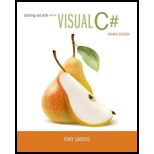
Starting out with Visual C# (4th Edition)
4th Edition
ISBN: 9780134382609
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 5, Problem 9PP
Program Plan Intro
Dice simulator
Program plan:
Design the form:
- Place a two picture box controls on the form, and change its name and properties to display the dices.
- Place a one command button control on the form, and change its name and properties to simulates the rolling a pair of dices.
In code window, write the code:
Program.cs:
- Include the required libraries.
- Define the namespace “Dice”.
- Define a class “Program”.
- Define a constructor for the class.
- Define required functions to run “Form1”.
- Define a class “Program”.
Form1.cs:
- Include the required libraries.
- Define namespace “Dice”.
- Define a class “Form1”.
- In Form1_Load() method,
- Create an object for Random class to generate two random numbers in the range of “1” through “6” for Dice1 and Dice2.
- Use switch case to display the respective dice image in picture Box1.
- Use switch case to display the respective dice image in picture Box2.
- Close the application.
Form Design:
View the Form Design in IDE.
Set the Form Control properties in Properties window as follows:
Object | Property | Setting |
Form1 | Text | Program5_9 |
pictureBox1 | InitialImage | Select Resource |
pictureBox1 | InitialImage | Select Resource |
btnDice | Text | Roll Dices |
- Add two picture box controls to the form from Toolbox.
- Add one command button control to the form from Toolbox.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Kinetic Energy In physics, an object that is in motion is said to have kinetic energy. The following formula can be used to determine a moving object’s kinetic energy: KE =1/2mv2 In the formula KE is the kinetic energy, m is the object’s mass in kilograms, and v is the object’s velocity in meters per second. Create an application that allows the user to enter an object’s mass and velocity and then displays the object’s kinetic energy. The application should have a method named KineticEnergy that accepts an object’s mass (in kilograms) and velocity (in meters per second) as arguments. The method should return the amount of kinetic energy that the object has.
Shape1. Shape =5 will make the shape
control appears as a
rectangle O
square O
oval O
rounded square O
Falling Distance When an object is falling because of gravity, the following formula can be used to determine the distance the object falls in a specific time period: d =1/2gt2 The variables in the formula are as follows: d is the distance in meters, g is 9.8, and t is the amount of time in seconds that the object has been falling. Create an application that allows the user to enter the amount of time that an object has fallen and then displays the distance that the object fell. The application should have a method named FallingDistance. The FallingDistance method should accept an object’s falling time (in seconds) as an argument. The method should return the distance in meters that the object has fallen during that time interval.
Chapter 5 Solutions
Starting out with Visual C# (4th Edition)
Ch. 5.1 - Prob. 5.1CPCh. 5.1 - Prob. 5.2CPCh. 5.1 - Prob. 5.3CPCh. 5.2 - What is a loop iteration?Ch. 5.2 - What is a counter variable?Ch. 5.2 - What is a pretest loop?Ch. 5.2 - Does the while loop rest its condition before or...Ch. 5.2 - What is an infinite loop?Ch. 5.3 - What messages will the following code sample...Ch. 5.3 - How many rimes will the following loop iterate?...
Ch. 5.4 - Name the three expressions that appear inside the...Ch. 5.4 - You want to write a for loop that displays I love...Ch. 5.4 - What would the following code display? for (int...Ch. 5.4 - What would the following code display? for (int...Ch. 5.5 - What is a posttest loop?Ch. 5.5 - What is the difference between the while loop and...Ch. 5.5 - How many times will the following loop iterate?...Ch. 5.6 - What is an output file?Ch. 5.6 - What is an input file?Ch. 5.6 - What three steps must be taken by a program when...Ch. 5.6 - What is the difference between a text file and a...Ch. 5.6 - Prob. 5.22CPCh. 5.6 - What type of object do you create if you want to...Ch. 5.6 - What type of object do you create if you want to...Ch. 5.6 - If you call the File.CreateText method and the...Ch. 5.6 - If you call the File.AppendText method and the...Ch. 5.6 - What is the difference between the WriteLine and...Ch. 5.6 - What method do you call to open a text file to...Ch. 5.6 - What is a files read position? Initially, where is...Ch. 5.6 - How do you read a line of text from a text file?Ch. 5.6 - How do you close a file?Ch. 5.6 - Assume inputFile references a StreamReader object...Ch. 5.7 - What is the benefit of using an Open and/or Save...Ch. 5.7 - Prob. 5.34CPCh. 5.8 - What does a Random objects Next method return?Ch. 5.8 - What does a Random objects NextDouble method...Ch. 5.8 - Write code that creates a Random object and then...Ch. 5.8 - Write code that creates a Random object and then...Ch. 5.8 - Prob. 5.39CPCh. 5.8 - What happens if the same seed value is used each...Ch. 5.9 - Prob. 5.41CPCh. 5.9 - Prob. 5.42CPCh. 5 - ListBox controls have an __________ method that...Ch. 5 - A __________ is commonly used to control the...Ch. 5 - A(n) __________ loop tests its condition before...Ch. 5 - The term __________ is used to describe a file...Ch. 5 - The term __________ file is used to describe a...Ch. 5 - A __________ file contains data that has been...Ch. 5 - When you work with a __________ file you access...Ch. 5 - Prob. 8MCCh. 5 - A __________ object is an object that is...Ch. 5 - When a program works with an input file, a special...Ch. 5 - When the user selects a file with the Open dialog...Ch. 5 - The __________ control displays a standard Windows...Ch. 5 - Once you have created a Random object, you can...Ch. 5 - Prob. 14MCCh. 5 - When you run an application, the applications form...Ch. 5 - If the ListBox is empty, the Items.Count property...Ch. 5 - To increment a variable means to increase its...Ch. 5 - When a variable is declared in the initialization...Ch. 5 - The while loop always performs at least one...Ch. 5 - The term read file is used to describe a file that...Ch. 5 - To append data to an existing file, you open it...Ch. 5 - As items are read from the file, the read position...Ch. 5 - The numbers that are generated by the Random class...Ch. 5 - Prob. 10TFCh. 5 - What is contained in the body of a loop?Ch. 5 - Write a programming statement that uses postfix...Ch. 5 - How many iterations will occur if the test...Ch. 5 - What are filename extensions? What do they...Ch. 5 - When an input file is opened, what is its read...Ch. 5 - How can you read all of the items in a file...Ch. 5 - What is a variable that is used to accumulate a...Ch. 5 - By default, the Open dialog box displays the...Ch. 5 - Prob. 9SACh. 5 - Prob. 10SACh. 5 - Write a loop that displays your name 10 times.Ch. 5 - Write a loop that displays all the odd numbers...Ch. 5 - Write a loop that displays every fifth number from...Ch. 5 - Write a code sample that uses a loop to write the...Ch. 5 - Assume that a file named People.txt contains a...Ch. 5 - Distance Calculator If you know a vehicles speed...Ch. 5 - Distance File Modify the Distance Calculator...Ch. 5 - Celsius to Fahrenheit Table Assuming that C is a...Ch. 5 - Prob. 4PPCh. 5 - Pennies for Pay Susan is hired for a job, and her...Ch. 5 - Prob. 6PPCh. 5 - Prob. 7PPCh. 5 - Prob. 8PPCh. 5 - Prob. 9PPCh. 5 - Addition Tutor Create an application that...Ch. 5 - Random Number Guessing Game Create an application...Ch. 5 - Calculating the Factorial of a Number In...Ch. 5 - Random Number File Writer Create an application...Ch. 5 - Random Number File Reader This exercise assumes...
Knowledge Booster
Similar questions
- Theater RevenueA movie theater only keeps a percentage of the revenue earned from ticket sales. The remainder goes to the movie company. Create a GUI application that allows the user to enter the following data into text fields:� Price per adult ticket� Number of adult tickets sold� Price per child ticket� Number of child tickets soldThe application should calculate and display the following data for one night�s box office business at a theater:� Gross revenue for adult tickets sold. This is the amount of money taken in for all adult tickets sold.� Net revenue for adult tickets sold. This is the amount of money from adult ticket sales left over after the payment to the movie company has been deducted.� Gross revenue for child tickets sold. This is the amount of money taken in for all child tickets sold.� Net revenue for child tickets sold. This is the amount of money from child ticket sales left over after the payment to the movie company has been deducted.� Total gross revenue. This…arrow_forwardGUI calculator in python - The user enters two integers into the text fields. - When the Add button is pressed, the sum of values in the text fields are shown after the Equals: as a label. - The Clear button clears the values in the text fields and the result of the previous calculation. The cleared values can be blank or zero. - The Quit button closes the GUI window.arrow_forward02 - Rectangular Prisms Prompt the user for three integers representing the sides of a rectangular prism. Calculate the surface area and volume of the prism, then draw three rectangles of asterisks portraying the top, side, and front view of the prism labeled accordingly (which set of numbers represent each view is at your discretion). Enter three integers: 2 5 6 Surface Area: 104 Volume 60 Top View (2 x 5): Side View (2 x 6): Front View (5 x 6):arrow_forward
- Tuition Increase At one college the tuition for a full-time student is $6000 per semester. It has been announced that the tuition will increase by 2 percent each year for the next five years. Create an application with a loop that displays the projected semester tuition amount for the next 5 years in a ListBox control.arrow_forwardFor each of the following exercises, you may choose to write a console-based or GUI application, or both. Write a program for The Carefree Resort named ResortPrices that prompts the user to enter the number of days for a resort stay. Then display the price per night and the total price. Nightly rates are $200 for one or two nights; $180 for three or four nights; $160 for five, six, or seven nights; and $145 for eight nights or more.arrow_forwardFor each of the following exercises, you may choose to write a console-based or GUI application, or both. Write a program named TestScoreList that accepts eight int values representing student test scores. Display each of the values along with a message that indicates how far it is from the average.arrow_forward
- Python tkinter GUI: Create a search box where the user types in a word If the user types in the word "cat" and clicks the button "enter" the following words appear in a drop down menu: animal friend companion Otherwise the output will be "Try Again!"arrow_forwardInstructions Objectives: Use a while loop Use multiple loop controlling conditions Use a boolean method Use the increment operator Extra credit: Reuse earlier code and call two methods from main Details:This assignment will be completed using the Eclipse IDE. Cut and paste your code from Eclipse into the Assignment text window. This is another password program. In this case, your code is simply going to ask for a username and password, and then check the input against four users. The program will give the user three tries to input the correct username-password combination. There will be four acceptable user-password combinations: alpha - alpha1 beta - beta1 gamma - gamma1 delta - delta1 If the user types in one of the correct username-password combinations, then the program will output: “Login successful.” Here are a couple of example runs (but your code needs to work for all four user-password combinations): Username: betaType your current password: beta1Login…arrow_forwardProperty Tax If you own real estate in a particular county, the property tax that you owe each year is calculated as 64 cents per $100 of the property’s value. For example, if the property’s value is $10,000, then the property tax is calculated as follows: Tax = $10,000 ÷ 100 × 0.64 Create an application that allows the user to enter a property’s value and displays the sales tax on that property.arrow_forward
- 2. Image ViewerWrite an application that allows the user to view image files. The application should useeither a button or a menu item that displays a file chooser. When the user selects an imagefile, it should be loaded and displayed.arrow_forwardJAVA Guess-the-Number Game Write an application that plays “guess the number” as follows: Your application chooses the number to be guessed by selecting an integer at random in the range 1–1000. The application then displays the following in a label: I have a number between 1 and 1000. Can you guess my number? Please enter your first guess. A JTextField should be used to input the guess. } As each guess is input, the background color should change to either red or blue. Red indicates that the user is getting “warmer,” and blue, “colder.” A JLabel should display either "Too High" or "Too Low" to help the user zero in. When the user gets the correct answer, "Correct!" should be displayed, and the JTextField used for input should be changed to be uneditable. A JButton should be provided to allow the user to play the game again. When the JButton is clicked, a new random number should be generated and the input JTextField changed to be editable.arrow_forwardTheater RevenueA movie theater only keeps a percentage of the revenue earned from ticket sales. The remainder goes to the movie company. Create a GUI application that allows the user to enterthe following data into text fields:• Price per adult ticket• Number of adult tickets sold• Price per child ticket• Number of child tickets soldThe application should calculate and display the following data for one night’s box officebusiness at a theater:• Gross revenue for adult tickets sold. This is the amount of money taken in for all adulttickets sold.• Net revenue for adult tickets sold. This is the amount of money from adult ticket salesleft over after the payment to the movie company has been deducted.• Gross revenue for child tickets sold. This is the amount of money taken in for all childtickets sold.• Net revenue for child tickets sold. This is the amount of money from child ticket salesleft over after the payment to the movie company has been deducted.• Total gross revenue. This is the…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
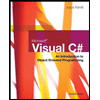
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
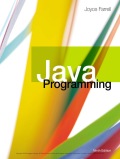
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
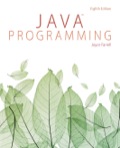
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT