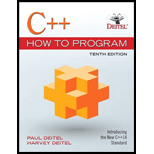
C++ How to Program (10th Edition)
10th Edition
ISBN: 9780134448237
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 6, Problem 6.38E
Program Plan Intro
Tower of Hanoi Using Recursion
- Include required header files.
- Create a function “tower_of_hanoi()”.
- The description of parameter variables are like this -int diskfor number of disks, char srcpegfor source peg, char destpegfor destination peg and char temppegas auxiliary peg.
- If number of disk is 1 then display srcpegtodestpeg.
- Else make a recursive call totower_of_hanoi().
- Display srcpegtodestpeg.
- Make another recursive call totower_of_hanoi().
- Define the “main()” function
- Create an integer variabledisk and assign it.
- Make a call to tower_of_hanoi()with number of disks, source peg, auxiliary peg and destination peg.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Answer the following mention question with opps and JS
Write the simplest C++ program that will demonstrate iteration vs recursion using the following guidelines -
Please include pre/post and pseudocode for the 3 functions which are not 'main' in the solution.
Write two primary helper functions - one iterative (IsArrayPrimeIter) and one recursive (IsArrayPrimeRecur) - each of which
Take an array and its size as input params and return a bool such that
'true' ==> array and all elements are prime,
'false' ==> at least one element in array is not prime, so array is not prime.
Print out a message "Entering <function_name>" as the first statement of each function.
Perform the code to test whether every element of the array is a Prime number.
Print out a message "Leaving <function_name>" as the last statement before returning from the function.
Remember - there will be nested loops for the iterative function and there can be no loops at all in the recursive function.
For the recursive function - define one other helper…
I need the answer quickly
Chapter 6 Solutions
C++ How to Program (10th Edition)
Ch. 6 - Show the value of x after each of the following...Ch. 6 - (Parking Charges) A parking garage charges a...Ch. 6 - Prob. 6.13ECh. 6 - (Rounding Numbers) Function floor can be used to...Ch. 6 - Prob. 6.15ECh. 6 - (Random Numbers) Write statement that assign...Ch. 6 - (Random Numbers) Write a single statement that...Ch. 6 - Prob. 6.18ECh. 6 - Prob. 6.19ECh. 6 - Prob. 6.20E
Ch. 6 - Prob. 6.21ECh. 6 - Prob. 6.22ECh. 6 - Prob. 6.23ECh. 6 - (Separating Digits) Write program segments that...Ch. 6 - (Calculating Number of Seconds) Write a function...Ch. 6 - (Celsius and Fahrenheit Temperature) Implement the...Ch. 6 - (Find the Minimum) Write a program that inputs...Ch. 6 - Prob. 6.28ECh. 6 - (Prime Numbers) An integer is said to be prime if...Ch. 6 - Prob. 6.30ECh. 6 - Prob. 6.31ECh. 6 - (Quality Points for Numeric Grades) Write a...Ch. 6 - Prob. 6.33ECh. 6 - (Guess-the-Number Game) Write a program that plays...Ch. 6 - (Guess-the-Number Game Modification) Modify the...Ch. 6 - Prob. 6.36ECh. 6 - Prob. 6.37ECh. 6 - Prob. 6.38ECh. 6 - Prob. 6.39ECh. 6 - Prob. 6.40ECh. 6 - Prob. 6.41ECh. 6 - Prob. 6.42ECh. 6 - Prob. 6.43ECh. 6 - Prob. 6.44ECh. 6 - (Math Library Functions) Write a program that...Ch. 6 - (Find the Error) Find the error in each of the...Ch. 6 - (Craps Game Modification) Modify the craps program...Ch. 6 - (Circle Area) Write a C++ program that prompts the...Ch. 6 - (pass-by-Value vs. Pass-by-Reference) Write a...Ch. 6 - (Unary Scope Resolution Operator) What’s the...Ch. 6 - (Function Templateminimum) Write a program that...Ch. 6 - Prob. 6.52ECh. 6 - (Find the Error) Determine whether the following...Ch. 6 - (C++ Random Numbers: Modified Craps Game) Modify...Ch. 6 - (C++ Scoped enum) Create a scoped enum named...Ch. 6 - (Function Prototype and Definitions) Explain the...Ch. 6 - Prob. 6.57MADCh. 6 - Prob. 6.58MADCh. 6 - (Computer-Assisted Instruction: Monitoring Student...Ch. 6 - (Computer-Assisted Instruction: Difficulty Levels)...Ch. 6 - (Computer-Assisted Instruction: Varying the Types...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In your own words, explain the two rules that a proper recursive definitionor function must followarrow_forwardAnswer the given question with a proper explanation and step-by-step solution. Write the provided sequence as a recursive function. 1,2,4,8,16,32,dotsarrow_forwardFor each of the following applications, mention the data structure that will be most suitable to use. Justify your answer. a) For finding the shortest path between source and destination location. b) For implementing a recursive function, where each call to function must return to the immediate last call. c) For checking balanced parenthesis in an arithmetic expression. Please don't copy the answer anywhere. Please.arrow_forward
- 8. Ackerman's Function Ackermann's Function is a recursive mathematical algorithm that can be used to test how well a system optimizes its performance of recursion. Design a function ackermann(m, n), which solves Ackermann's function. Use the following logic in your function: If m = 0 then return n + 1 If n = 0 then return ackermann(m-1,1) Otherwise, return ackermann(m-1,ackermann(m,n-1)) Once you've designed yyour function, test it by calling it with small values for m and n. Use Python.arrow_forwardPlease help. I don’t understandarrow_forward4. Write a recursive function code that finds the elements of the given sequence: a, 3a-1 + n², and a 1. What is the time complexity of your code? Explain it.arrow_forward
- Define recursive function. Explain tracing of recursive function with suitable example.arrow_forwardUsing Python Recursion is the concept of a function calling itself until the problem is solved when the Base Case is met. Study Recursion: See slides in Modules, Practice Slides, lab12 and also Recursion is in chapter 9 of the textbook. Some examples of recursion are: compute [ factorial of a number, towers of Hanoi, fractals (as shown in the textbook), and many more]. Example: See slides Page 2 For this assignment we'll use Collatz Cojecture (see Wikipedia). Collatz Conjecture algorithm: Given a number n, first call to the function: f(n): In the function: if n == 1 return 1: if n is even then f(n/2), i.e. call self with the new value. else (n is odd) call self with the new value, f((n*3)+1) and repeat. All numbers eventually end up with 1. The program should test for the base case which is: if n == 1, in which case it returns to the caller with 1. This problem is perfect to demonstrate Recursion.Create a list with random numbers (you can just do this part manually) in the list as 1,…arrow_forwardA recursive function must have two parts: its basis and its recursive part. Explain what each of these is and why it is essential to recursion.arrow_forward
- Why is it required to provide a base case for every recursive function?arrow_forwardprogram in C++ with the name, towers.cpp which helps to solve the Towers of Hanoi puzzle using this recursive approach. The main() routine should make a single call to the recursive member function do Towers(). This function then calls itself recursively until the puzzle is solved. Note: solve as soon as possiblearrow_forwardChoose the correc answer in the question below.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
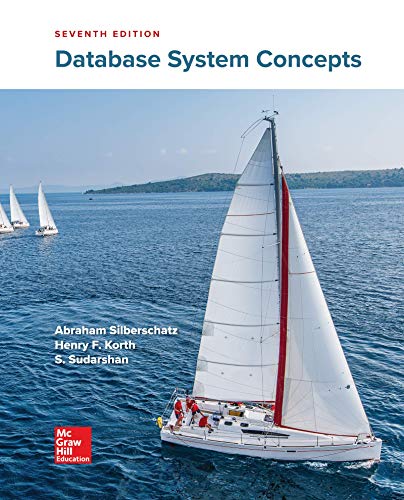
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
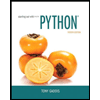
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
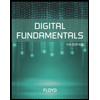
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
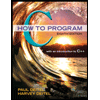
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
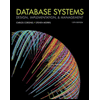
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
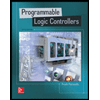
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education