, two might be labeled “1,” two might be labeled “2,” and two might be labeled “3.” The cards are shuffled and placed face down on the table. The player then selects two cards that are face down, turns them face up, and if they match they are left face up. If the two cards do not match, they are returned to their original position face down. The game continues in this fashion until all cards are face up. Write a program that plays the memory matching game. Use 16 cards that are laid out in a 4 × 4 square and are labeled with pairs of numbers from 1 to 8. Your program should allow the player to specify the cards that she would like to select through a coordinate system. For example, suppose the cards are in the following layout:
All of the cards are face down except for the pair 8, which has been located at coordinates (1, 1) and (2, 3). To hide the cards that have been temporarily placed face up, output a large number of newlines to force the old board off the screen.
(Hint: Use a two-dimensional array for the arrangement of cards and another two-dimensional array that indicates if a card is face up or face down. Write a function that “shuffles” the cards in the array by repeatedly selecting two cards at random and swapping them. Random number generation is described in Chapter 4.)

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Concepts of Programming Languages (11th Edition)
C Programming Language
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Modern Database Management
C++ How to Program (10th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
- Use java languagearrow_forwardKingdom of Trolls is celebrating their Kingdom Day and one of the activities that is taking place is a game where a player rolls a magic ball down the hill on a path with spikes. As the ball rolls down, it strikes a spike and bursts open to release a number of smaller balls (in our simulated game, the number of smaller balls is a randomly generated integer between 2 and 6, inclusive). As the smaller balls further roll down, when one strikes a spike, that ball and all its sibling balls burst and each generates another set of smaller balls (using the same random number already generated for the first roll). The balls keep rolling downhill and striking spikes and bursting into smaller balls until a golden ball is released by one of the bursts. At this time, the game is over and the player is told how many balls were generated during the last burst (including the golden ball). The game is played by two players at a time and the player who had the lowest number of balls generated on the…arrow_forwardPython tic tac toe. Tic tac toe is a very popular game. Only two players can play at a time. Game Rules Traditionally the first player plays with "X". So you can decide who wants to go with "X" and who wants to go with "O". Only one player can play at a time. If any of the players have filled a square then the other player and the same player cannot override that square. There are only two conditions that may match will be a draw or may win. The player that succeeds in placing three respective marks (X or O) in a horizontal, vertical, or diagonal row wins the game. Winning condition Whoever places three respective marks (X or O) horizontally, vertically, or diagonally will be the winner. Submit your code and screenshots of your code in action. Hints : Have a function that draws the board Have a function that checks position if empty or not Have a function that checks player or won or not expected output:arrow_forward
- Python tic tac toe. Tic tac toe is a very popular game. Only two players can play at a time. Game Rules Traditionally the first player plays with "X". So you can decide who wants to go with "X" and who wants to go with "O". Only one player can play at a time. If any of the players have filled a square then the other player and the same player cannot override that square. There are only two conditions that may match will be a draw or may win. The player that succeeds in placing three respective marks (X or O) in a horizontal, vertical, or diagonal row wins the game. Winning condition Whoever places three respective marks (X or O) horizontally, vertically, or diagonally will be the winner. Submit your code and screenshots of your code in action. Hints : Have a function that draws the board Have a function that checks position if empty or not Have a function that checks player or won or not expected output:arrow_forwardJava Program Scenario: A high school has 1000 students and 1000 lockers, one locker for each student. On the first day of school, the principal plays the following game: She asks the first student to open all the lockers. She then asks the second student to close all the even-numbered lockers. The third student is asked to check every third locker. If it is open, the student closes it; if it is closed, the student opens it. The fourth student is asked to check every fourth locker. If it is open, the student closes it; if it is closed, the student opens it. The remaining students continue this game. In general, the nth student checks every nth locker. If the locker is open, the student closes it; if it is closed, the student opens it. After all the students have taken their turns, some of the lockers are open and some are closed. Write a program that prompts the user to enter the number of lockers in a school. After the game is over, the program outputs the number of lockers and the…arrow_forwardCCC '13 J1 - Next in line Canadian Computing Competition: 2013 Stage 1, Junior #1 You know a family with three children. Their ages form an arithmetic sequence: the difference in ages between the middle child and youngest child is the same as the difference in ages between the oldest child and the middle child. For example, their ages could be 5, 10 and 15, since both adjacent pairs have a difference of 5 years. Given the ages of the youngest and middle children, what is the age of the oldest child? Input Specification The input consists of two integers, each on a separate line. The first line is the age Y of the youngest child (0arrow_forwardA popular word game involves finding words from a grid of randomly generatedletters. Words must be at least three letters long and formed from adjoining letters.Letters may not be reused and it is valid to move across diagonals. As an example,consider the following 4 * 4 grid of letters: A B C DE F G HI J K LM N O P The word “FAB” is valid (letters in the upper left corner) and the word “KNIFE”is valid. The word “BABE” is not valid because the “B” may not be reused. Theword “MINE” is not valid because the “E” is not adjacent to the “N”. Write a program that uses a 4 * 4 two-dimensional array to represent the gameboard. The program should randomly select letters for the board. You may wishto select vowels with a higher probability than consonants. You may also wish toalways place a “U” next to a “Q” or to treat “QU” as a single letter. The programshould read the words from the text file words.txt (included on the website withthis book) and then use a recursive algorithm to…arrow_forwardMoving Magic Square is the name of a game that is based on the concept of a magic square. A magic square is any square array of numbers, usually positive integers, in which the sums of the numbers in each row, each column, and both main diagonals are the same. For example, the 3 x 3 square in Table 1 is a magic square because the sum of every row, every column and the two diagonals is 15. Table 1 6 18 753 294 The game, Moving Magic Square, is played on any n x n grid containing positive integer numbers from 1, ..., n². The number n² is the movable number. You can move the number n² in one of four directions (up/down/left/right), and swap n² with the number that is currently occupying that cell. The player wants to move the number n² to reach a goal state such that the sum of the n numbers in every row, column, and both diagonals is equal to k. There are multiple states that satisfy this condition, and you can stop the game when you find the first goal state. In a 3 x 3 game, 9 is the…arrow_forwardThe classic Eight Queens puzzle is to place eight queens on a chessboard such that no two queens can attack each other (i.e., no two queens are in the same row, same column, or same diagonal). There are many possible solutions. Write a program that displays one such solution.arrow_forwardTiling: The precondition to the problem is that you are given threeintegers n, i, j, where i and j are in the range 1 to 2n. You have a 2n by 2n squareboard of squares. You have a sufficient number of tiles each with the shape . Your goalis to place nonoverlapping tiles on the board to cover each of the 2n × 2n tiles except forthe single square at location i, j. Give a recursive algorithm for this problem in whichyou place one tile yourself and then have four friends help you. What is your base case?arrow_forwardCan you help me with this code because i am struggling and I don't know what to do with this part: he Eight Puzzle consists of a 3 x 3 board of sliding tiles with a single empty space. For each configuration, the only possible moves are to swap the empty tile with one of its neighboring tiles. The goal state for the puzzle consists of tiles 1-3 in the top row, tiles 4-6 in the middle row, and tiles 7 and 8 in the bottom row, with the empty space in the lower-right corner. In this section, you will develop two solvers for a generalized version of the Eight Puzzle, in which the board can have any number of rows and columns. We have suggested an approach similar to the one used to create a Lights Out solver in Homework 2, and indeed, you may find that this pattern can be abstracted to cover a wide range of puzzles. If you wish to use the provided GUI for testing, described in more detail at the end of the section, then your implementation must adhere to the recommended interface. However,…arrow_forwardBeeblebrox question help requiredarrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
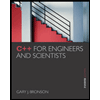
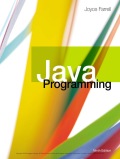